One of the most important components of web application security and performance management is rate limitation. It promotes equitable use of APIs and services, safeguards resources, and helps stop abuse. We’ll look at how to add rate limitation to an ASP.NET Core application in this article. We’ll go over the idea, its advantages, and offer a detailed how-to with explanations for the code.
Understanding Rate Limiting
A method called rate limitation limits how many requests a client may make to a server in a certain amount of time. By preventing malicious or excessive resource utilization, this technique makes sure that a single user or IP address cannot overload the server and cause performance degradation.
Rate restriction in ASP.NET Core can be accomplished with a number of packages, but we’ll concentrate on the AspNetCoreRateLimit package because it makes the implementation process easier.
Benefits of Rate Limiting
- Security: By flooding a server with requests, brute force assaults, DDoS attacks, and other malicious actions can be avoided with the use of rate limitation.
- Fair Usage: By preventing any one client from controlling all of the server’s resources, it guarantees that every user has an equitable experience.
- Stability: Rate limitation helps to maintain the stability and dependability of your application by stopping excessive requests.
- Resource Conservation: Rate limitation helps to maintain the stability and dependability of your application by stopping excessive requests.
Implementation Steps
Let’s have a look at how to use the AspNetCoreRateLimit package to enable rate limitation in an ASP.NET Core application. We’ll begin at the beginning and go over each step in detail.
Step 1. Create a new ASP.NET Core Project
Using your preferred development environment, start by creating a new ASP.NET Core project. The following command can be entered into the terminal.
dotnet new web -n RateLimitExample
Step 2. Install the AspNetCoreRateLimit Package
Install the AspNetCoreRateLimit package in the terminal by navigating to the root directory of your project and running the following command.
dotnet add package AspNetCoreRateLimit
Step 3. Configure Rate Limiting in Startup.cs
Locate and open the ConfigureServices function in the Startup.cs file. To set up rate limitation with the IPRateLimitPolicy, add the following code.
using AspNetCoreRateLimit; public void ConfigureServices(IServiceCollection services) { // Other configurations... services.AddMemoryCache(); services.Configure<IpRateLimitOptions>(Configuration.GetSection("IpRateLimiting")); services.AddHttpContextAccessor(); services.AddSingleton<IRateLimitCounterStore, MemoryCacheRateLimitCounterStore>(); services.AddSingleton<IIpPolicyStore, MemoryCacheIpPolicyStore>(); services.AddSingleton<IRateLimitConfiguration, RateLimitConfiguration>(); // Other configurations... }
Step 4. Configure Rate Limiting Settings in appsettings.json
Add the following configuration line to your appsettings.json file so that the rate-limiting settings are specified.
"IpRateLimiting": { "EnableEndpointRateLimiting": true, "StackBlockedRequests": false, "RealIpHeader": "X-Real-IP", "ClientIdHeader": "X-ClientId", "HttpStatusCode": 429, "GeneralRules": [ { "Endpoint": "*", "Period": "1s", "Limit": 5 } ] }
Each IP address is restricted to 5 requests per second for all endpoints under this setup.
Step 5. Apply Rate Limiting to Endpoints
You must, for example, add the [RateLimit] attribute to your controller actions or methods in order to apply rate restriction to particular endpoints.
[ApiController] [Route("api/[controller]")] public class ValuesController : ControllerBase { [HttpGet] [RateLimit(Name = "CustomLimit", Seconds = 10, Requests = 2)] public ActionResult<IEnumerable<string>> Get() { // Your action logic... } }
The Get action in this example is restricted to two requests per ten seconds.
Step 6. Test the Rate Limiting
To test the rate restriction, run your ASP.NET Core application and use a tool like curl or Postman. You will see that you will receive a 429 Too Many Requests response if you exceed the specified rate restrictions.
Conclusion
It is imperative that you implement rate restriction in your ASP.NET Core application to ensure optimal performance, fair resource utilization, and security. The process is made simpler by the AspNetCoreRateLimit package, which offers middleware and properties that are simple to use. You can successfully install rate limiting and safeguard your application from abusive or excessive requests by following the instructions provided in this article.
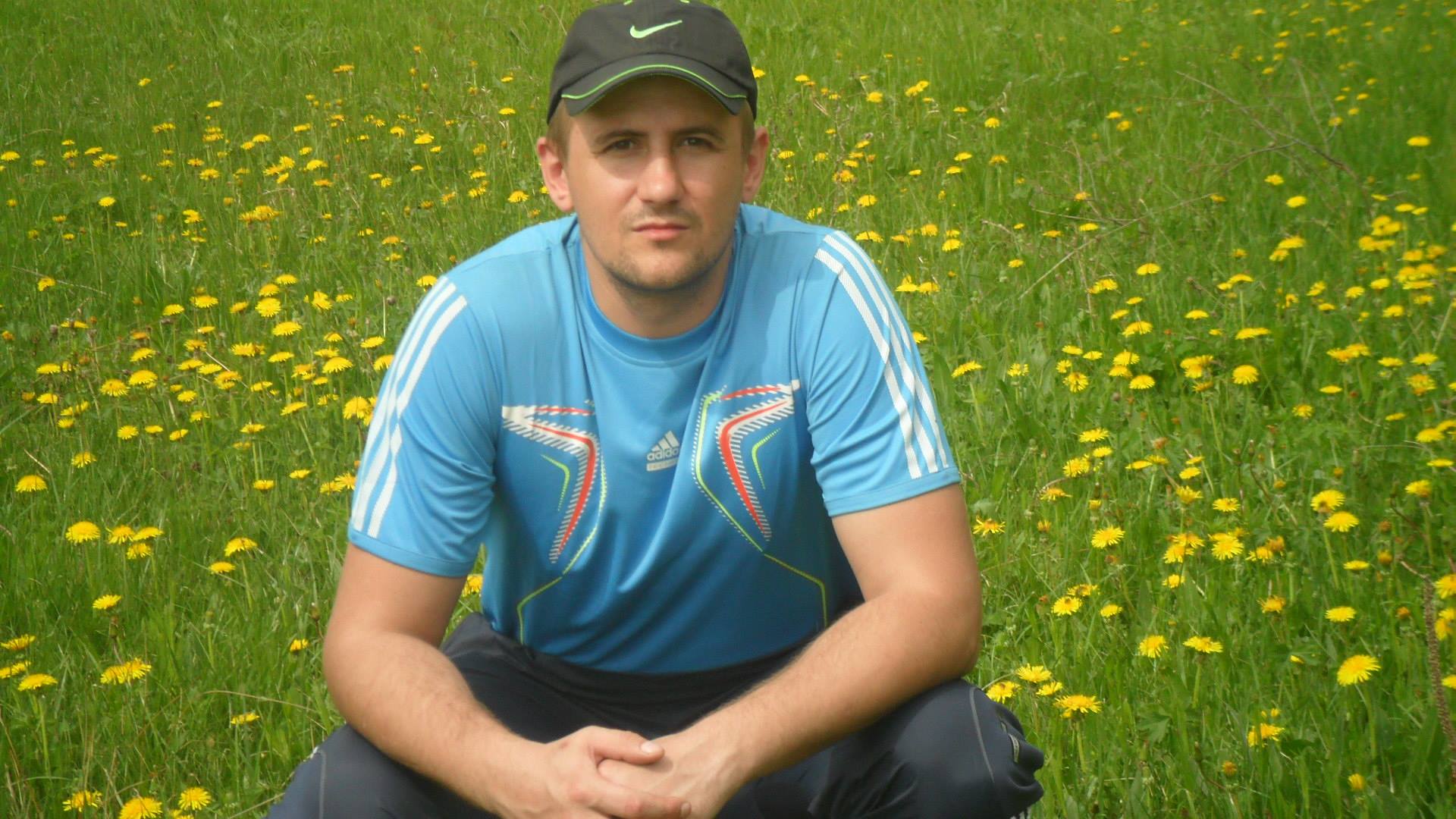
Yury Sobolev is Full Stack Software Developer by passion and profession working on Microsoft ASP.NET Core. Also he has hands-on experience on working with Angular, Backbone, React, ASP.NET Core Web API, Restful Web Services, WCF, SQL Server.