Occasionally, we must submit application data in the form of a document, such as a PDF or an image. In those situations, we had to translate the HTML layout into a document; in this case, we’ll perform the same process to translate HTML into PDF.
The following is quick solution to convert HTML to PDF.
First, please make sure you use below npm commands.
npm install jspdf
And use the provided npm command to install the html2canvas package.
npm install html2canvas
It’s time to use the import statement to import it into our component after the installation process is complete.
import * as jspdf from 'jspdf'; import html2canvas from 'html2canvas';
The UI design process is now underway. Copy the following snippet of code into app.component.html and open it.
</pre> <div id="content"> <div class="alert alert-info"><strong>Html To PDF Conversion - Angular 6</strong></div> <div></div> </div> <div> <table id="contentToConvert"> <tbody> <tr> <th>Column1</th> <th>Column2</th> <th>Column3</th> </tr> <tr> <td>Row 1</td> <td>Row 1</td> <td>Row 1</td> </tr> <tr> <td>Row 2</td> <td>Row 2</td> <td>Row 2</td> </tr> <tr> <td>Row 3</td> <td>Row 3</td> <td>Row 3</td> </tr> <tr> <td>Row 4</td> <td>Row 4</td> <td>Row 4</td> </tr> <tr> <td>Row 5</td> <td>Row 5</td> <td>Row 5</td> </tr> <tr> <td>Row 6</td> <td>Row 6</td> <td>Row 6</td> </tr> </tbody> </table> </div> <pre>
As you can see from the code above, I simplified this example by creating a straightforward HTML table. This is not the same as tags; instead, I used the Angular Material component to style my user interface. Now that we have finished the user interface, let’s run the application and view the results.
I created a PDF of an HTML table using a capture button. Upon clicking the Capture button, an A4-sized PDF will be generated. To write the method, open the app.component.ts file and follow the steps listed below.
import { Component, OnInit, ElementRef ,ViewChild} from '@angular/core'; import * as jspdf from 'jspdf'; import html2canvas from 'html2canvas'; @Component({ selector: 'app-htmltopdf', templateUrl: './htmltopdf.component.html', styleUrls: ['./htmltopdf.component.css'] }) export class HtmltopdfComponent{ public captureScreen() { var data = document.getElementById('contentToConvert'); html2canvas(data).then(canvas => { // Few necessary setting options var imgWidth = 208; var pageHeight = 295; var imgHeight = canvas.height * imgWidth / canvas.width; var heightLeft = imgHeight; const contentDataURL = canvas.toDataURL('image/png') let pdf = new jspdf('p', 'mm', 'a4'); // A4 size page of PDF var position = 0; pdf.addImage(contentDataURL, 'PNG', 0, position, imgWidth, imgHeight) pdf.save('MYPdf.pdf'); // Generated PDF }); } }
I have developed a method called captureScreen(), which generates a document, adds an image, and then saves it locally, as you can see from the code snippet above. I’ve included a few settings, like image height, width, and left margin, for that. When JsPDF generates a PDF, its layout is determined by its syntax.
new JsPDF( Orientation, // Landscape or Portrait unit, // mm, cm, in format // A2, A4 etc );
Using pdf.addImage(), which is used to use the image into our document, I will insert the image into my document after JsPDF has been initialized. Some comparable techniques are listed below.
- addHTML()
- addFont()
- addPage()
- addMetaData()
It’s now time to run our application and view the desired result by running ng serve -o.
Conclusion
This is how we can convert our HTML code, with all font settings, images, metadata, and other elements, into a PDF file. You can consult their official documentation for additional functionalities.
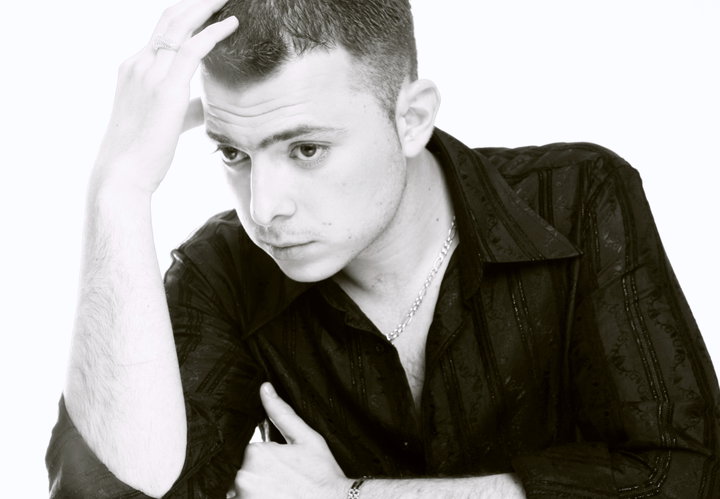
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.