Web APIs are essential whether you want to build a web application or incorporate other features. A potent web application development framework for building web APIs that set them apart from other web-based solutions is ASP.NET Core. It is one of the main ways that the.NET Core platform is implemented.
It is possible to create a variety of web APIs with ASP.NET Core that have multiple uses. It enables programmers to design safe and scalable APIs. Development of ASP.NET core has many benefits, such as cross-platform compatibility, high performance, and flexibility.
One of the main benefits of using ASP.NET Core for web API development is performance. In fact, ASP.NET Core can process more user requests in a second than Node.js and Java Servlet put together. This tutorial is intended for those who intend to create a web API using ASP.NET Core or who are searching for API integration services. It offers comprehensive details on Web APIs as well as instructions for building APIs with ASP.NET Core.
What is API?
An Application Programming Interface, or API, provides a way for data exchange and communication between two different systems. It establishes guidelines and conventions for the communication between various software systems. To put it simply, APIs are similar to connecting wires that transfer data between ends.
APIs are made up of different functions that facilitate the retrieval of specific features or data from them. SDKs and APIs are frequently confused. That being said, an SDK (Software Development Kit) is not the same as an API. An SDK is a set of tools for creating features and applications, whereas the application programming interface facilitates communication between two systems.
What is a Web API?
As the name suggests, a web API is an API for web-based interfaces. The HTTP protocol and other web-based technologies are used by web APIs. Thus, server-client architecture-based web applications are created using web APIs.
Types of Web APIs
If you want to use ASP.NET to create a Web API, you should first choose the kind of API you want to build. As stated below, there are various kinds of Web APIs according to their range of applications and accessibility.
Public API
An application programming interface that is publicly accessible and that any outside developer or company can use for software development is called a Public API, also referred to as an Open API or External API. These APIs can be freely integrated with software systems and are not limited to any particular user.
Developers can use a Public API to get particular features and data from third-party software that they can use in their applications. Numerous Open APIs, such as Websocket, GraphQL, Google Translate API, and JSONPlaceholder API, are readily accessible in the public domain.
Private API
This type of API is restricted and not accessible to the general public. Internal or private APIs are designed exclusively for internal use within an organization; they are never made available to external users. The purpose of these APIs is to link the systems and software in an organization. For instance, a company may have an API to link its payroll and human resources systems.
Because they are only used internally and are not available to the public, private APIs are extremely secure. The Netflix internal APIs, which let developers easily access metadata and other content, are an example of a private API.
Partner API
One type of API that is only available to particular users is a Partner API. Users who are authorized and trusted can access partner APIs. These APIs facilitate cooperation and business-to-business transactions. For instance, in order to function, CRM systems require access to customer data. An organization can use a Partner API to help it share this custom data with a third-party service on a selective basis.
Composite API
The purpose of composite APIs is to minimize the round-trip time for each call by combining several API requests into a single call. It speeds up and improves the effectiveness of your web application. You can process multiple requests in a single database transaction by using this API. When placing an order, an API can perform multiple requests in one call, such as validating payment information, checking inventory, and updating order history. This is the best example of a composite API in the eCommerce industry.
Architectures and Protocols of APIs
An architecture and protocol should be in place to facilitate the efficient exchange of data and commands between APIs. Different architectures and protocols specify guidelines, limitations, and ways to communicate commands and data. They dictate the overall behavior of an API.
RPC
The first type of API that allows for straightforward interactions is called RPC (Remote Procedure Call). RPC APIs send and receive data with multiple parameters. The RPC protocol functions similarly to calling functions in Python, JavaScript, PHP, and other programming languages with parameters. RPC can use either JSON or XML for coding.
SOAP
Another standard for developing Web APIs is SOAP (Simple Object Access Protocol). The WWW (World Wide Web) Consortium established this messaging standard. With the help of this protocol, web apps can exchange data in XML format. Applications that are sending and receiving messages exchange messages via application protocols such as TCP/IP, SMTP, HTTP, etc.
REST
The most widely used and current API protocol is REST. Representational State Transfer, or REST, uses a client/server architecture. Development becomes more effective and adaptable when the frontend and backend of APIs are separated.
It is a stateless API protocol, meaning that information is not stored in between requests. An API that complies with the REST constraints is called a RESTful API. REST is the architecture for APIs, and RESTful is the API built upon it, according to this comparison of REST and RESTful APIs.
Why Opt for Web APIs with ASP.NET Core?
For web development, there are numerous frameworks and technologies available. Building safe, scalable, and adaptable web apps is made possible by ASP.NET Core, which has a ton of features and many benefits. A lot of businesses are creating their enterprise apps with ASP.NET Core. For the following reasons, Web API development uses it extensively.
- It provides unified features and tools for creating Web UIs and APIs.
- Features for testing are already included.
- With Razor Pages, you can create a web page and its related data in a single file by using a page-focused approach.
- It is a cross-platform framework for Linux, macOS, and Windows application development and execution.
- This framework is open source.
- It has dependency injection built in and is ready for the cloud.
- A quick, lightweight, and modular HTTP request pipeline is provided by ASP.NET Core.
- Integrated support for a variety of data formats.
- It is compatible with ODATA (Open Data Protocol) and routing.
- For CRUD operations, it makes use of common web verbs like GET, PUT, POST, and DELETE.
How Can ASP.NET Core Be Used to Create Web APIs?
Are you curious about the variety of APIs available in ASP.NET? You can create SOAP, REST, and other kinds of APIs with ASP.NET as it supports a variety of API types.
There are two methods you can use to create web APIs for your application: controller-based APIs and minimal APIs. Controllers manage the endpoint logic in a controller-based API. The ControllerBase class has subclasses that are called controllers. Therefore, the controller serves as a conduit for communication between the client (a web application or browser) and the API.
In contrast, a Minimal API uses logical handlers to define its endpoints, such as functions and lambdas. To create your web API, you can contrast and select between a Controller-based API and a Minimal API. The instructions for creating a basic API with the ASP.NET Core framework are provided here. Let’s examine these actions.
Step 1: Prerequisites
- An IDE or code editor such as Visual Studio Code (VSC) or Visual Studio is required.
- You also need to install the web development workload and ASP.NET in the case of Visual Studio.
- Other than Visual Studio Code, the most recent versions of C# for VSC and.NET 7 are needed.
Step 2: Start with an API Project
- With Visual Studio, you can select ASP.NET Core Empty from templates to start an API project. In the ensuing window, give your project a name, select.NET 7.0, and deselect “Do not use top-level statements” in the “Additional information” window.
- When using Visual Studio Code, open the integrated terminal, navigate to the project’s directory, and type the following command:
dotnet new web -o “Your API name”
without quotes. Navigate to the project directory withcd “Your API name”
without quotes. Now use commandcode –r ../”Your API name”
without quotes. Choose “Yes” for dialog boxes asking to trust the authors and add required assets. - A web minimal API project is created after this step.
Step 3: Run the Application
- Press CTRL+F5 to launch the application in Visual Studio, then click Accept in the dialog box that displays the SSL certificate.
Step 4: Create Code
- You must put your logic and code in the
Program.cs
file, which is the main file.Program.cs
files should use the classes you create based on your requirements. - NuGet packages must also be added in order to support databases and diagnostics. Use the GET, PUT, POST, and DELETE endpoints in accordance with the specifications of your application. To send or store data in JSON format, set up JSON serialization.
Conclusion
ASP.NET Core Web API facilitates the development of HTTP services that are accessed by various clients, including web browsers and mobile devices. A feature-rich web framework for creating APIs and web apps is called ASP.NET Core. If you want to create web applications using the.NET platform, this is a good option. Moreover, you can create more adaptable web APIs by utilizing ASP.NET Core MVC to take advantage of the advantages of MVC patterns.
ASPHostPortal is one of the best alternatives for your ASP.NET hosting, offering:
- Better pricing and value for money
- Feature-rich hosting plans
- 99.9% uptime guarantee
- Enhanced security features
- Global data center locations
- Multilingual customer support
Whether you’re a seasoned web developer, a personal blogger, or a business owner, we’ve kept you in mind when designing our hosting services.
We provide everything you need, from developer-made tools like Git integration and access managers for user management to basic features like a free domain name, unlimited bandwidth, and free SSL.
Make the switch to ASPHostPortal right now to see the difference for yourself.
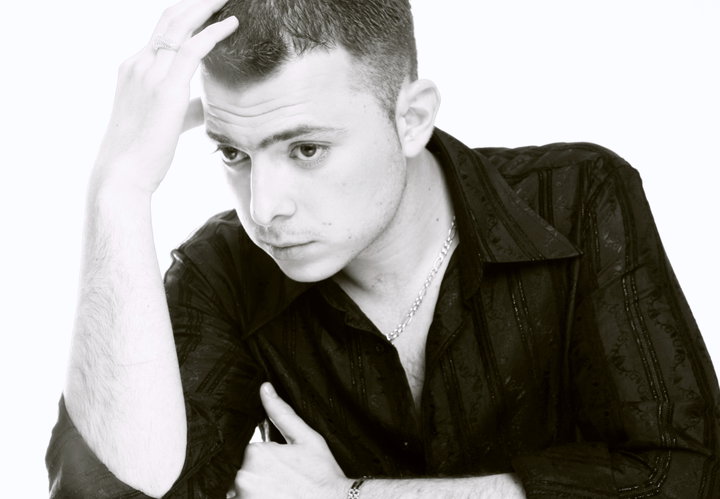
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.