What is meant by caching? A basic explanation of the caching mechanism is given, along with instructions on how to implement it using the ASP.NET Core API.
Introduction
The quantity of thumbnails and calculations involved in recent projects has prevented each screen from rendering at the appropriate speed. To improve data reading speed, other optimizations such as lazy loading and T-SQL can be applied in addition to the own code optimization.
Caching is another technique that can be utilized in addition to these to improve performance. This article describes how to set up a caching mechanism using the ASP.NET Core API and the Blazor WASM architecture.
Caching Mechanism
Caching is the process of using or reading data that has already been stored. The speed can be effectively increased and the website’s load can be decreased because there is no need to re-obtain files from the database or server.
Fluency issues will unavoidably arise if the website is static, requires a lot of static resources, or requires the resources to load each time a user visits the website. In this instance, transmission speed and static resource performance can be effectively increased by using a CDN (Content Delivery Network) service, which operates over a global network made up of server nodes. Caching technology makes use of pre-stored static resources across multiple locations to achieve efficient transmission.
By pre-storing data in memory, a similar caching mechanism can be implemented within the backend API to enable efficient data transmission. The purpose of this article is to explain how to use the MemoryCache
class for caching. The IDistributedCache
class can be used for distributed systems or larger websites, but it might take me more time to comprehend and implement.
Situations for appropriate usage
Since the data is stored in memory, if you choose to use the MemoryCache
class for caching, it will vanish if the application is closed or restarted, the system malfunctions, or the system manages and optimizes the memory. As a result, it is not advised to use caching to store crucial data or data that changes regularly as this could lead to inaccurate website content information.
Using this article as an example, the homepage of the project website will feature a cover image. Pre-storing the data in the cache can help users load the homepage more quickly because the cover image is not updated often and is stored in both the database and ASPHostPortal.
Code
Regardless of whether it is a Blazor WASM or a general Web API, the architecture modifies the controller’s API. Code appears as follows:
using Microsoft.Extensions.Caching.Memory; namespace BlazorApp.Server.Controllers { [Route("api/[controller]")] [ApiController] public class StorageController : ControllerBase { private readonly IStorageService _storageService; private readonly IMemoryCache _cache; public StorageController(IStorageService storageService, IMemoryCache memoryCache) { _storageService = storageService; _cache = memoryCache; } [HttpGet("logo/admin-no-cache")] public async Task<List<S3FileInfo>> GetLogoFileNoCache() { List<S3FileInfo> FileInfo = await _storageService.GetFileByKeyAsync(); return FileInfo; } [HttpGet("logo")] public async Task<List<S3FileInfo>> GetLogoFile() { string cacheKey = "logo"; if (!_cache.TryGetValue<List<S3FileInfo>>(cacheKey, out List<S3FileInfo> cachedData)) { List<S3FileInfo> FileInfo = await _storageService.GetFileByKeyAsync(); cachedData = FileInfo; _cache.Set(cacheKey, cachedData, TimeSpan.FromHours(24)); } return cachedData; } } }
Administrators use the logo/admin-no-cache
API, one of the two available. Administrators must verify the data’s accuracy right away because it is a no-cache version.
When the home page loads, the other one is the logo
. Setting the cache key to the logo
makes sense. You must verify whether data with the key logo
is in the cache each time you call.
Use it if it’s there; if not, get the information.
After the data is obtained, store it in the cache and set its expiration date to 24 hours in _cache.Set(cacheKey, cachedData, TimeSpan.FromHours(24))
. As a result, the cache will clear every 24 hours, causing the first visitor to the website to load more slowly as the data needs to be retrieved. After that, each subsequent user will still load the page just as quickly and efficiently.
Remove cache
Clearing the cache is sometimes necessary, for example, to enable users to view content that is updated in real time.
Eliminate particular cache codes:
_cache.Remove("logo");
Remove all cache codes:
_cache.Dispose();
Conclusion
This is the way that the memory caching mechanism can be implemented using ASP.NET Core. Since it’s simpler to categorize, I personally split the data that needs to be cached into two APIs. Actually, by changing the API conditions, you can choose whether to retrieve actual data or data that has been cached. I think the website can save a lot of money on data transmission if it makes good use of cache.
Every successful website is built on a strong ASP.NET hosting infrastructure. Without having to constantly worry about technical failure, it enables you to deliver content and connect with the greatest number of potential customers.
ASPHostPortal has you covered if you’re looking for a dependable hosting solution. Our hosting offers market-leading performance, a 100% uptime guarantee, and first-rate technical support.
We also provide a variety of hosting packages at reasonable prices, including shared, managed, VPS, cloud, and more. To see how simple it can be to host a website online, sign up right away.
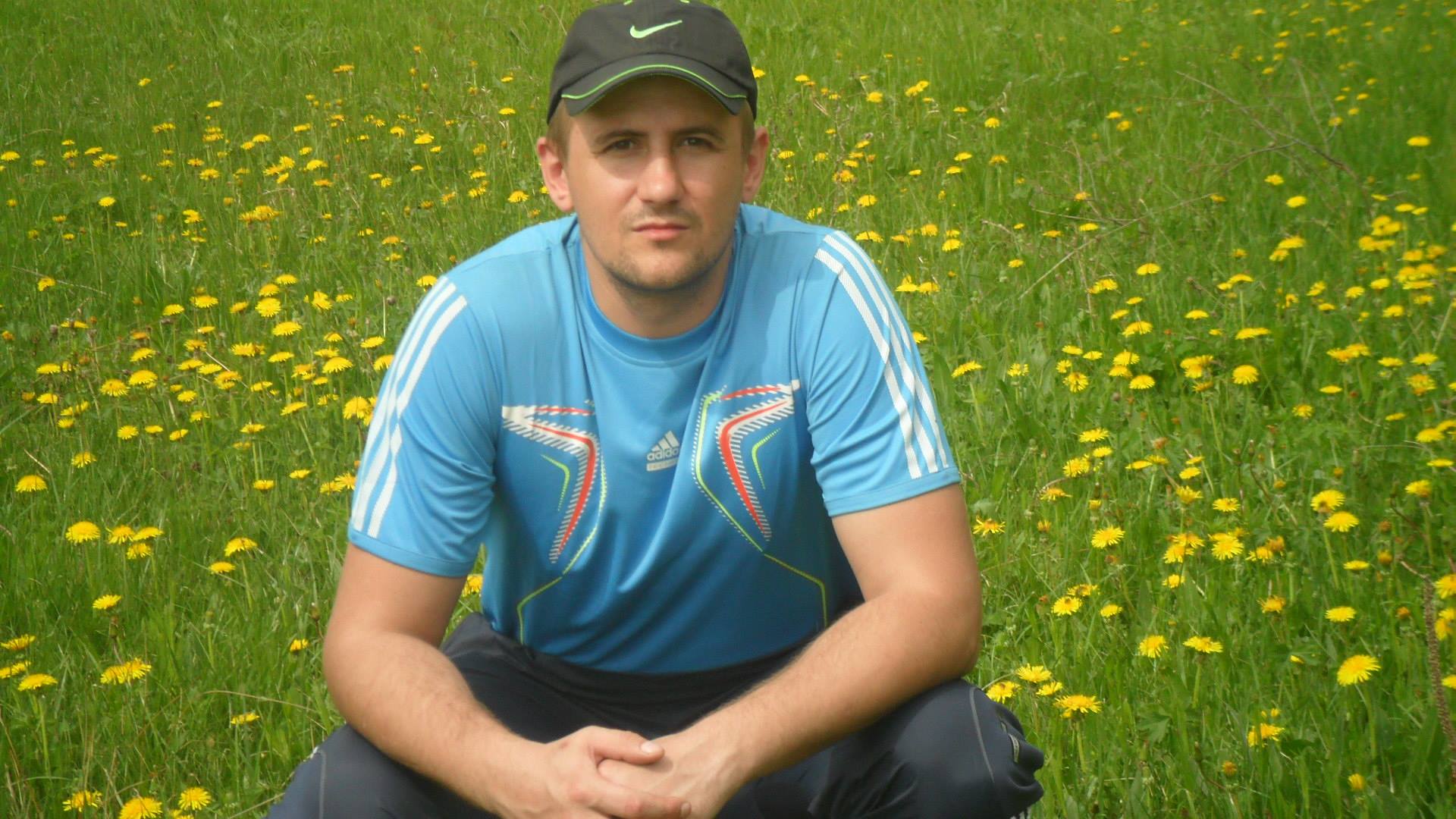
Yury Sobolev is Full Stack Software Developer by passion and profession working on Microsoft ASP.NET Core. Also he has hands-on experience on working with Angular, Backbone, React, ASP.NET Core Web API, Restful Web Services, WCF, SQL Server.