Improving the performance of an ASP.NET Core application is crucial for delivering a fast and responsive user experience. One of the most effective tools for identifying and resolving performance bottlenecks is a .NET Profiler. A profiler helps you analyze your application’s runtime behavior, pinpoint inefficiencies, and optimize code for better performance. In this guide, we’ll explore how to use a .NET Profiler to increase the performance of your ASP.NET Core application.
What is a .NET Profiler?
A .NET Profiler is a tool that monitors the execution of a .NET application and provides detailed insights into its performance. It helps you:
- Identify slow methods and database queries.
- Analyze memory usage and garbage collection.
- Detect CPU and I/O bottlenecks.
- Measure the impact of specific code changes.
Popular .NET Profilers include:
- JetBrains dotTrace
- Redgate ANTS Performance Profiler
- Visual Studio Diagnostic Tools
- MiniProfiler (lightweight and open-source
Steps to Increase ASP.NET Core Performance Using a .NET Profiler
1. Set Up Your Profiler
Before you start profiling, ensure your ASP.NET Core application is configured for performance analysis:
- Debug Build: Use a debug build for detailed profiling, but also test with a release build to simulate production conditions.
- Profiler Integration: Install and configure your chosen profiler. For example:
- For dotTrace, install the JetBrains profiler and attach it to your ASP.NET Core process.
- For MiniProfiler, add the
MiniProfiler.AspNetCore
NuGet package and configure it inStartup.cs
.
2. Profile Your Application
Run your application with the profiler attached and simulate real-world usage:
- Load Testing: Use tools like Apache JMeter or k6 to generate traffic and simulate user behavior.
- Scenario-Based Profiling: Focus on specific scenarios (e.g., user login, product search) to identify bottlenecks.
3. Analyze Performance Data
Once the profiler collects data, analyze the results to identify performance issues:
- Slow Methods: Look for methods with high execution times or CPU usage.
- Memory Usage: Identify memory leaks or excessive allocations.
- Database Queries: Check for slow or inefficient database queries.
- I/O Operations: Identify bottlenecks in file or network I/O.
4. Optimize Code and Configuration
Based on the profiler’s findings, implement optimizations:
a. Optimize Database Queries
- N+1 Queries: Use eager loading (e.g.,
Include
in Entity Framework) to reduce the number of database queries. - Indexing: Ensure proper indexing for frequently queried columns.
- Caching: Cache frequently accessed data using
MemoryCache
orDistributedCache
.
b. Reduce Memory Usage
- Object Pooling: Reuse objects instead of creating new ones (e.g., use
ArrayPool<T>
for arrays). - Dispose Resources: Properly dispose of unmanaged resources (e.g., database connections, file streams).
- Garbage Collection: Minimize allocations in hot paths to reduce GC pressure.
c. Improve CPU Efficiency
- Asynchronous Programming: Use
async
/await
to avoid blocking threads. - Parallelism: Use
Parallel.ForEach
orTask.WhenAll
for CPU-bound tasks. - Algorithm Optimization: Replace inefficient algorithms with faster alternatives.
d. Optimize I/O Operations
- Batching: Batch I/O operations to reduce overhead (e.g., batch database inserts).
- Buffering: Use buffered streams for file or network I/O.
- Compression: Compress data to reduce network transfer times.
5. Validate Improvements
After implementing optimizations, re-run the profiler to validate the changes:
- Compare performance metrics (e.g., response time, memory usage) before and after optimization.
- Ensure no new bottlenecks have been introduced.
6. Monitor in Production
Profiling in development is essential, but real-world performance can differ. Use monitoring tools to track performance in production:
- Application Insights: Monitor request rates, response times, and exceptions.
- Prometheus + Grafana: Set up custom dashboards for performance metrics.
- Health Checks: Use ASP.NET Core’s health checks to monitor critical components.
Best Practices for Profiling ASP.NET Core Applications
1. Profile Early and Often
- Integrate profiling into your development workflow to catch performance issues early.
- Use lightweight profilers like MiniProfiler for continuous monitoring.
2. Focus on Hot Paths
- Prioritize optimizing code that runs frequently or has a significant impact on performance.
- Use the Pareto Principle (80/20 rule): 20% of the code often accounts for 80% of the performance issues.
3. Simulate Real-World Conditions
- Profile under realistic workloads to identify bottlenecks that only occur under stress.
- Use load testing tools to simulate high traffic.
4. Avoid Premature Optimization
- Focus on optimizing code that has been proven to be a bottleneck through profiling.
- Avoid over-optimizing code that has minimal impact on performance.
5. Leverage Built-In Tools
- Use ASP.NET Core’s built-in diagnostics tools (e.g., logging, middleware) to complement profiler data.
- Enable Kestrel’s request logging to track request/response times.
6. Collaborate with Your Team
- Share profiling results with your team to prioritize performance improvements.
- Use profiling data to guide code reviews and architectural decisions.
Example: Using MiniProfiler in ASP.NET Core
Here’s how to set up and use MiniProfiler to profile an ASP.NET Core application:
Step 1: Install MiniProfiler
dotnet add package MiniProfiler.AspNetCore dotnet add package MiniProfiler.EntityFrameworkCore
Step 2: Configure MiniProfiler
In Startup.cs
:
public void ConfigureServices(IServiceCollection services) { services.AddMiniProfiler(options => { options.RouteBasePath = "/profiler"; options.EnableEntityFrameworkTracking(); // Track EF Core queries }).AddEntityFramework(); } public void Configure(IApplicationBuilder app, IHostingEnvironment env) { app.UseMiniProfiler(); }
Step 3: Add Profiling to Your Code
Wrap code blocks you want to profile:
using (MiniProfiler.Current.Step("GetProducts")) { var products = _dbContext.Products.ToList(); }
Step 4: View Profiling Results
Run your application and navigate to /profiler
to view detailed profiling data.
Conclusion
Using a .NET Profiler is one of the most effective ways to increase the performance of your ASP.NET Core application. By identifying bottlenecks, optimizing code, and validating improvements, you can deliver a faster and more responsive application. Whether you choose a full-featured profiler like dotTrace or a lightweight tool like MiniProfiler, integrating profiling into your development process will help you build high-performance applications that scale with your users’ needs.
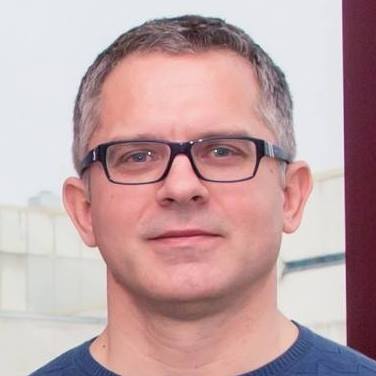
Javier is Content Specialist and also .NET developer. He writes helpful guides and articles, assist with other marketing and .NET community work