Performance and resource optimization are now requirements rather than just options in application development. This is where the asp.net core or any other framework’s caching comes into play, providing a reliable way to improve application performance by storing copies of data momentarily.
I’ll go into great detail about the types, implementation, significance, and best practices of the caching options in ASP.NET Core in this article.
Understanding Caching and Its Significance
The act of keeping data in a cache—a temporary storage area—is referred to as caching. This method is essential for cutting down on the time and resources needed to retrieve data from the main storage each time a request is made. Caching is essential to the ASP.NET Core for the following reasons:
- Minimizing Database Load: The amount of direct database queries is decreased by keeping frequently accessed data in the cache, which lessens the database’s load.
- Enhancing Response Time: Response times are accelerated because data from the cache is retrieved much more quickly than from a slower storage component.
Types of Caching in ASP.NET Core
Three types of caching are primarily supported by.NET Core:
- In-Memory Caching: The web server’s memory is used for data storage in this most basic form of caching. Its speed is unmatched, but it is limited to the memory of a single server.
- Distributed Caching: Distributed caching, as opposed to in-memory caching, enables the cache to be shared among several servers. This is especially helpful when the application is spread across several servers in a microservices architecture. Popular options for distributed caching in.NET Core are Redis and SQL Server.
- Response Caching: This kind entails caching a web request’s response. For web pages or web APIs where the content is static, it’s especially helpful.
Implementing Caching in ASP.NET Core
Let’s examine each type of caching in more detail and see how it can be applied to an ASP.NET Core application.
I prefer to create a single interface that describes how the cache works before getting into the implementation details for each type. Using this method, I can switch between various caching strategies without interfering with the application’s functionality.
namespace NhaCard.Application.Cache { public interface ICacheService { Task<T> GetOrCreateAsync<T>( string cacheKey, Func<Task<T>> retrieveDataFunc, TimeSpan? slidingExpiration = null); } }
In-Memory Caching
You can use the IMemoryCache
interface to implement in-memory caching. Here’s an introductory example of its use:
using Microsoft.Extensions.Caching.Memory;
namespace NhaCard.Application.Cache
{
public class NhaCardCacheService : ICacheService
{
private readonly IMemoryCache _cache;
public NhaCardCacheService(IMemoryCache cache) => _cache = cache;
public async Task<T> GetOrCreateAsync<T>(
string cacheKey,
Func<Task<T>> retrieveDataFunc,
TimeSpan? slidingExpiration = null)
{
if (!_cache.TryGetValue(cacheKey, out T cachedData))
{
// Data not in cache, retrieve it
cachedData = await retrieveDataFunc();
// Set cache options
var cacheEntryOptions = new MemoryCacheEntryOptions
{
SlidingExpiration = slidingExpiration ?? TimeSpan.FromMinutes(60)
};
// Save data in cache
_cache.Set(cacheKey, cachedData, cacheEntryOptions);
}
return cachedData;
}
}
}
For more info about ‘MemoryCacheEntryOptions’
In your Program.cs
, add the following lines to set up and use Serilog:
builder.Services.AddMemoryCache(); builder.Services.AddScoped<ICacheService, NhaCardCacheService>();
Your cache engine is primed and ready to run if you follow these simple steps. See it in operation, please.
private async Task LoadSpecialityData()
{
var cachedSpecialties = await _cacheService.GetOrCreateAsync(
loadSpecialtyCacheKey,
async () =>
{
//The structureId parameter is left blank to enable the loading of all specialties, regardless of the health structure.
var result = await _client.PostLoadSpecialtyAsync("");
if (result.IsSuccess)
{
return result.Value;
}
else
{
throw new Exception("Error on loading speciality: " + result.Error);
}
});
cachedSpecialties.ForEach(s =>
{
MedicalSpecialisationSelectListItems.Add(new SelectListItem
{
Text = s.Name,
Value = s.Id.ToString(),
});
});
}
Distributed Caching
ASP.NET Core offers an IDistributedCache
interface for distributed caching. Here’s a sample showing how to use it with Redis:
You must install the following package in order to use Redis cache in =application:
dotnet add package Microsoft.Extensions.Caching.StackExchangeRedis
dotnet add package Microsoft.Extensions.Caching.Abstractions
Use the ‘AddStackExchangeRedisCache’ method in your Program.cs
file to add distributed Redis cache middleware.
// Configure Redis
builder.Services.AddStackExchangeRedisCache(options =>
{
options.Configuration = "localhost:6379"; // Update with your Redis server configuration
options.InstanceName = "NhaCardUI"; // Optional
});
// Add the cache service
builder.Services.AddScoped<ICacheService, NhaCardCacheService>();
Now, proceed to implement the ICacheService interface as follows:
using Microsoft.Extensions.Caching.Distributed;
using System.Text.Json;
namespace NhaCard.Application.Cache
{
internal class NhaCardDistributedCacheService : ICacheService
{
private readonly IDistributedCache _cache;
public NhaCardDistributedCacheService(IDistributedCache cache) => _cache = cache;
public async Task<T> GetOrCreateAsync<T>(
string cacheKey,
Func<Task<T>> retrieveDataFunc,
TimeSpan? slidingExpiration = null)
{
// Try to get the data from the cache
var cachedDataString = await _cache.GetStringAsync(cacheKey);
if (!string.IsNullOrEmpty(cachedDataString))
{
return JsonSerializer.Deserialize<T>(cachedDataString);
}
// Data not in cache, retrieve it
T cachedData = await retrieveDataFunc();
// Serialize the data
var serializedData = JsonSerializer.Serialize(cachedData);
// Set cache options
var cacheEntryOptions = new DistributedCacheEntryOptions
{
SlidingExpiration = slidingExpiration ?? TimeSpan.FromMinutes(60)
};
// Save data in cache
await _cache.SetStringAsync(cacheKey, serializedData, cacheEntryOptions);
return cachedData;
}
}
}
For more info about ‘DistributedCacheEntryOptions’
Response Caching
You can use the Program.cs
file’s middleware to implement response caching. By using the ResponseCache
attribute on your action methods, you can set the caching behavior.
// Configure
builder.services.AddResponseCaching();
...
//Use
app.UseResponseCaching();
//In you controller use this atribute
[HttpGet]
[ResponseCache(Duration = 60)]
public IActionResult GetLoadSpecialityData()
{
// Action code here
}
That’s it.
Conclusion
Although caching can greatly improve performance, it’s important to adhere to best practices to stay clear of typical pitfalls:
- Cache Invalidation: To make sure that the cache doesn’t serve outdated data, implement a strong cache invalidation strategy.
- Memory Management: Use memory carefully, especially when using in-memory caching. Set and keep an eye on the proper size limits.
- Secure Sensitive Data: Make sure the sensitive data you’re caching is secure and encrypted.
- Evaluate Cache Efficiency: Maintain a regular check on your cache’s efficacy to make sure it’s enhancing performance as planned.
To sum up, caching is an effective method for enhancing application performance in the ASP.NET Core ecosystem. Your applications will run much faster and more efficiently if you know about the various kinds of caching and know how to use them. However, keep in mind that in order to fully benefit from caching, as with any powerful tool, it must be used carefully and managed.
ASPHostPortal is one of the best alternatives for your ASP.NET hosting, offering:
- Better pricing and value for money
- Feature-rich hosting plans
- 99.9% uptime guarantee
- Enhanced security features
- Global data center locations
- Multilingual customer support
Whether you’re a seasoned web developer, a personal blogger, or a business owner, we’ve kept you in mind when designing our hosting services.
We provide everything you need, from developer-made tools like Git integration and access managers for user management to basic features like a free domain name, unlimited bandwidth, and free SSL.
Make the switch to ASPHostPortal right now to see the difference for yourself.
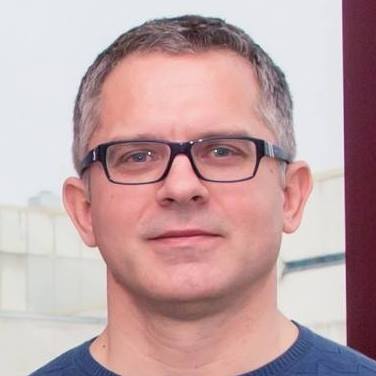
Javier is Content Specialist and also .NET developer. He writes helpful guides and articles, assist with other marketing and .NET community work