In previous article, we have written few articles about error that you might find when deploying your Asp.net Core application. In this article, we will continue other error, something like returning HTTP Status code 500 internal server error etc.
Example: One may return Web API with ASP.NET Core MVC 200, 500 or 503 error codes
RESTFul services principle talks about HTTTP Status code and their usage. It’s important to follow these REST HTTP Status codes appropriately when following REST specifications.
.NET Core has inbuilt support for all basic HTTP Status code and they are easy to configure for almost all standard HTTP status code.
Built-in Types for.NET Core
Status Code – 200 (OK)
- The 200 (OK) status code indicates success.
- This response also meant the response has the payload.
- If no payload is desired, the server should send a 204 (NoContent) status code.
One can use built-in type Ok() as below,
[HttpGet]
public
IActionResult GetSynchrounous(
string
id)
{
Book book =
null
;
//run method - Synchronously
book = _bookService.Get(id);
return
Ok(book);
}
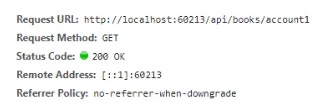
Status Code – 400 (Bad Request)
This status code means the server cannot process the request due to malformed requests, invalid requests, etc.
One can use built-in type BadRequest() as below,
Example:
[HttpGet(
"{id}"
)]
public
IActionResult GetAccount(
string
id)
{
if
(id ==
null
)
{
return
BadRequest();
}
return
Ok();
}
Below is the response for the above request (Using Chrome) will produce Status Code: 400 Bad Request,
Request URL: http://localhost:60213/api/books/1
Request Method: GET
Status Code: 400 Bad Request
Remote Address: [::1]:60213
Referrer Policy: no-referrer-when-downgrade
This built-in type does support sending additional details with its overloaded API.
Status Code – 500 (InternalServerError )
This status code means the server encountered an unexpected condition that prevented it from fulfilling the input request.
One can use built-in type StatusCode() as below,
Example:
public
IActionResult GetAccount1(
string
id)
{
Book book =
null
;
try
{
//run method - Synchronously
book = _bookService.Get(id);
if
(book ==
null
)
{
return
NotFound();
}
book.Price = 10;
}
catch
(Exception ex)
{
return
StatusCode(500,
"Internal Server Error. Somthing went Wrong!"
);
}
return
Ok(book);
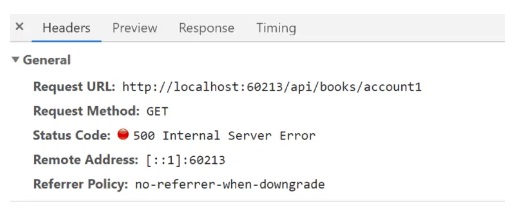
This built-in type does support sending additional details with its overloaded API.
- StatusCode( int statusCode, object value);
- StatusCode( int statusCode);
StatusCode can be used as an alternative for all other HTTP status codes where the built-in type is missing.
StatusCode class cab be used for all below-supported codes,
public
static
class
StatusCodes
{
public
const
int
Status100Continue = 100;
public
const
int
Status412PreconditionFailed = 412;
public
const
int
Status413PayloadTooLarge = 413;
public
const
int
Status413RequestEntityTooLarge = 413;
public
const
int
Status414RequestUriTooLong = 414;
public
const
int
Status414UriTooLong = 414;
public
const
int
Status415UnsupportedMediaType = 415;
public
const
int
Status416RangeNotSatisfiable = 416;
public
const
int
Status416RequestedRangeNotSatisfiable = 416;
public
const
int
Status417ExpectationFailed = 417;
public
const
int
Status418ImATeapot = 418;
public
const
int
Status419AuthenticationTimeout = 419;
public
const
int
Status421MisdirectedRequest = 421;
public
const
int
Status422UnprocessableEntity = 422;
public
const
int
Status423Locked = 423;
public
const
int
Status424FailedDependency = 424;
public
const
int
Status426UpgradeRequired = 426;
public
const
int
Status428PreconditionRequired = 428;
public
const
int
Status429TooManyRequests = 429;
public
const
int
Status431RequestHeaderFieldsTooLarge = 431;
public
const
int
Status451UnavailableForLegalReasons = 451;
public
const
int
Status500InternalServerError = 500;
public
const
int
Status501NotImplemented = 501;
public
const
int
Status502BadGateway = 502;
public
const
int
Status503ServiceUnavailable = 503;
public
const
int
Status504GatewayTimeout = 504;
public
const
int
Status505HttpVersionNotsupported = 505;
public
const
int
Status506VariantAlsoNegotiates = 506;
public
const
int
Status507InsufficientStorage = 507;
public
const
int
Status508LoopDetected = 508;
public
const
int
Status411LengthRequired = 411;
public
const
int
Status510NotExtended = 510;
public
const
int
Status410Gone = 410;
public
const
int
Status408RequestTimeout = 408;
public
const
int
Status101SwitchingProtocols = 101;
public
const
int
Status102Processing = 102;
public
const
int
Status200OK = 200;
public
const
int
Status201Created = 201;
public
const
int
Status202Accepted = 202;
public
const
int
Status203NonAuthoritative = 203;
public
const
int
Status204NoContent = 204;
public
const
int
Status205ResetContent = 205;
public
const
int
Status206PartialContent = 206;
public
const
int
Status207MultiStatus = 207;
public
const
int
Status208AlreadyReported = 208;
public
const
int
Status226IMUsed = 226;
public
const
int
Status300MultipleChoices = 300;
public
const
int
Status301MovedPermanently = 301;
public
const
int
Status302Found = 302;
public
const
int
Status303SeeOther = 303;
public
const
int
Status304NotModified = 304;
public
const
int
Status305UseProxy = 305;
public
const
int
Status306SwitchProxy = 306;
public
const
int
Status307TemporaryRedirect = 307;
public
const
int
Status308PermanentRedirect = 308;
public
const
int
Status400BadRequest = 400;
public
const
int
Status401Unauthorized = 401;
public
const
int
Status402PaymentRequired = 402;
public
const
int
Status403Forbidden = 403;
public
const
int
Status404NotFound = 404;
public
const
int
Status405MethodNotAllowed = 405;
public
const
int
Status406NotAcceptable = 406;
public
const
int
Status407ProxyAuthenticationRequired = 407;
public
const
int
Status409Conflict = 409;
public
const
int
Status511NetworkAuthenticationRequired = 511;
}
Summary
It’s important to follow HTTP Status codes appropriately when following REST specifications for any communication we do use WebAPI or HTTPClient objects. Today we learned that .NET Core has support for almost all HTTP status codes which we can rely on and they are easy to use.
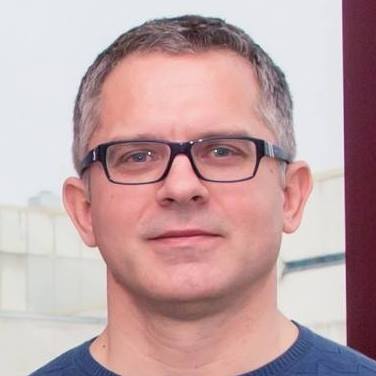
Javier is Content Specialist and also .NET developer. He writes helpful guides and articles, assist with other marketing and .NET community work