This article demonstrates how to use Message Pack as the protocol and SignalR to send messages between various C# console clients. To host the SignalR Hub, an ASP.NET Core web application is utilized.
Configuring the SignalR Message Pack server
To the ASP.NET Core server application hosting the SignalR Hub, add the Microsoft.AspNetCore.SignalR and Microsoft.AspNetCore.SignalR.MsgPack NuGet packages. You can accomplish this by using the Visual Studio NuGet Package Manager.
You can also just add it straight to the project file (.csproj).
<PackageReference Include="Microsoft.AspNetCore.SignalR" Version="1.1.0" /> <PackageReference Include="Microsoft.AspNetCore.SignalR.Protocols.MessagePack" Version="7.0.1" />
Establish a SignalR Hub as necessary. The Hub class is implemented to accomplish this.
using Microsoft.AspNetCore.SignalR; namespace AspNetCoreAngularSignalR.SignalRHubs; public class LoopyHub : Hub { public Task Send(string data) { return Clients.All.SendAsync("Send", data); } }
To transmit the Message Pack messages, a DTO class is made. You’ll see that the class lacks any Message Pack properties or attributes and is just a standard C# class.
namespace Dtos; public class MessageDto { public Guid Id { get; set; } public string Name { get; set; } = string.Empty; public int Amount { get; set; } }
Then add the Message Pack protocol to the SignalR service.
services.AddSignalR().AddMessagePackProtocol();
app.MapHub<LoopyHub>("/loopy"); app.MapHub<NewsHub>("/looney"); app.MapHub<LoopyMessageHub>("/loopymessage"); app.MapHub<ImagesMessageHub>("/zub");
Configuring the SignalR Message Pack client
To the SignalR client console application, add the Microsoft.AspNetCore.SignalR.Client and Microsoft.AspNetCore.SignalR.Client.MsgPack NuGet packages.
The packages are added to the project file.
<PackageReference Include="Microsoft.AspNetCore.SignalR.Client" Version="7.0.1" /> <PackageReference Include="Microsoft.AspNetCore.SignalR.Protocols.MessagePack" Version="7.0.1" />
public static async Task SetupSignalRHubAsync() { _hubConnection = new HubConnectionBuilder() .WithUrl("https://localhost:44324/loopymessage") .AddMessagePackProtocol() .ConfigureLogging(factory => { factory.AddConsole(); factory.AddFilter("Console", level => level >= LogLevel.Trace); }).Build(); await _hubConnection.StartAsync(); }
static async Task MainAsync() { await SetupSignalRHubAsync(); if(_hubConnection != null ) { _hubConnection.On<MessageDto>("Send", (message) => { Console.WriteLine($"Received Message: {message.Name}"); }); Console.WriteLine("Connected to Hub"); Console.WriteLine("Press ESC to stop"); do { while (!Console.KeyAvailable) { var message = Console.ReadLine(); if (message != null) { await _hubConnection.SendAsync("Send", new MessageDto { Id = Guid.NewGuid(), Name = message, Amount = 7 }); } Console.WriteLine("SendAsync to Hub"); } } while (Console.ReadKey(true).Key != ConsoleKey.Escape); } }
Testing
Launch the two console apps and the server application. After that, SignalR messages—which employ Message Pack as their protocol—can be sent and received.
Conclusion
Now, you have learned about how to use Message Pack in ASP.NET Core SignalR. We hope you enjoy this article and you can always share this tutorial to others.
Do you want to develop enterprise apps that run on multiple platforms? Are you looking to host your .NET Core SignalR applications?
ASPHostPortal is one of the best alternatives for your ASP.NET SignalR hosting, offering:
- Better pricing and value for money
- Feature-rich hosting plans
- 99.9% uptime guarantee
- Enhanced security features
- Global data center locations
- Multilingual customer support
Whether you’re a seasoned web developer, a personal blogger, or a business owner, we’ve kept you in mind when designing our hosting services.
We provide everything you need, from developer-made tools like Git integration and access managers for user management to basic features like a free domain name, unlimited bandwidth, and free SSL.
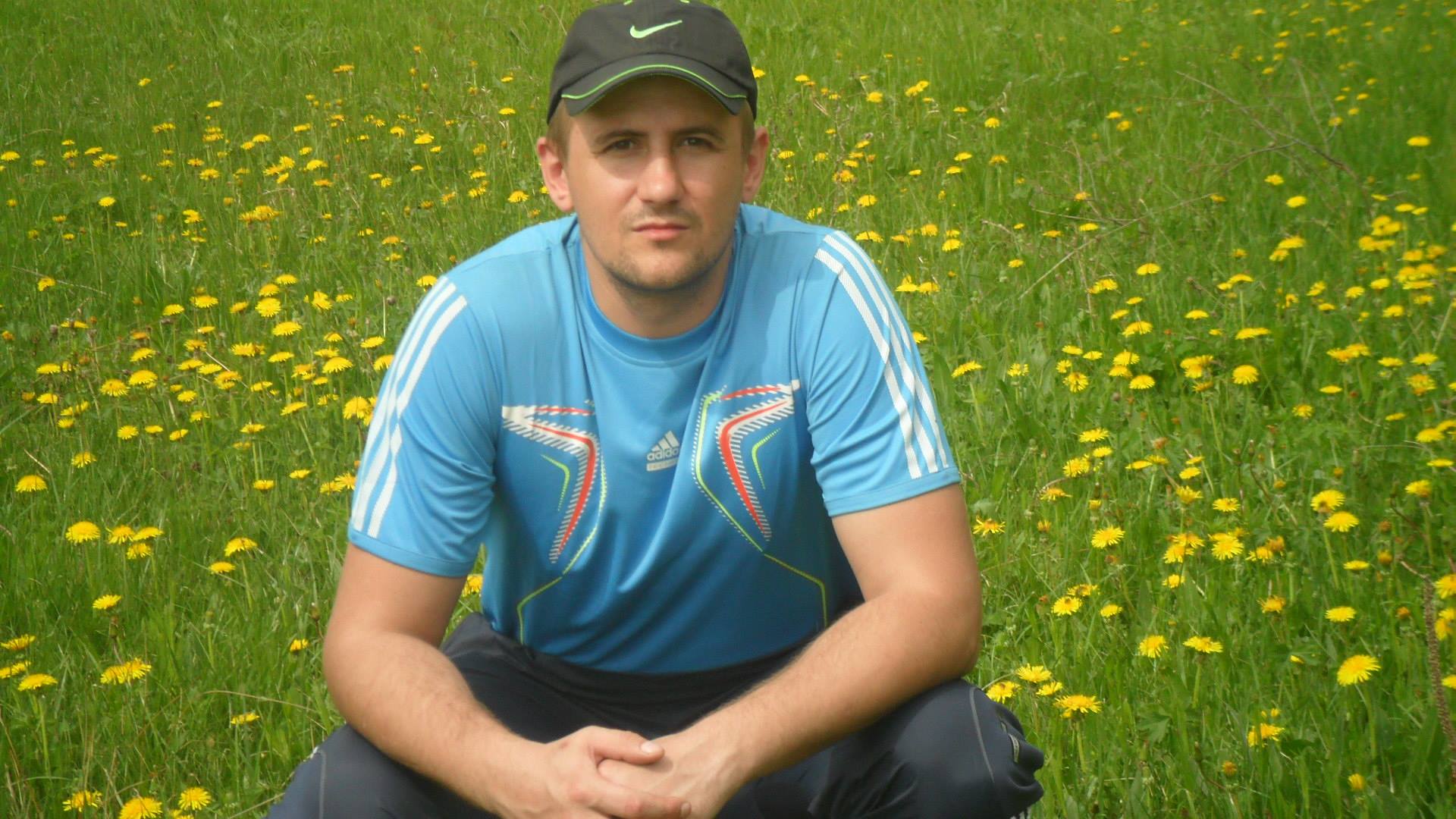
Yury Sobolev is Full Stack Software Developer by passion and profession working on Microsoft ASP.NET Core. Also he has hands-on experience on working with Angular, Backbone, React, ASP.NET Core Web API, Restful Web Services, WCF, SQL Server.