Being able to restart an ASP.NET Core web application programmatically can be very useful, you may also read our previous post about how to publish Asp.net core in IIS.
In this post we’ll deal with one of them, which is strongly related to the deployment/publishing phase of your web application, updating or overwriting the web.config file won’t cause the Kestrel web server to always recycle/restart the app and reload the updated assemblies: this basically means that, whenever you deploy, you’ll often need to restart the whole Kestrel service to replace the old assemblies with the new ones.
In order to fix that I took advantage of the IApplicationLifetime object, which exposes a very handy Shutdown() method that does exactly what its name suggests: since it’s also injectable, we can easily use it on any controller through DI and then use it to implement an action method that would issue a remote restart through the web.
Here’s a sample controller that does just that:
[Authorize(Roles = "Admin")] public class AdminController : Controller { private IApplicationLifetime ApplicationLifetime { get; set; } public AdminController(IApplicationLifetime appLifetime) { ApplicationLifetime = appLifetime; } public IActionResult Shutdown() { ApplicationLifetime.StopApplication(); return Content("Done"); } }
Needless to say, it’s wise to protect such method – or the whole controller, like I did in the above example – with an appropriate [Authorize] attribute or any other measure to prevent it to be called from standard users and/or malevolent third-parties: in the above scenario, only authenticated administrators (the “Admin” membership role) would be able to use it.
Conclusion
That’s it, at least for now: I sincerely hope that this small guide will be useful to those ASP.NET Core developers who are looking for a way to restart/recycle their web application programmatically and/or from a remote request.
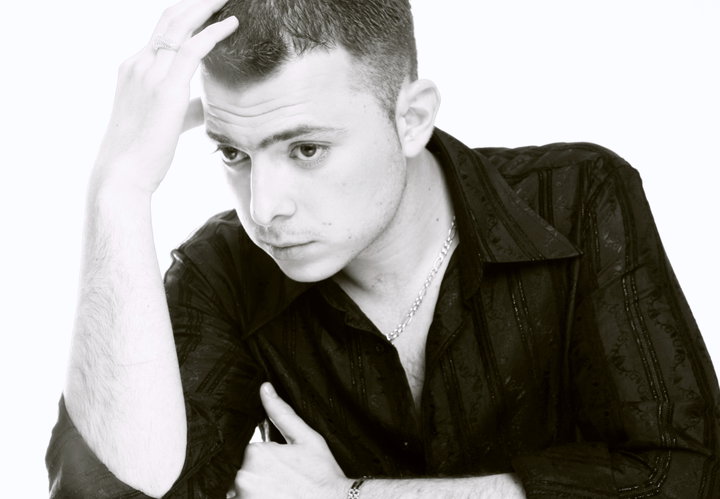
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.