For some reason, this particular error seems to just confuse the stuffing out of ASP.NET MVC developers in particular. Granted, it’s not one of the most obvious or informative errors that you can receive, but it’s actually pretty self-explanatory, once you actually know what it’s trying to tell you.
Simply, you receive this exception when you reference a property or method on an object instance that resolves to null at runtime. Let’s see an example:
Entity
public class Foo { public int Id { get; set; } public string Bar { get; set; } }
Action
public ActionResult GetFoo(int id) { var foo = db.Foos.Find(id); return View(foo); }
View
@model Foo <p>Bar: @Model.Bar</p>
Everything looks kosher, so far. We pull the Foo
instance with the matching id from the database and pass it to the view. In the view, we reference the Bar
property, which intellisense is fine with because Bar
is a property of Foo
. However, what happens if no Foo
with that id can be found in our database? If that’s the case, the value of our foo
variable is actually null, and guess what? There’s no Bar
property on null
. What should have been a perfectly valid reference is now invalid because the “object reference”, which is foo
here, is “not set to an instance of an object” (Foo
), but rather is null.
If you receive this exception, you’re being told that somewhere in your code you’ve done something like the above: reference a property on what you expect to be an object instance, but which actually resolves to null. The solution is to do proper null-checking. In the above example, we could have prevented this error by returning a 404:
var foo = db.Foos.Find(id); if (foo == null) { return new HttpNotFoundResult(); } return View(foo);
Then, if foo
ends up as null, the user never hits the view which references its Bar
property.
This isn’t always appropriate, though. Sometimes you’ll have to just only take some action if you know you have a valid instance:
if (foo != null) { // Do something with `foo.Bar` }
You can also use a ternary to provide a default:
<p>Bar: @(Model != null ? Model.Bar : "")</p>
Then, if you can safely reference Bar
, its value will be output. Otherwise, you’ll get an empty string.
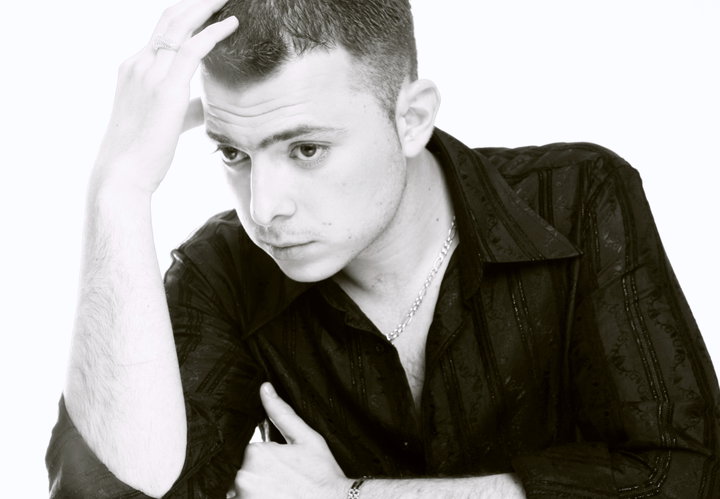
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.