Razor files (.cshtml) are compiled at both build and publish time and this gives better performance as your views are compiled. We can also enable runtime compilation, which will help developers to see any modified view change in real-time, without starting the application again. The recent version of ASP.NET Core (ASP.NET Core 5.0) Preview 2 came out and you can start building an app on ASP.NET Core 5.0 framework. ASP.NET Core 5.0 changes this experience for enabling razor runtime compilation. This is now available as an option while creating the ASP.NET Core 5.0 project. It’s a tiny enhancement, but good to know.
To enable Razor runtime compilation till ASP.NET Core 3.1
Till now, if you need to enable Razor runtime compilation, you will follow the below steps.
- Install the
Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation
NuGet package. - Update the project’s
Startup.ConfigureServices
method to include a call toAddRazorRuntimeCompilation
.
public
void
ConfigureServices(IServiceCollection services)
{
services.AddControllersWithViews()
.AddRazorRuntimeCompilation();
}
To enable Razor runtime compilation in ASP.NET Core 5.0
- First, to use ASP.NET Core in .NET 5.0 Preview2 install the .NET 5.0 SDK.
- Install the latest preview of Visual Studio 2019 16.6.
With ASP.NET Core 5, to enable razor file compilation, there is no need to explicitly add Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation
NuGet package. When you create a new project targeted to ASP.NET Core 5, Visual Studio 2019 provides an option to enable this feature. Like,
When you check this option, Visual Studio 2019 will add this package for you in dependencies.
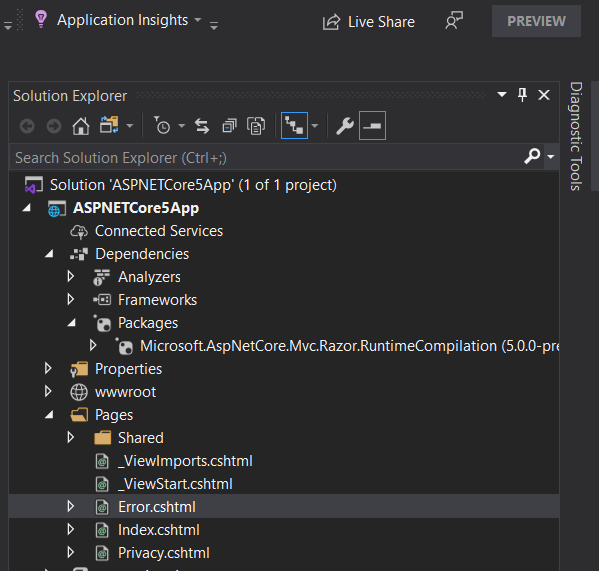
services.AddControllersWithViews()
.AddRazorRuntimeCompilation();
With ASP.NET core 5.0, this is handled via launchsetting.json
file. You will see the following content in the launchsetting.json
file.
{
"iisSettings"
: {
"windowsAuthentication"
:
false
,
"anonymousAuthentication"
:
true
,
"iisExpress"
: {
"sslPort"
: 44340
}
},
"profiles"
: {
"IIS Express"
: {
"commandName"
:
"IISExpress"
,
"launchBrowser"
:
true
,
"environmentVariables"
: {
"ASPNETCORE_ENVIRONMENT"
:
"Development"
,
"ASPNETCORE_HOSTINGSTARTUPASSEMBLIES"
:
"Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation"
}
},
"ASPNETCore5App"
: {
"commandName"
:
"Project"
,
"launchBrowser"
:
true
,
"environmentVariables"
: {
"ASPNETCORE_ENVIRONMENT"
:
"Development"
,
"ASPNETCORE_HOSTINGSTARTUPASSEMBLIES"
:
"Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation"
}
}
}
}
To disable this on the development environment, comment or remove the line 16 and 25.
While creating the project, if you forgot to check the “Enable Razor runtime compilation” option, then you can still enable it via adding the nuget package Microsoft.AspNetCore.Mvc.Razor.RuntimeCompilation
and then configuring in the Startup.cs
.
Keep visiting this blog for other interesting tutorial. Thank you
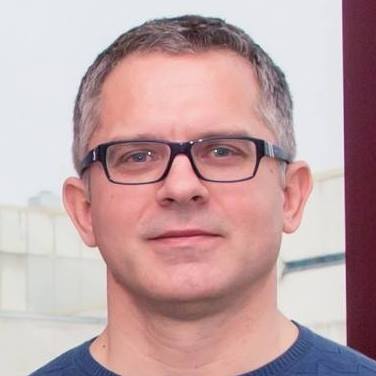
Javier is Content Specialist and also .NET developer. He writes helpful guides and articles, assist with other marketing and .NET community work