One of the most well-known programming languages and application development platforms is the Microsoft.NET Framework. Millions of developers use the C# and ASP.NET frameworks to create Windows client applications, XML Web services, distributed components, client-server applications, database applications, and other types of software. It comes as no surprise that the majority of application owners and developers prioritize.NET application performance when creating new applications.
There are a variety of causes for slow.NET applications. Inadequate memory sizing, GC pauses, code-level errors, excessive exception logging, high usage of synchronized blocks, IIS server bottlenecks, and other issues are some of these. In this blog, we’ll examine some of the most prevalent performance issues with.NET applications and offer solutions.
6 Cause Make Your ASP.NET Site Working Slowly
1. Exceptions and Logs One Too Many
Exceptions in.NET are not always bad. Just mistakes are bad. The majority of developers hold this view. And if exceptions are properly handled—that is, thrown, caught, and addressed—rather than ignored, it is true. The broth is ruined by too many cooks, with some exceptions. The code can become inefficient and impact application performance if there are too many unhandled exceptions. Hidden exceptions are a minefield, which is worse. They may slow down the loading of web pages if they are left unchecked.
The excessive logging of exceptions is another issue with.NET. Your debugging toolbox may include logging as a powerful tool to find anomalies noted during application processing. The same exception, however, might end up being logged at the web, service, and data tiers if logging is configured to catch exceptions at each level of the application architecture. The application code may experience an increase in workload as a result, slowing down response times. One must be careful to only log fatal events and errors in production environments. If you log everything, including debugs, warnings, and informational messages, your production log file will quickly become bloated, which will affect how the code is processed.
Few tips to fix it:
- To handle exceptions, make sure your C# code contains “try catch finally” blocks.
- Use exception filters, which are available in C# 6 and higher and allow you to specify a conditional clause for each catch block.
- To prevent potential exceptions, make sure there are no null values and use TryParse.
- Second-chance exceptions are important to pay attention to because they show that the first-chance exception occurred but wasn’t properly handled.
- To log exceptions to a file or a database, use libraries for exception handling and logging like Enterprise Library, NLog, Serilog, or log4net.
- Make sure to only log exceptions as much as is necessary to avoid overwriting the log file.
2. Using thread synchronization and locking excessively
The.NET Framework provides numerous thread synchronization options, including Reader/Writer locks, inter-process mutexes, and more. There will be times when a.NET developer will write the code so that only one thread can be serviced at a time, forcing the other parallel threads to wait in a queue. For instance, a checkout application should process items one request at a time in accordance with its business logic. The incoming threads are serialized for execution with the aid of synchronization and locking. An incoming thread must wait until the lock on the synchronized object is available when a synchronized block of code has been created and applied to a particular object. While this tactic is useful in some circumstances, it shouldn’t be employed too frequently. If threads are serialized excessively, incoming threads will have to wait longer, which will slow down user transactions.
Few tips to fix it:
- Use locks and synchronized coding only when necessary. Before choosing to use locks, be aware of the need for code execution.
- Set up locks to last for the shortest amount of time possible so that other threads are not kept waiting around for a long time after they are acquired.
- Use loose coupling to lessen concurrency-related problems. Lock contention can also be reduced by using event-delegation models.
- To determine whether thread locks are the cause of sluggish application processing, track the.NET code using code profiling tools.
3. Your Applications Hangs
It is one thing to deal with a slow URL in a specific case. But things can only get worse when the IIS website simply hangs and all or the majority of web pages take an eternity to load. Typically, a hang could happen when an application is overloaded or deadlocked. .NET applications typically experience two different types of application hang scenarios.
IIS issue (hard hang)
This typically takes place where the request is queued, at the start of the request processing pipeline. All of the available threads may become blocked due to an application deadlock, placing incoming requests in a queue while they wait to be handled. This may also occur if there are more open requests than the IIS Server’s configured concurrency limit. Such a hang would show up as timed-out requests and 503 Service Unavailable errors. All URLs and the entire web application itself are impacted by a hard hang. To fix this issue, please kindly read our previous post about how to fix 503 service unavailable errors.
ASP.NET issue (soft hang)
This typically occurs as a result of poor application code in a particular area, which only affects a small number of URLs and not the entire website. Typically, the ExecuteRequestHandler stage is where an ASP.NET controller or page hangs. You might want to fire up a debugger to see precisely where the request is stuck to confirm this. Verify the URL, stage name, and module name. The URL will show which controller or page is to blame for the hang.
How to fix this issue:
- Check the Http Service Request QueuesCurrentQueueSize counter in the Windows performance monitor to see if IIS is the cause of the problem. There is no request backed up in an IIS queue if it equals 0.
- If the problem isn’t with IIS, it must be at the controller or page level of the ASP.NET code.
- Use any code profiling to determine which URL(s) are hanging and to obtain a thorough request trace. To confirm that it is an ASP.NET problem, check the module name and stage name at which the request hangs.
- Code profiling with a transaction tracing tool can assist in pinpointing the precise line of code that has the issue.
4. Regular Pauses in Garbage Collection
When the memory used by the allocated objects on the managed heap exceeds the acceptable threshold set by the application developer, garbage collection (GC) is initiated in the.NET CLR. At this point, the GC.Collect method engages and frees the memory used by dead objects. In the CLR, GC typically takes place in the Generation 0 heap, which is where transient objects are kept. When GC occurs in a Generation 2 heap, which contains long-lived objects, it is referred to as a full GC. Every time GC occurs, it increases the CPU load on the CLR and slows down the processing of applications. Therefore, the application tends to experience slowdowns in the case of longer and more frequent GC pauses.
Tips to fix this issue:
- Make sure GC limits are set appropriately and that GC heap memory is appropriately sized.
- Avoid using large strings and objects where they are not required.
- Keep track of when GC occurs, how long it takes, and what percentage of that time is used by the JVM.
- Watch for the Full GC to occur. This might slow down the application.
- Depending on the requirements of the application, use server or workstation GC wisely.
- The CLR layer should be continuously monitored to spot memory usage, GC activity, CPU spikes, etc.
5. IIS Bottlenecks
The.NET Framework’s Microsoft IIS Server is an essential component. The.NET-built web application or website is hosted by IIS, which also runs the W3WP process, which is in charge of responding to incoming requests. The Common Language Runtime (CLR), which distributes resources for thread processing, is a component of IIS as well. IIS has many moving parts, so a bottleneck there could directly affect the performance of the.NET application.
6. Database Issue
Application performance issues aren’t always related to.NET code. One frequent cause is frequently queries that run slowly. However, the developers of.NET applications are frequently held accountable for poor application performance. This is because there is no contextual visibility of how the processing of.NET applications is impacted by SQL performance. Slow query processing could be caused by connectivity issues with ADO.NET and ODP.NET, but this is less common than poorly-formed queries. The database’s ability to process queries could also be impacted by poor execution plans, missing indexes, a poorly designed schema, small buffer pools, missing joins, poor caching, improperly pooled connections, etc.
While the DBAs are in charge of the database’s performance and query creation, the owner of the.NET application must look for query-level problems as the application is processed. By doing so, it will be easier to distinguish between database issues and code-level issues and spare the.NET developers the time-consuming task of searching for code problems.
Few tips to fix it:
- To identify slow queries, track query processing within the context of application transactions.
- To ensure consistent performance, properly plan database sizing and configuration.
- To find and fix missing indexes, improve the database layout by re-indexing, etc., use database monitoring tools.
- Utilize the application to monitor database connectivity and identify any connection problems.
- .NET code profiling will help catch.NET method-level issues, database query-level issues, and slow remote procedure calls, in addition to slowness caused by internal calls like HTTP, Web Service, and WCF.
Conclusion
The performance of your server can be negatively impacted by a variety of factors, and mistakes can occur for a variety of reasons. If you think about it, there are unfortunately no short cuts to creating a quick and dependable system. You’ll need thorough planning, qualified engineers, and lots of time to allow for unforeseen circumstances. Finding the issue and its root cause typically takes up 90% of the work. We hope above article can help to fix your ASP.NET performance issue.
Are you looking for an affordable and reliable Asp.net core hosting solution?
Get your ASP.NET hosting as low as $1.00/month with ASPHostPortal. Our fully featured hosting already includes
- Easy setup
- 24/7/365 technical support
- Top level speed and security
- Super cache server performance to increase your website speed
- Top 9 data centers across the world that you can choose.
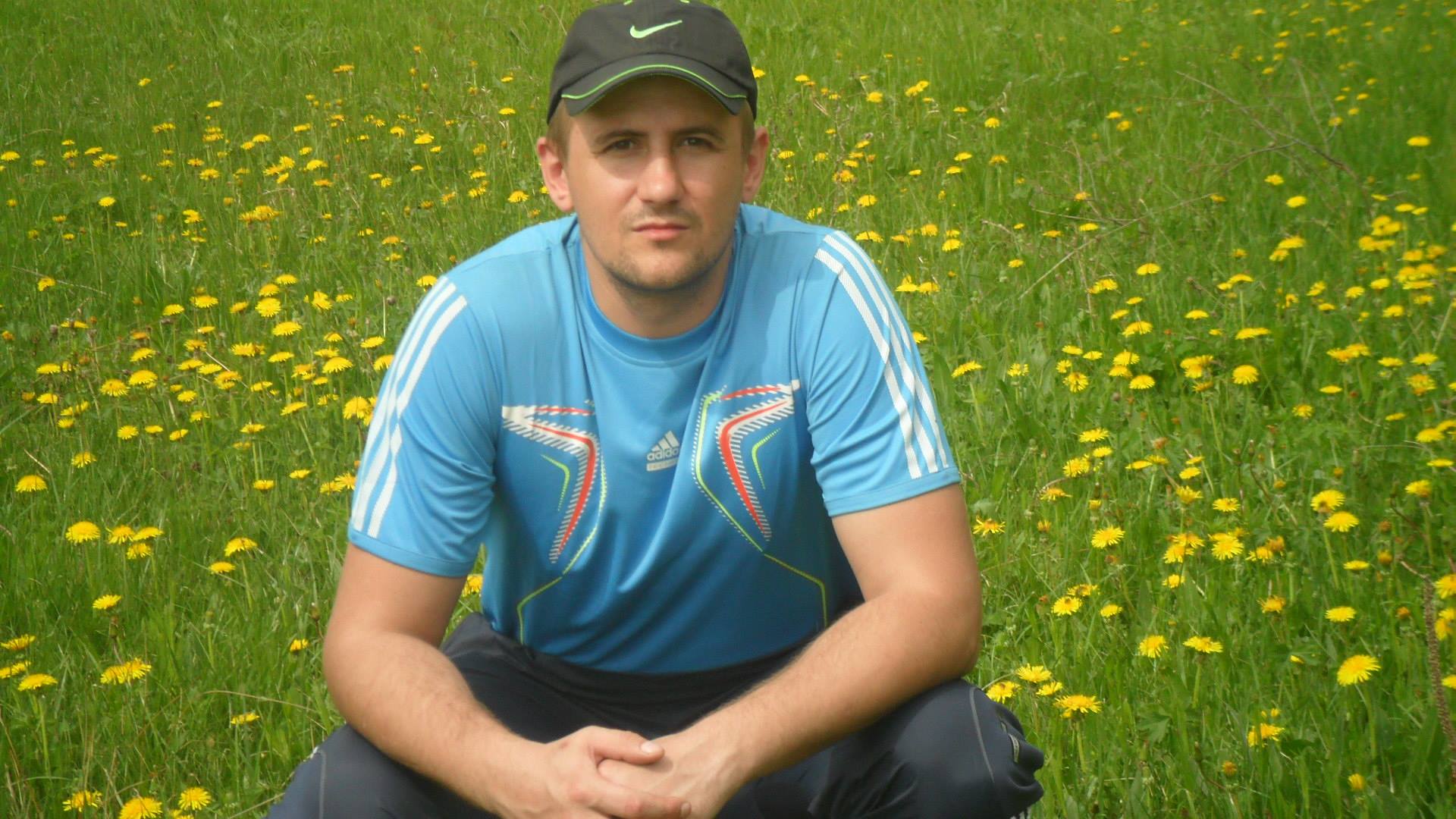
Yury Sobolev is Full Stack Software Developer by passion and profession working on Microsoft ASP.NET Core. Also he has hands-on experience on working with Angular, Backbone, React, ASP.NET Core Web API, Restful Web Services, WCF, SQL Server.