Two open-source tools for creating interactive single-page applications (SPAs) are Blazor and React. Facebook introduced React, a Javascript library, in 2013 to help developers create user-friendly interfaces and UI elements. On the other hand, Microsoft introduced Blazor, a UI framework, in 2018. In contrast to React, Blazor uses the syntax of C# and Razor rather than Javascript to build reusable and user-friendly user interfaces. Blazor has made a bit of a stir among developers because, prior to its creation, JavaScript served as the foundation for all significant frontend frameworks.
Nevertheless, despite the fact that both tools perform the same task, their suitability for various tasks varies. To help identify which projects are best suited for each, we’ll compare their features and consider the advantages and disadvantages of each in this post.
We’ve had a lot of inquiries about Blazor’s suitability for larger SaaS projects as an alternative to React, Vue, or Angular since we published this article. At the conclusion of the article, there is a special section dedicated to questions and answers. Please feel free to ask any more questions in the comments section, and we’ll answer them so that others can also benefit!
Blazor overview
Microsoft created the open-source Blazor web development framework. It lets you create web user interfaces (UIs) for client-side NET applications by utilizing C# in addition to HTML and CSS. Because Web Assembly is used for application execution, the programs can run on any browser. Because of this, you can operate the application without the need for any external plugins or add-ons, providing users with platform independence.
Blazor is a favorite tool among developers because it is compatible with Javascript. This enables the calling of Javascript functions from.NET methods and Javascript functions from.NET methods within a Blazor application. additionally uses C# as the codebase to unify the client and server-side, enabling developers to share libraries and create cutting-edge single-page applications.
Blazor features
Among the most notable Blazor characteristics are:
1. Offers two hosting models
Server-side Blazor and Blazor WebAssembly are the two main hosting models that the Blazor framework offers. You can host your application on ASP.NET Core, where “Razor components” are executed, using server-side Blazor. Razor components are separate interface elements with logic execution capabilities that allow for dynamic behavior. Through a SignalR connection, the frontend transmits event handling, Javascript functional calls, and UI updates.
Blazor server applications have quick load times and full.NET API compatibility. They have problems with scalability and high latency, though.
With the Blazor WebAssembly (WASM) model, your.NET application can run in a browser. In order to accomplish this, the application must first be downloaded to the client’s browser along with all of its dependencies, which include the.NET runtime, images, HTM, and CSS. Because of this, Browser WASM apps can operate without a server connection, which makes the host model perfect for hosting static websites. After the files are downloaded, Blazor WASM allows clients to run the application offline in addition to offloading processing to the browser.
2. Supports HTML and CSS
Blazor renders the display in standard HTML and CSS, even though it creates user interface components using Razor templates. As a result, you can style your page using any CSS framework and preprocessor. For teams like ours that have embraced Tailwind CSS, this is wonderful news.
Blazor also has CSS isolation capabilities, which let you style each component independently and avoid style conflicts. In addition, Blazor has built-in form and input components that facilitate the creation of HTML syntax for forms.
3. Pre-rendering
The majority of single-page applications load slowly because of the time it takes for the client’s browser to render the data after it has been fetched from servers. Server-side pre-rendering, provided by Blazor, ensures that all webpage elements are compiled on the server and that static HTML is sent to the client, providing a solution to this issue. This implies that the user interface is visible even before the program has finished downloading. Because of Blazor’s pre-rendering, SPAs load more quickly, which enhances both their SEO and user experience.
4. Virtualization
One way to restrict UI rendering to only the elements that the user can currently see is through virtualization. When working with large volumes of data, which can take some time to process and display, this idea is helpful. For instance, you can use virtualization to render only a portion of the items on the user’s viewport if your page has more than 2000 items. As the user scrolls the viewport, the remaining data will be loaded and rendered dynamically.
To increase performance, Blazor’s virtualization features can be applied to Blazor server-side apps or Blazor WASM applications. You must use the virtualize HTML tag to wrap the data in order to activate this feature. On the other hand, you would need to iterate through the items using the C# foreach loop if virtualization wasn’t present.
Without virtualization:
@foreach (var employee in employees)
{
<tr>
<td>@employee.EmployeeId</td>
<td>@employee.Name</td>
<td>@employee.Role</td>
</tr>
}
Implementing virtualization:
<Virtualize Items="employees" Context="employee">
<tr>
<td>@employee.EmployeeId</td>
<td>@employee.Name</td>
<td>@employee.Role</td>
</tr>
</Virtualize>
5. Lazy Loading
A design pattern called lazy loading enables an application to load particular files only when the user requests them. Lazy loading is supported by Blazor WASM, which accelerates your app’s startup. This means that the app doesn’t download all of the resources at startup; instead, it delays loading a resource until the user requests it.
6. gRPC-web support
An open-source remote procedure call framework called gRPC makes it easier for client-side and server-side apps to communicate effectively. Recently, the framework and a library were integrated into Blazor to facilitate the creation of a gRPC server client-side in ASP.NET and Blazor.
Blazor pros and cons
Pros
- Permits the execution of.NET applications in a web browser.
- SPAs can run in an offline state
- Because Blazor WASM and Javascript are compatible, developers can utilize both.NET methods and Javascript functions.
- Most browsers can run Blazor WASM applications without the need for extra plug-ins or source compilers.
Cons
- Extended first load times, particularly on devices using a shoddy connection. This is due to the fact that downloading the complete.NET runtime is necessary.
- It has.NET tools and restricted debugging capabilities.
- Not suitable for thin clients
Server-side Blazor advantages include:
- C# is used in the development of both server-side Blazor WASM applications. This facilitates quicker development and frees up developers to work on more crucial procedures.
- Provides a quick and effective build time.
- The app performs better because its component download sizes are substantially smaller than those of the Browser WASM.
- Operates on all browsers, even those that don’t support WebAssembly and thin clients
- Utilizes the complete.NET Core runtime
Cons
- Less capacity to scale. This is because event handling in server-side Blazor apps is done through SignalR connections. The number of events that can be managed from the client-side with this kind of connection is limited.
- It does not provide offline support because it requires constant connection to the server.
- Does not function well in environments with high latency
React overview
React is a library for creating UI components that runs on top of Javascript. Facebook created and released it in 2013, and since then, it has gained popularity as a UI library for creating incredibly user-friendly interfaces. Notable businesses like Paypal, DropBox, Twitter, Netflix, and Walmart use it.
By the time it was released, developers were using different frameworks like Vue and Angular, which frequently meant that most of the code needed to be redone. React positioned itself as the answer to this issue by facilitating the creation of reusable code components, which sped up the development process and simplified SPA maintenance.
React features
Some of the notable features of React include:
1. JSX
Javascript and HTML are combined in the Javascript Syntax Extension (JSX). It is used in React to write HTML and Javascript together more easily, which facilitates debugging and understanding of the code. In addition to being quicker than standard Javascript code, JSX contributes to the high performance of React apps. Here is some basic JSX code:
const name = ‘JSX sample’
const ele = <h1>This is a {name}</h1
It should be noted that JSX cannot be run directly in a browser since it is not legitimate Javascript code. To translate it into browser-friendly Javascript code, the Babel compiler must be used for compilation.
2. Virtual DOM
An essential component of UI web development is DOM manipulation. It enables developers to alter user interface elements and carry out user actions on web components. However, because it updates the entire DOM at once, this process is typically slower in most Javascript frameworks, which reduces web application performance. Most frameworks will rebuild the entire list in order to update the three items if a user checks off only three of the twenty items in an app.
React leverages virtual DOM, which is essentially a memory-based representation of the actual DOM, to increase performance. In this manner, whenever an element within the web application is changed, the virtual DOM is updated first, and any discrepancies between the elements are compared with the actual DOM. Then, ReactDOM updates only the modified portions of the application, leaving the rest unchanged. We refer to this procedure as reconciliation.
3. One Way Data Binding
Data flows from parent components to child components in a single direction when using React’s unidirectional data flow feature. Because of this, a child component’s properties cannot transmit data to its parent component, but they can still interact to change states in response to inputs. This model aids in keeping all app operations quick and modular.
4. Components
The user interface is divided into multiple components by React. These components are simple to debug because they each have a unique set of attributes and capabilities. Reusing the components also aids in accelerating the development process. Additional attributes of React components consist of:
- Nesting is the ability of a component to hold multiple smaller components inside of it.
- You can specify the rendering style of a particular UI component in the DOM by using the render method.
- Allowing a component to inherit properties from its parent component is known as passing properties.
5. Extenstion Support
Many extensions are supported by React, which facilitates the development of complete user interface applications. They also make it possible for server-side rendering and mobile app development with React. Redux, React Native, and Flux are a few of the extensions.
React pros and cons
Pros
- Increases app performance because of the virtual DOM feature.
- Makes use of reusable components to reduce development time
- Uses less and writes easily understood and debugged code that is maintainable
- Improves SEO due to improved performance
- Has a large selection of development tools
- Supports Next.js, our preferred front-end framework
- The library receives frequent updates to provide additional functionality.
- React boasts a robust user community that offers solutions and resources for optimizing the library’s use.
Cons
- Inadequate documentation, particularly concerning recent updates and releases
- Developers must continuously invest in learning about new React developments because of its rapid updates.
Comparing Blazor and React for building SPAs
An alternative to using Javascript for SPAs is the relatively new UI framework called Blazor. Its main language for creating interactive web applications is C#. Thus, Blazor is the best option for companies that already employ a team of.NET developers. The process of hiring Javascript developers to create SPAs is lessened because the current developers can create reusable front- and back-end code with ease.
React is a popular Javascript-based user interface library with a large number of third-party packages, extensions, and libraries. These resources aid in optimizing React apps and streamlining the development process. Moreover, React is a superior option than Blazor since it aids in the development of high-performance SPAs.
FAQ
– Is Blazor faster than React?
No. Blazor apps typically load more slowly than React apps, especially when compared to Blazor WASM, which needs to download additional app dependencies in addition to the full.NET runtime. Nevertheless, Blazor server-side applications outperform React apps in terms of speed.
– JavaScript is unfamiliar to the experts on my team, who specialize in.net. Is Blazor the best option available to us?
Both yes and no. Yes, Blazor is the best option if your team is adamant about not using JavaScript or hiring a seasoned agency to develop your frontend. But only because it’s the best non-JS option, not because it’s superior to JavaScript frameworks. Blazor is far behind React, Vue, and Angular in terms of maturity, resources, and tooling.
Blazor may be picked up by your team quickly, but in the end, you’re giving up the opportunity to switch to a better stack.
Blazor is incompatible with a large number of excellent libraries, packages, and tools that are designed to be used with JavaScript frameworks. Imagine finding a library that could accomplish a complicated requirement in 15 minutes of implementation, only to discover that your tech stack makes it necessary for you to build the same feature over the course of a whole week.
– Is Blazor developed enough for use in enterprise applications?
It truly depends on how intricate your application is. When compared to other well-known JavaScript frameworks, Blazor is still in its infancy.
Nothing would stop Blazor from developing an enterprise application, particularly when combined with an asp.net core microservice architecture REST API.
But, Blazor is not as developed as the well-known JavaScript frameworks, as we mentioned in the previous response, so there will undoubtedly be occasions when you wish you were using the same stack as everyone else.
– Is Blazor replacing Javascript?
No. Building SPAs is a common use for Javascript, which has a thriving ecosystem of development tools. Its libraries and frameworks—React in particular—are well-known and have a track record of producing reliable applications. However, Blazor hasn’t advanced past the experimental stage or gained the support of Javascript developers.
Conclusion
Overall, you need to weigh the trade-offs associated with each tool when choosing between Blazor and React. Most importantly, it all depends on your team: while React is ideal for Javascript developers, Blazor is best suited for teams of.NET developers.
Whether you choose Blazor or React, we (ASPHostPortal) have support this both features on our hosting environment. We provide everything you need, from developer-made tools like Git integration and access managers for user management to basic features like a free domain name, unlimited bandwidth, and free SSL.
Make the switch to ASPHostPortal right now to see the difference for yourself.
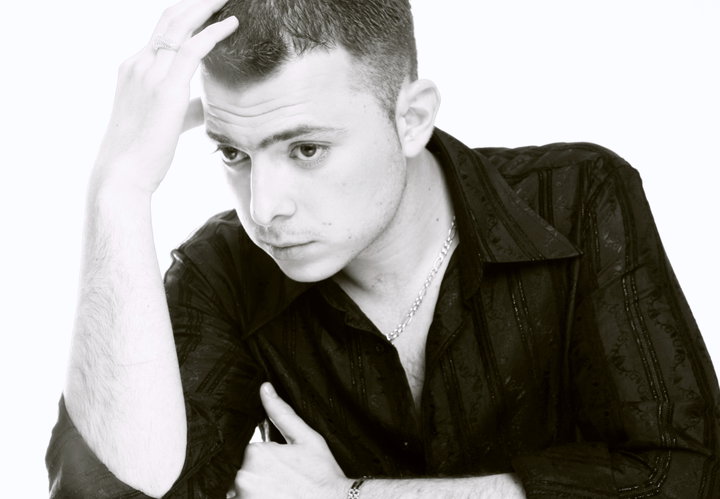
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.