Introduction
Databases are the backbone of modern applications. Whether you’re building a mobile app, a website, or enterprise software, choosing the right database is crucial. Two popular options are SQLite and Microsoft SQL Server, but they serve very different purposes.
This guide will explain:
- What SQLite and SQL Server are
- Key differences between them
- When to use each one
- Step-by-step implementation examples
- Performance and security considerations
- Real-world use cases
By the end, you’ll know exactly which database to choose for your project.
What is SQLite?
SQLite is a lightweight, file-based database that doesn’t require a separate server. It stores all data in a single file on disk.
Key Features
✅ No server needed – Runs inside your application
✅ Zero configuration – Just create a file and start using it
✅ Cross-platform – Works on Windows, Linux, macOS, Android, iOS
✅ ACID-compliant – Ensures data reliability
✅ Small footprint – The entire library is less than 1MB
Example Use Cases
- Mobile apps (WhatsApp, Android contacts)
- Desktop applications (Firefox, Skype)
- Embedded systems (car systems, IoT devices)
- Temporary datasets for testing
SQLite Code Example (Python)
Pros and Cons
Pros | Cons |
---|---|
Easy to set up | No user management |
Fast for small datasets | Poor concurrency (only 1 writer at a time) |
Portable (single file) | Limited scalability |
No server maintenance | No network access |
What is SQL Server?
Microsoft SQL Server is a full-featured enterprise database designed for large-scale applications.
Key Features
✅ Client-server architecture – Dedicated database server
✅ High performance – Handles millions of transactions
✅ Advanced security – Encryption, user roles, auditing
✅ Scalability – Supports clustering and replication
✅ Business intelligence tools – Built-in reporting and analytics
Example Use Cases
- Banking systems
- E-commerce websites
- Hospital record systems
- Large corporate applications
SQL Server Code Example (C#)
using System; using System.Data.SqlClient; class Program { static void Main() { string connectionString = "Server=localhost;Database=MyApp;User Id=sa;Password=your_password;"; using (SqlConnection conn = new SqlConnection(connectionString)) { conn.Open(); // Create table string createTable = @"CREATE TABLE Users ( Id INT PRIMARY KEY IDENTITY, Name NVARCHAR(100), Email NVARCHAR(100))"; new SqlCommand(createTable, conn).ExecuteNonQuery(); // Insert data string insertData = "INSERT INTO Users (Name, Email) VALUES (@Name, @Email)"; SqlCommand cmd = new SqlCommand(insertData, conn); cmd.Parameters.AddWithValue("@Name", "John"); cmd.Parameters.AddWithValue("@Email", "[email protected]"); cmd.ExecuteNonQuery(); // Query data SqlDataReader reader = new SqlCommand("SELECT * FROM Users", conn).ExecuteReader(); while (reader.Read()) { Console.WriteLine($"{reader["Name"]} - {reader["Email"]}"); } } } }
Pros and Cons
Pros | Cons |
---|---|
Handles high traffic | Complex setup |
Excellent security | Expensive licensing |
Advanced features | Resource intensive |
Good for teams | Requires maintenance |
Main Differences Between SQLLite and SQL Server
Aspect | SQLite | SQL Server |
---|---|---|
Type | Embedded, serverless RDBMS | Full-fledged client-server RDBMS |
Installation | No installation; just a DLL or NuGet package | Requires installation and configuration (standalone or cloud-based) |
File Storage | Single .db or .sqlite file on disk |
Data stored in .mdf and .ldf files managed by SQL Server engine |
Deployment Target | Mobile, desktop, IoT, embedded systems | Enterprise apps, web apps, cloud, and high-traffic systems |
Concurrency Support | Single-writer, multiple-readers (file-level locking) | High concurrency with row-level locking and isolation levels |
Multi-User Support | Not designed for multi-user access | Fully supports multi-user, concurrent connections |
Performance (Write-heavy) | Limited (due to locking) | Optimized for high-throughput write workloads |
Transaction Support | ACID-compliant with limited isolation levels | Full ACID compliance with multiple isolation levels (Read Committed, etc.) |
Data Typing | Dynamic typing (type affinity model) | Strong static typing; schema enforces column data types strictly |
Stored Procedures | Not supported | Fully supported |
Triggers & Views | Basic triggers and views supported | Advanced triggers, views, indexed views, and INSTEAD OF triggers |
Indexing | Supports basic indexing | Advanced indexing: full-text, spatial, filtered, columnstore, etc. |
Authentication & Security | No built-in user management | Integrated security, Active Directory, roles, permissions |
Tools | SQLite CLI, DB Browser for SQLite, DBeaver | SQL Server Management Studio (SSMS), Azure Data Studio |
Backup & Restore | Manual file copy or backup APIs | Full backup/restore support, point-in-time recovery, differential backups |
Replication | Not supported | Multiple replication strategies: transactional, merge, snapshot |
Cloud Integration | Not natively supported | Fully integrated with Azure SQL, AWS RDS, etc. |
File Size Limit | ~281 TB theoretically (practical limits much smaller) | 524 PB (per database); practically limited by edition and disk space |
License | Public domain (free for commercial and personal use) | Free (Express), paid editions (Standard, Enterprise) with licensing models |
Cross-platform Support | Windows, Linux, macOS, Android, iOS | Windows-native; Linux and container support available (2017+) |
Language Support | SQL-92 subset | T-SQL (Transact-SQL), richer procedural extensions |
Use Case Suitability | Single-user apps, prototyping, testing, lightweight data stores | Multi-user apps, enterprise-grade applications, data warehousing |
When to Use SQLite or SQL Server
SQLite Best For:
-
Lightweight, single-user applications
-
Offline-first mobile or desktop apps
-
Rapid prototyping and development
-
Embedded systems and IoT devices
-
Testing environments with minimal overhead
Use SQLite When:
Scenario | Why SQLite is Suitable |
---|---|
Mobile Apps (Android/iOS) | Minimal overhead, no server dependency, local storage via a .db file. |
Desktop Applications | Easy deployment—just ship the .db file with the app. |
IoT or Edge Devices | Low-resource environments; no need for a separate DB server. |
Unit Tests / CI Pipelines | In-memory DB mode is fast and isolated for testing logic. |
Small Internal Tools | No need for user access control or enterprise features. |
Offline-First Apps | App functions without internet; DB resides locally. |
Prototyping / PoC | Spin up a quick app with persistent local data without complex setup. |
SQLite May Not Be Suitable When:
-
You expect many concurrent users or high transaction throughput.
-
You need advanced features like stored procedures, role-based security, or data replication.
-
You’re dealing with high-volume writes, large datasets, or complex queries.
SQL Server Best For:
-
Enterprise applications
-
Web APIs or microservices requiring centralized data
-
High-concurrency or multi-user systems
-
Data warehousing, analytics, reporting
-
Secure and scalable business applications
Use SQL Server When:
Scenario | Why SQL Server is Suitable |
---|---|
Enterprise Web Applications | Robust support for transactions, concurrency, and performance tuning. |
Multi-user Business Apps | Built-in security, access control, and concurrent access. |
APIs/Microservices | Centralized storage for data consistency across services. |
Business Intelligence (BI) & Reporting | Advanced indexing, stored procs, and integration with SSIS, SSRS, Power BI. |
Cloud-Based Apps (Azure SQL) | Fully managed options in the cloud, with high availability and scaling. |
Auditing & Compliance | SQL Server supports audit logs, encryption, and fine-grained permissions. |
Data Warehousing & ETL Pipelines | Strong integration with ETL tools and support for massive datasets. |
SQL Server May Be Overkill When:
-
You’re building a small-scale app with just a few users.
-
You want zero configuration or deployment complexity.
-
You’re targeting offline or embedded environments.
Conclusion
SQLite and SQL Server serve different purposes:
- SQLite = Simple, embedded, single-user
- SQL Server = Powerful, scalable, multi-user
Choose based on your project needs. For small apps, SQLite is often perfect. For business applications, SQL Server is worth the investment.
Looking for a powerful, reliable, and fully managed SQL Server hosting solution? Look no further! ASPHostPortal offers premium SQL Server hosting with high performance, security, and scalability—perfect for your .NET applications, enterprise software, and data-driven websites.
Get Started Today!
💡 Choose from flexible hosting plans—from shared SQL Server hosting to dedicated cloud databases.
🔥 Special Offer: Get 15% OFF your first SQL Server hosting plan!
🔗 Visit ASPHostPortal Now!
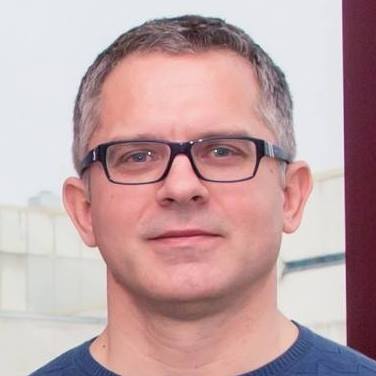
Javier is Content Specialist and also .NET developer. He writes helpful guides and articles, assist with other marketing and .NET community work