In previous posts, we have written an article about how to use Gmail to send emails in ASP.NET Core. And there are others popular email library called “Mailkit“. Because it is open source, incredibly adaptable, and based on.NET Standard, you may reuse the same code in.NET Full Framework, UWP, and.NET Core projects.
OK, here we go!!
Create An Email Service
Building an abstraction on top of a new library when you integrate it is always a good idea. What if a superior emailing library were to replace MailKit in the future, using it as an example? Will every reference in our code need to be updated in order to reference this new library? Or perhaps MailKit needs to alter something that breaks between versions; in that case, will we need to go through our code and repair every change that is now broken?
Creating an abstraction also gives us the opportunity to map out the appearance of our service before worrying about implementation specifics. Without having to concern ourselves with the specifics of how MailKit functions, we can take a very high level view of, say, sending an email. I won’t spend too much time explaining at this point; instead, we will simply proceed through the code because there is a lot to get through. Move along!
Let’s start by building an EmailAddress class. There will only be two properties in this that provide an EmailAddress.
public class EmailAddress { public string Name { get; set; } public string Address { get; set; } }
We now require a basic means of describing an email message. There are a ton of characteristics on an email, such as headers, attachments, CC, and BCC, but for now, we’ll stick to the fundamentals. All of this being contained within a class allows us to add more characteristics as we require them in the future.
public class EmailMessage { public EmailMessage() { ToAddresses = new List<EmailAddress>(); FromAddresses = new List<EmailAddress>(); } public List<EmailAddress> ToAddresses { get; set; } public List<EmailAddress> FromAddresses { get; set; } public string Subject { get; set; } public string Content { get; set; } }
We must now configure our email system. Our SMTP servers, ports, credentials, etc. are listed there. To hold everything, we will create a straightforward settings class for this. We shall employ an interface as well because we are skilled programmers.
public interface IEmailConfiguration { string SmtpServer { get; } int SmtpPort { get; } string SmtpUsername { get; set; } string SmtpPassword { get; set; } string PopServer { get; } int PopPort { get; } string PopUsername { get; } string PopPassword { get; } } public class EmailConfiguration : IEmailConfiguration { public string SmtpServer { get; set; } public int SmtpPort { get; set; } public string SmtpUsername { get; set; } public string SmtpPassword { get; set; } public string PopServer { get; set; } public int PopPort { get; set; } public string PopUsername { get; set; } public string PopPassword { get; set; } }
We now need to actually load this settings into our application. You must include a section for email settings at the base of your appsettings.json file. It should resemble the following:
{ "EmailConfiguration": { "SmtpServer": "smtp.myserver.com", "SmtpPort": 465, "SmtpUsername": "smtpusername", "SmtpPassword": "smtppassword", "PopServer": "popserver", "PopPort": 995, "PopUsername": "popusername", "PopPassword" : "poppassword" } ....Other settings here... }
In the ConfigureServices method or your startup.cs, with just one line, we can extract this configuration and import it into our program.
public void ConfigureServices(IServiceCollection services) { services.AddMvc(); services.AddSingleton<IEmailConfiguration>(Configuration.GetSection("EmailConfiguration").Get<EmailConfiguration>()); }
Injecting our configuration class everywhere in our program is made possible by this.
A straightforward email service that can be used to send and receive email is the last piece of the puzzle. Let’s start by developing an empty implementation and interface. Our settings object should be accepted as a constructor by the implementation.
public interface IEmailService { void Send(EmailMessage emailMessage); List<EmailMessage> ReceiveEmail(int maxCount = 10); } public class EmailService : IEmailService { private readonly IEmailConfiguration _emailConfiguration; public EmailService(IEmailConfiguration emailConfiguration) { _emailConfiguration = emailConfiguration; } public List<EmailMessage> ReceiveEmail(int maxCount = 10) { throw new NotImplementedException(); } public void Send(EmailMessage emailMessage) { throw new NotImplementedException(); } }
Return back to our ConfigureServices method of our startup.cs to add in a final line to inject in our EmailService everywhere.
public void ConfigureServices(IServiceCollection services) { services.AddMvc(); services.AddSingleton<IEmailConfiguration>(Configuration.GetSection("EmailConfiguration").Get<EmailConfiguration>()); services.AddTransient<IEmailService, EmailService>(); }
Congratz! We’re done now. If at this time we decide that MailKit is not for us, we still have an email service that can switch in and out libraries as needed, and our calling application doesn’t have to be concerned with what’s happening behind the scenes. The beauty of abstracting a library is just that!
Getting Started with Mailkit
Installing a Nuget package is all that is required to get MailKit up and running. Run the following command straightaway from your Package Management Console:
Install-Package MailKit
Now, you have access to Mailkit in your application.
How to Send Email via SMTP with Mailkit
Returning to our email service class, let’s fill out the “Send” method with the real MailKit email sending code. The necessary code is listed below:
public void Send(EmailMessage emailMessage) { var message = new MimeMessage(); message.To.AddRange(emailMessage.ToAddresses.Select(x => new MailboxAddress(x.Name, x.Address))); message.From.AddRange(emailMessage.FromAddresses.Select(x => new MailboxAddress(x.Name, x.Address))); message.Subject = emailMessage.Subject; //We will say we are sending HTML. But there are options for plaintext etc. message.Body = new TextPart(TextFormat.Html) { Text = emailMessage.Content }; //Be careful that the SmtpClient class is the one from Mailkit not the framework! using (var emailClient = new SmtpClient()) { //The last parameter here is to use SSL (Which you should!) emailClient.Connect(_emailConfiguration.SmtpServer, _emailConfiguration.SmtpPort, true); //Remove any OAuth functionality as we won't be using it. emailClient.AuthenticationMechanisms.Remove("XOAUTH2"); emailClient.Authenticate(_emailConfiguration.SmtpUsername, _emailConfiguration.SmtpPassword); emailClient.Send(message); emailClient.Disconnect(true); } }
The comments ought to be very self-explanatory, but let’s go over it quickly.
- You can send clear text or HTML emails depending on the “TextFormat” you use when creating your message body
- The framework class and MailKit’s Smtp class both go by the name “SmtpClient.” If you use Resharper or similar software, take care to click “Add Reference” on the right reference.
- While establishing a connection to the SMTP Server, you ought to always opt to use SSL.
Because we created our EmailService, EmailMessage, and EmailConfiguration classes earlier, they are all ready to use right away!
How to Receive Emails via POP with Mailkit
And now the code to receive email via POP.
public List<EmailMessage> ReceiveEmail(int maxCount = 10) { using (var emailClient = new Pop3Client()) { emailClient.Connect(_emailConfiguration.PopServer, _emailConfiguration.PopPort, true); emailClient.AuthenticationMechanisms.Remove("XOAUTH2"); emailClient.Authenticate(_emailConfiguration.PopUsername, _emailConfiguration.PopPassword); List<EmailMessage> emails = new List<EmailMessage>(); for(int i=0; i < emailClient.Count && i < maxCount; i++) { var message = emailClient.GetMessage(i); var emailMessage = new EmailMessage { Content = !string.IsNullOrEmpty(message.HtmlBody) ? message.HtmlBody : message.TextBody, Subject = message.Subject }; emailMessage.ToAddresses.AddRange(message.To.Select(x => (MailboxAddress)x).Select(x => new EmailAddress { Address = x.Address, Name = x.Name })); emailMessage.FromAddresses.AddRange(message.From.Select(x => (MailboxAddress)x).Select(x => new EmailAddress { Address = x.Address, Name = x.Name })); emails.Add(emailMessage); } return emails; } }
Again, everything is pretty straightforward.
While we only retrieve a few basic details about the email message, the actual MailKit email object contains a wealth of information that you can inspect, such as headers, CC addresses, and so on. Extend as needed!
Other FREE SMTP Server that You Can Use
It’s worth noting if you’re a hobbyist with your own website and want to send a few emails under your own domain every now and then. MailGun is a fantastic solution that I use for this blog. It has a great free plan that will be more than enough for most people, but it also has paid plans for when you really need to start sending a lot of email.
Summary
Now, you have learned how to send and receive emails using Mailkit in your ASP.NET Core application. We also give other emails option that you can use like Mailgun or you can also use Gmail as we have written previously on our blog.
Instead of hosting services, we also provide business email and also marketing email that can help you to grow bigger. Get your ASP.NET hosting as low as $1.00/month with ASPHostPortal. Our fully featured hosting already includes
- Easy setup
- 24/7/365 technical support
- Top level speed and security
- Super cache server performance to increase your website speed
- Top 9 data centers across the world that you can choose.
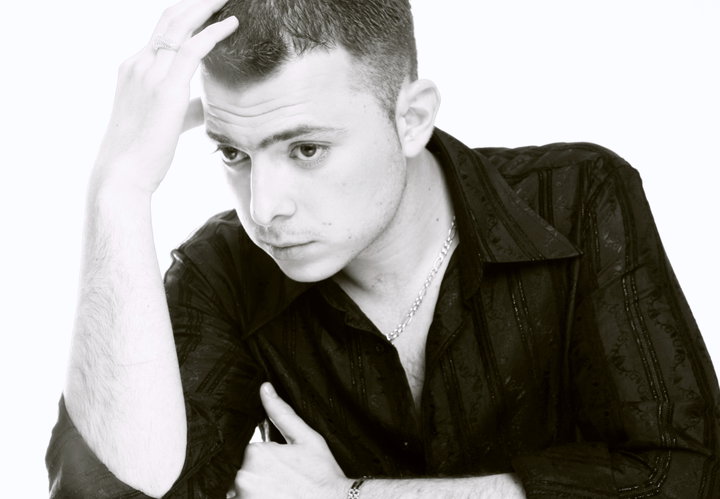
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.