There are a few options available if you want to use captcha code to shield your website from spam messages, including captcha.com and Google ReCaptcha. They can both be incorporated into ASP.NET Core programs. However, for some reason, such as the possibility that mainland China will access your website, you might still want to generate the captcha code yourself. In the most recent release of ASP.NET Core, this post will demonstrate how to create and use captcha code.
How to Create Captcha in Your ASP.NET Core Website
As shown below, we are going to create a new application called WebCaptcha for the demo.
The Project Name WebCaptcha and Location will be set next. We will make a decision in the final section.We won’t enable docker settings for this project, but Net Core framework and ASP.NET Core Version 3.1 will serve as the application’s framework. Other advanced settings include configuring https and enabling docker.
Now finally click on create button to create a project.
Project Structure
The configuration led to the creation of the project structure.
Next, after project creation, we’ll install DNTCaptcha.Core package obtained from NuGet.
After installing next, we are going to add TagHelper in _ViewImports.cshtml file.
Adding DNTCaptcha and @addTagHelper *.The _ViewImports.cshtml file’s Core directive makes DNTCaptcha.Core Helpers accessible to the View.
_ViewImports.cshtml file is located in Views folder.
@using WebCaptcha @using WebCaptcha.Models @addTagHelper *, Microsoft.AspNetCore.Mvc.TagHelpers @addTagHelper *, DNTCaptcha.Core
Following the addition of DNTCaptcha.Core Tag Helpers, we will add Model for Login to the Models folder in order to display the captcha demo.
Next, We’re going to add a model called LoginViewModel to the Model folder.
using System.ComponentModel.DataAnnotations; namespace WebCaptcha.Models { public class LoginViewModel { [Required(ErrorMessage = "Username Required")] public string Username { get; set; } [Required(ErrorMessage = "Password Required")] public string Password { get; set; } } }
After adding LoginViewModel Next, we will add a controller called PortalController and a Login Action method.
using Microsoft.AspNetCore.Mvc; using WebCaptcha.Models; namespace WebCaptcha.Controllers { public class PortalController : Controller { [HttpGet] public IActionResult Login() { return View(); } [HttpPost] [ValidateAntiForgeryToken] public IActionResult Login(LoginViewModel loginViewModel) { return View(); } } }
After adding Controller and Action Method next, we are going to register DNTCaptcha.Core Captcha Service in Startup class.
We have added the AddDNTCaptcha Service here, and you can configure it using a variety of storage providers, including
- SessionStorageProvider
- MemoryCacheStorageProvider
- CookieStorageProvider
- DistributedCacheStorageProvider
In addition, we can configure other options like Separators and Expiration.
For this demo, we are going to use CookieStorageProvider.
public void ConfigureServices(IServiceCollection services) { services.AddControllersWithViews(); services.AddDNTCaptcha(options => options.UseCookieStorageProvider() .ShowThousandsSeparators(false) ); }
After registering the service, we will add a view and use the DNTCaptcha.Core Tag Helper on that view.
Before adding Captcha on Login View let’s have a look at what different options, we can configure in it.
<dnt-captcha asp-captcha-generator-max="999999" asp-captcha-generator-min="111111" asp-captcha-generator-language="English" asp-captcha-generator-display-mode="ShowDigits" asp-use-relative-urls="true" asp-placeholder="Enter Security code" asp-validation-error-message="Please enter the security code." asp-font-name="Tahoma" asp-font-size="20" asp-fore-color="#333333" asp-back-color="#ccc" asp-text-box-class="text-box form-control" asp-text-box-template="<span class='input-group-prepend'><span class='form-group-text'></span></span>{0}" asp-validation-message-class="text-danger" asp-refresh-button-class="fas fa-redo btn-sm" asp-use-noise="false" />
Generator-language
If the captcha generator is used, we can set the maximum and minimum numbers. It also supports 5 different languages.
- English
- Persian
- Norwegian
- Italian
- Turkish
Display Mode
There are 4 different Display Mode.
- NumberToWord
- ShowDigits
- SumOfTwoNumbers
- SumOfTwoNumbersToWords
Placeholder and Error Message
We can Configure Custom Placeholder and Error Message.
Also, we can Customize various other options such as Font, Colour, Text box class, Error Message class.
Important Thing Required
- For Refresh button you can configure various class such as font awesome, glyphicon any one of this reference should be added then you can see refresh button rendering
- E.g.: – asp-refresh-button-class=”fas fa-redo btn-sm”
- Adding Validation Library Reference for Making Captcha Validate
- jquery-validation
- jquery-validation-unobtrusive
- Adding jquery.unobtrusive-ajax library
Due to DNTCaptcha, we must add the jquery.unobtrusive-ajax library in order for the refresh button on the Captcha to function.For the refresh button, the core uses jquery.unobtrusive-ajax, which you can download from the link below, add to your project, and reference.
Link to Download:- https://github.com/aspnet/jquery-ajax-unobtrusive/releases
Code Snippet of Login View
@model LoginViewModel @{ Layout = null; } <link rel="stylesheet" href="~/lib/bootstrap/dist/css/bootstrap.min.css" /> <link rel="stylesheet" href="~/css/site.css" /> <link href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.13.0/css/all.min.css" rel="stylesheet"> <div class="container"> <br /> <div class="col-md-4"> <div class="card"> <div class="card-body"> <div asp-validation-summary="ModelOnly" class="text-danger"></div> <form id="account" asp-controller="Portal" asp-antiforgery="true" asp-action="Login" method="post"> <span asp-validation-for="Username" class="text-danger"></span> <div class="input-group mb-3"> <input asp-for="Username" class="form-control" placeholder="UserName" /> </div> <span asp-validation-for="Password" class="text-danger"></span> <div class="input-group mb-3"> <input type="password" asp-for="Password" autocomplete="new-password" class="form-control" placeholder="Password" /> </div> <div class="input-group mb-3"> <dnt-captcha asp-captcha-generator-max="999999" asp-captcha-generator-min="111111" asp-captcha-generator-language="English" asp-captcha-generator-display-mode="ShowDigits" asp-use-relative-urls="true" asp-placeholder="Enter Captcha" asp-validation-error-message="Please enter the security code." asp-font-name="Tahoma" asp-font-size="20" asp-fore-color="#333333" asp-back-color="#ccc" asp-text-box-class="text-box form-control" asp-text-box-template="<span class='input-group-prepend'><span class='form-group-text'></span></span>{0}" asp-validation-message-class="text-danger" asp-refresh-button-class="fas fa-redo btn-sm" asp-use-noise="false" /> </div> <div class="row"> <div class="col-8"> </div> <div class="col-4"> <button type="submit" id="btnsubmit" class="btn btn-primary btn-block">Sign In</button> </div> </div> </form> </div> </div> </div> </div> <script src="~/lib/jquery/dist/jquery.min.js"></script> <script src="~/lib/bootstrap/dist/js/bootstrap.bundle.min.js"></script> <script src="~/js/site.js" asp-append-version="true"></script> <script src="~/lib/jquery-validation/dist/jquery.validate.min.js"></script> <script src="~/lib/jquery-validation-unobtrusive/jquery.validate.unobtrusive.min.js"></script> <script src="~/js/jquery.unobtrusive-ajax.js"></script>
Output
Validate Captcha on Post Request
To validate Captcha on Post DNTCaptcha has 2 method
- ValidateDNTCaptcha attribute
- IDNTCaptchaValidatorService
- ValidateDNTCaptcha
We only need to add the ValidateDNTCaptcha attribute to the Post Action method, and we can also configure other options like ErrorMessage, CaptchaGeneratorLanguage, and CaptchaGeneratorDisplayMode.
using DNTCaptcha.Core; using DNTCaptcha.Core.Providers; using Microsoft.AspNetCore.Mvc; using WebCaptcha.Models; namespace WebCaptcha.Controllers { public class PortalController : Controller { [HttpGet] public IActionResult Login() { return View(); } [HttpPost] [ValidateAntiForgeryToken] [ValidateDNTCaptcha( ErrorMessage = "Please Enter Valid Captcha", CaptchaGeneratorLanguage = Language.English, CaptchaGeneratorDisplayMode = DisplayMode.ShowDigits)] public IActionResult Login(LoginViewModel loginViewModel) { if (ModelState.IsValid) { } return View(); } } }
- IDNTCaptchaValidatorService
We can validate Captcha using the HasRequestValidCaptchaEntry method of the IDNTCaptchaValidatorService. Here, we need to manually add the error to the ModelState.
using System; using DNTCaptcha.Core; using DNTCaptcha.Core.Providers; using Microsoft.AspNetCore.Mvc; using WebCaptcha.Models; namespace WebCaptcha.Controllers { public class PortalController : Controller { private readonly IDNTCaptchaValidatorService _validatorService; public PortalController(IDNTCaptchaValidatorService validatorService) { _validatorService = validatorService; } [HttpGet] public IActionResult Login() { return View(); } [HttpPost] [ValidateAntiForgeryToken] public IActionResult Login(LoginViewModel loginViewModel) { if (ModelState.IsValid) { if (!_validatorService.HasRequestValidCaptchaEntry(Language.English, DisplayMode.ShowDigits)) { this.ModelState.AddModelError(DNTCaptchaTagHelper.CaptchaInputName, "Please Enter Valid Captcha."); return View("Login"); } } return View(); } } }
Different Methods that You Can Try
1. NumbertoWord
2. SumOfTwoNumbersToWords
3. ShowDigits
4. SumOfTwoNumbers
Conclusion
In above article, we know how to use CAPTCHA in ASP.NET Core application. It is very simple and straight forward to implement in ASP.NET Core applications. If you have any questions, please feel free to comment below.
Are you looking for an affordable and reliable Asp.net core hosting solution?
Get your ASP.NET hosting as low as $1.00/month with ASPHostPortal. Our fully featured hosting already includes
- Easy setup
- 24/7/365 technical support
- Top level speed and security
- Super cache server performance to increase your website speed
- Top 9 data centers across the world that you can choose.
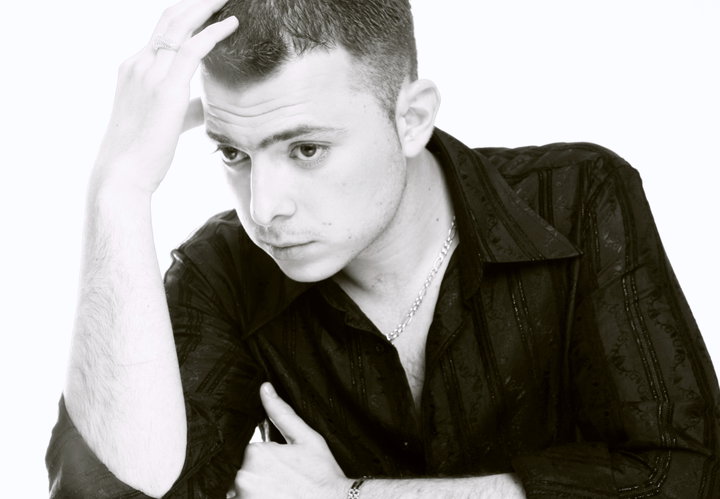
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.