Securing a Node.js API and its associated MSSQL (Microsoft SQL Server) database is critical to protecting sensitive information, ensuring data integrity, and minimizing vulnerabilities. This comprehensive guide covers essential techniques for strengthening your Node.js API and MSSQL database.
1. Use Environment Variables to Protect Sensitive Information
Storing sensitive data like API keys, database credentials, and other secrets directly in code is risky. Use environment variables to securely store these values and load them into your application at runtime.
– How to Use Environment Variables: Create a .env
file at the root of your project and include key-value pairs for your sensitive data. Use the dotenv
package in Node.js to access them securely.
// Load environment variables require('dotenv').config(); const dbUser = process.env.DB_USER; const dbPassword = process.env.DB_PASSWORD;
– Security Best Practices: Keep your .env
file out of version control (e.g., add .env
to .gitignore
). Additionally, consider using services like AWS Secrets Manager or Azure Key Vault for more robust secret management.
2. Use HTTPS for Secure Communication
Secure your Node.js API by enabling HTTPS. HTTPS encrypts the data transmitted between the client and server, protecting sensitive data from interception.
Setting Up HTTPS in Node.js: You’ll need an SSL/TLS certificate. Here’s a simple setup example:
CREATE DATABASE ENCRYPTION KEY WITH ALGORITHM = AES_256; ALTER DATABASE [YourDatabaseName] SET ENCRYPTION ON;
– Always Encrypted: For highly sensitive data like credit card information or Social Security numbers, Always Encrypted provides additional protection by encrypting data within the database.
7. Use Database-Level Security Controls
MSSQL has a variety of built-in security features to control access and protect your data.
– Role-Based Access Control (RBAC): MSSQL allows you to assign roles and limit access to specific users. For example, you could create a read-only role for users who only need to view data.
– Row-Level Security: This feature restricts data access at the row level, allowing different users to view different subsets of data.
CREATE SECURITY POLICY FilterPolicy ADD FILTER PREDICATE dbo.FilterFunction(user_id) ON dbo.SensitiveDataTable;
– Audit Logging: Enable SQL Server Audit to track database activities and detect unusual access patterns or suspicious behaviors.
8. Limit Database Permissions and Connections
Assigning minimal privileges and limiting database connections helps contain potential damage in case of a breach.
– Principle of Least Privilege: Limit each user’s permissions to only what is necessary. Avoid using the sa
account in your application.
– Connection Pooling: Use connection pooling to reduce the number of open connections and improve performance. Pooling also minimizes the risk of denial-of-service (DoS) attacks.
9. Implement Firewalls and Network Security
Isolating your MSSQL database server behind a firewall and setting up appropriate network security rules can protect against unauthorized access.
– Database Server Isolation: Place your database in a private network (VPC or VPN) that is not publicly accessible. This limits access to your internal application servers only.
– IP Whitelisting: Restrict access to your MSSQL server based on IP addresses, only allowing connections from trusted networks.
– Database Firewalls: Use SQL Server’s built-in firewall or network firewalls to block unauthorized IP addresses from accessing your database.
10. Regular Backups and Disaster Recovery Planning
Even with the best security measures, there’s always a risk of data loss. Regular backups and a disaster recovery plan ensure business continuity.
– Automated Backups: Schedule automated backups for both the database and the transaction logs. Ensure backups are stored securely and periodically tested for integrity.
– Disaster Recovery Plan: Have a well-defined plan for restoring data and system access in case of a security breach. Document and regularly update the plan to keep it current.
Conclusion
Securing your Node.js API and MSSQL database requires a layered approach, encompassing everything from input validation and query optimization to encryption and role-based access. By following best practices and implementing these strategies, you can significantly reduce vulnerabilities and protect your data. Regular maintenance, backups, and monitoring further ensure the security and availability of your application, providing a more reliable and trustworthy experience for users.
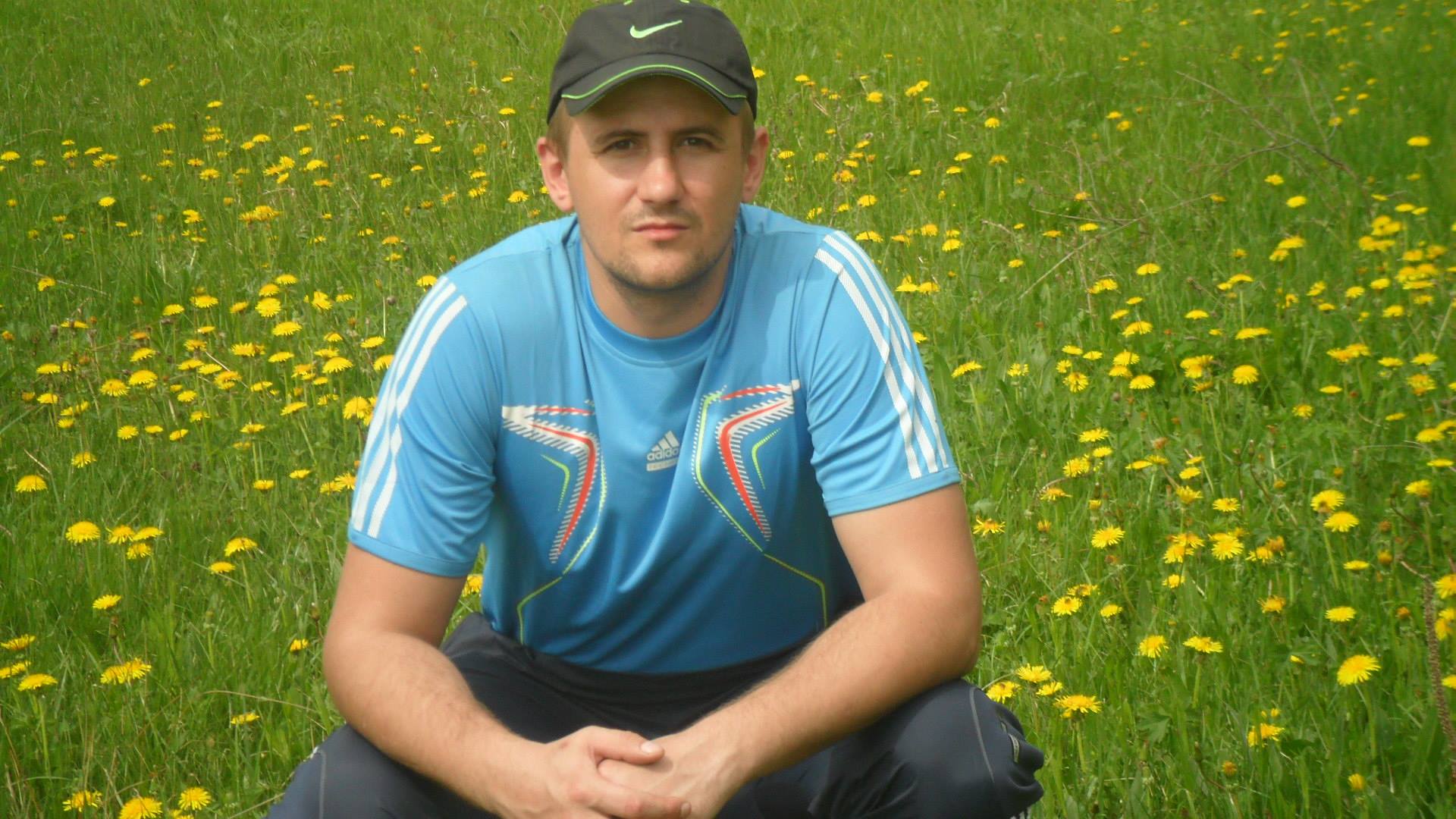
Yury Sobolev is Full Stack Software Developer by passion and profession working on Microsoft ASP.NET Core. Also he has hands-on experience on working with Angular, Backbone, React, ASP.NET Core Web API, Restful Web Services, WCF, SQL Server.