If you are a .net programmer you may be familiar with the below-shown exception which says IO exception was unhandled, the process cannot access the file because it is being used by another process. In this article, I explain the sample code to get rid of this exception.
At times, when you try to access a file programmatically you will get a message that Files not accessible.
In such cases, you can find out various reasons for non-accessibility of the file like,
1) File you are trying to open not even exist
2) File is in process by another thread
3) The file is already kept open etc.
How can we identify that the file is not available for access before trying to open the file?
See the below-given code sample that you can use to identify whether the file is in use or not.
Here the IOException was captured to check whether the file is available for access or not. Even though it is not the best approach this will do your work.
Use the below-given function
/// /// If file already open or in use by any other thread this function /// return true. Else this function return false /// protectedvirtualbool IsFileInUse(string filePath) { FileStream fileStream = null; FileInfo fInfo = newFileInfo(filePath); try { fileStream = fInfo.Open(FileMode.Open); } catch (IOException) { //File in use (file is already open or the file is locked by another thread). return true; } finally { if (fileStream != null) fileStream.Close(); } //file not in use so return false return false; }
The usage sample of this new method is:
//D:\\test.xlsx is the file you are trying to open if (IsFileInUse("D:\\test.xlsx")) MessageBox.Show("File already in use");w
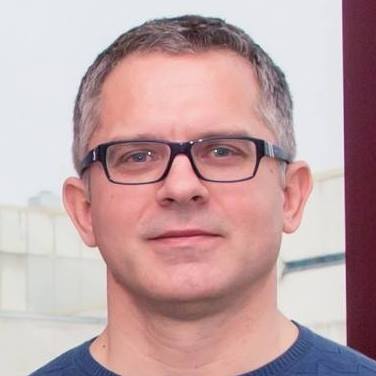
Javier is Content Specialist and also .NET developer. He writes helpful guides and articles, assist with other marketing and .NET community work