In this article we will discuss about new error that you might see when you deploying your Asp.net core project. The error is like:
Could not load type 'Microsoft.AspNetCore.Http.Internal.FormFile' from assembly 'Microsoft.AspNetCore.Http, Version=8.0.0.0, ...'
This error happened because Microsoft.AspNetCore.Http.Internal.FormFile
is no longer accessible in .NET 6+ and especially not in .NET 8. The Internal
namespace indicates that this class was never meant for public use, and it has been removed or made internal in later versions of ASP.NET Core.
How to Fix It
1. If your code tries to manually create or work with FormFile
using Microsoft.AspNetCore.Http.Internal.FormFile
, update it to use the public FormFile
class instead, which is available under:
Microsoft.AspNetCore.Http.FormFile
If you’re manually creating a FormFile
, you can do something like:
using Microsoft.AspNetCore.Http; using System.IO; var fileStream = new MemoryStream(yourFileBytes); var formFile = new FormFile(fileStream, 0, fileStream.Length, "name", "filename.txt") { Headers = new HeaderDictionary(), ContentType = "text/plain" };
2. Make sure your project references the correct version of the required packages.
In your .csproj
, you may need to ensure you are referencing the correct version:
<PackageReference Include="Microsoft.AspNetCore.Http" Version="8.0.0" />
Or use the ASP.NET Core shared framework via the SDK in your app’s target framework:
<TargetFramework>net8.0</TargetFramework>
Important Things to Know
-
Unit Tests: If you’re mocking file uploads in tests, use
FormFile
from the correct namespace. -
Third-Party Libraries: If a library still references
Internal.FormFile
, it’s outdated and incompatible with .NET 8. You’ll need to either downgrade your framework or find a maintained version of that library.
Conclusion
The error “Could not load type ‘Microsoft.AspNetCore.Http.Internal.FormFile'” occurs because you’re using an internal, now-removed class that was never intended for public use. In .NET 6 and beyond (including .NET 8), this class is no longer accessible.
To fix it:
-
Use the public
Microsoft.AspNetCore.Http.FormFile
class instead. -
Update any old code or test mocks that reference the internal type.
-
Make sure your project targets the correct .NET version and references the proper packages.
Bottom line: Avoid relying on internal APIs — always use public, supported types for compatibility with future .NET versions.
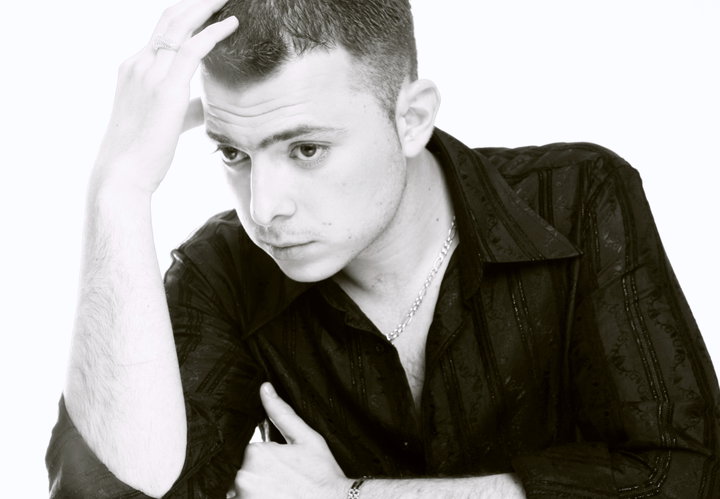
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.