One of the core tenets of Agile is incremental and iterative development. ASP.NET Core’s modular architecture supports this by allowing developers to build applications in smaller, manageable components.
- Modularity: With ASP.NET Core, developers can create separate middleware components, controllers, and services. These can be independently developed, tested, and deployed, aligning well with Agile sprints.
- Flexibility in Updates: Changes or updates to individual modules do not disrupt the entire application. Teams can roll out features incrementally, meeting Agile goals of frequent deliveries.
Example
Using Dependency Injection (DI), a cornerstone of ASP.NET Core, developers can build loosely coupled components. This approach simplifies testing and future-proofing, as components can be updated independently.
2. Built-in Support for CI/CD
Continuous Integration (CI) and Continuous Deployment (CD) are essential practices in Agile development. ASP.NET Core seamlessly integrates with CI/CD pipelines to ensure quick and reliable deployment.
- Integration with DevOps Tools: ASP.NET Core supports tools like Azure DevOps, GitHub Actions, and Jenkins, enabling automated builds, testing, and deployments.
- Rapid Feedback: Developers receive quick feedback about code quality, allowing for iterative improvement during Agile sprints.
3. Cross-Platform Development
Agile teams often prioritize delivering software that works across diverse environments and platforms. ASP.NET Core is designed to run seamlessly on Windows, Linux, and macOS, catering to a wide range of customer needs.
- Unified Codebase: Teams can use the same codebase to build web applications, APIs, and services that work on multiple platforms.
- Docker Integration: ASP.NET Core supports containerization with Docker, making it easier to replicate production environments during development.
Example
Developers can use Docker Compose to create isolated containers for ASP.NET Core apps, enabling Agile teams to test features in environments that mirror production settings.
4. Enhanced Collaboration with Open Source Ecosystem
Agile emphasizes collaboration, and ASP.NET Core’s open-source nature encourages it by default. Developers can contribute to the framework, access community resources, and leverage third-party libraries.
- GitHub Repository: ASP.NET Core’s source code is available on GitHub, enabling developers to understand, modify, and extend the framework.
- Community Contributions: A vibrant community of developers actively shares libraries and tools that can accelerate development.
Example
Developers can integrate community-driven tools like Serilog (for logging) or AutoMapper (for object mapping) to streamline common tasks and focus on delivering business value.
5. Lightweight and High Performance
Agile focuses on delivering high-quality software rapidly, and ASP.NET Core is optimized for performance and scalability.
- Minimal Overhead: ASP.NET Core applications use Kestrel, a lightweight web server, ensuring faster request handling.
- Optimized Middleware: Developers can configure only the required middleware components, reducing unnecessary processing and speeding up application response times.
Example
The following middleware pipeline configuration ensures only essential middleware is used:
app.UseRouting(); app.UseAuthentication(); app.UseAuthorization(); app.UseEndpoints(endpoints => { endpoints.MapControllers(); });
This configuration minimizes overhead and aligns with Agile principles of efficiency and value delivery.
6. Testing and Quality Assurance
Testing is integral to Agile’s goal of maintaining high-quality standards. ASP.NET Core simplifies testing through built-in features and compatibility with testing frameworks.
- Unit Testing: Developers can write unit tests for controllers, services, and other components using frameworks like xUnit or NUnit.
- Integration Testing: ASP.NET Core supports in-memory hosting, making it easier to test APIs without deploying them to a web server.
Example
Using the Microsoft.AspNetCore.Mvc.Testing
package, developers can write integration tests to validate API endpoints within the application.
7. Rapid Prototyping and MVP Development
Agile encourages delivering Minimum Viable Products (MVPs) to gather user feedback early. ASP.NET Core accelerates this process with features that simplify development.
- Scaffolding Tools: Quickly generate controllers, views, and models using command-line tools like
dotnet-aspnet-codegenerator
. - Razor Pages: A streamlined approach to building lightweight web applications with minimal coding.
Example
Generate a new controller using scaffolding:
dotnet aspnet-codegenerator controller -name ProductController -api -m Product -dc AppDbContext
This command generates a fully functional API controller in seconds.
8. Security and Compliance
Security is a critical aspect of software quality. ASP.NET Core provides built-in security features to protect applications without slowing down Agile workflows.
- Authentication and Authorization: ASP.NET Core Identity supports robust authentication mechanisms, including OAuth, JWT, and external providers.
- Data Protection API: Protect sensitive data with encryption and key management.
Example
Secure an API endpoint with role-based authorization:
[Authorize(Roles = "Admin")] [HttpGet("admin-only")] public IActionResult GetAdminData() { return Ok("This data is for admins only."); }
9. Scalability for Future Growth
Agile projects often evolve over time. ASP.NET Core ensures that applications can scale efficiently to accommodate growing user bases.
- Microservices Support: ASP.NET Core is ideal for building microservices that can be independently scaled and updated.
- Cloud Readiness: Built-in support for cloud platforms like Microsoft Azure enables seamless scaling and deployment.
10. Continuous Learning and Improvement
Agile thrives on continuous improvement, and ASP.NET Core aligns with this through regular updates, community support, and robust documentation.
- Regular Updates: Microsoft frequently releases updates, ensuring the framework stays aligned with modern development practices.
- Comprehensive Documentation: Developers can quickly learn new features and best practices, minimizing downtime.
Conclusion
ASP.NET Core is a natural fit for Agile development practices. Its modular architecture, cross-platform capabilities, performance optimizations, and robust ecosystem make it an ideal choice for iterative and collaborative development. By leveraging ASP.NET Core, Agile teams can focus on delivering high-quality, scalable, and secure applications that meet evolving business needs.
Whether you’re building an MVP or scaling an enterprise solution, ASP.NET Core offers the tools and flexibility required to succeed in an Agile environment.
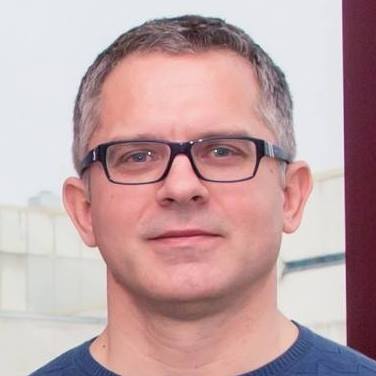
Javier is Content Specialist and also .NET developer. He writes helpful guides and articles, assist with other marketing and .NET community work