As web development continues to evolve, developers often face the dilemma of choosing between various front-end frameworks and libraries. Two powerful options are Blazor and React. While React has long been a dominant force in the JavaScript ecosystem, Blazor—a framework developed by Microsoft—has recently gained traction among .NET developers. It allows building interactive web applications using C# instead of JavaScript. This article explores the Blazor and React frameworks in-depth, comparing their architectures, development models, performance, tooling, and community support, and provides insights on implementation scenarios.
1. Introduction to Blazor and React
What is Blazor?
Blazor is a framework developed by Microsoft that enables developers to build interactive web UIs using C# instead of JavaScript. Blazor runs on either WebAssembly (Wasm) or Server-Side (via SignalR), offering two distinct approaches to how applications are rendered.
Blazor can be categorized into two models:
-
Blazor Server: Executes on the server, and UI events are sent to the client over SignalR.
-
Blazor WebAssembly: Runs on the client, where the application and .NET runtime are downloaded to the browser and executed using WebAssembly.
Blazor enables developers to write client-side web applications entirely in C#, leveraging the full power of the .NET ecosystem for both front-end and back-end development.
What is React?
React is a JavaScript library developed by Facebook (now Meta) for building user interfaces. It employs a component-based architecture that promotes reusability, and utilizes a virtual DOM for efficient updates and rendering.
React is popular for creating Single Page Applications (SPAs), but it can also be used for server-side rendering (SSR) and static site generation (SSG) via frameworks like Next.js. With a rich ecosystem of tools and libraries, React has become one of the most widely adopted frameworks for web development.
2. Language and Development Model
Blazor
Blazor applications are written in C# using Razor syntax, a templating language that mixes HTML with C# code. It integrates closely with the .NET ecosystem, making it ideal for developers familiar with C# and ASP.NET Core.
Key features of Blazor:
-
Strong typing and IntelliSense support for better developer productivity.
-
Dependency Injection support, built directly into the framework.
-
Two-way data binding with
@bind
directive. -
Full access to the .NET libraries, like LINQ, async/await, etc.
Blazor is especially beneficial for .NET developers because they can use the same language (C#) across both front-end and back-end code.
React
React uses JavaScript (or TypeScript for type safety) and JSX (JavaScript XML), a syntax extension that allows you to write HTML-like code inside JavaScript. The React ecosystem relies heavily on functional components and hooks, with libraries like Redux for state management and React Router for navigation.
Key features of React:
-
Component-based architecture for reusable UI elements.
-
Virtual DOM for efficient UI rendering and performance optimizations.
-
Unidirectional data flow for predictability.
-
Strong third-party ecosystem, including UI libraries, routing tools, and testing frameworks.
React’s flexibility gives developers a wide array of tools to structure their applications according to their needs.
3. Architecture
Blazor Server
Blazor Server keeps the application’s logic on the server while maintaining a persistent WebSocket connection to the client. The UI is rendered on the server and sent to the client via SignalR (a real-time web framework). This architecture is highly efficient in terms of initial load but requires a continuous network connection to work properly.
Advantages:
-
Smaller initial download size.
-
Minimal client resource usage.
-
Real-time, instantaneous updates.
Limitations:
-
Dependent on server availability and network latency.
-
Can be resource-intensive on the server side as concurrent users increase.
Blazor WebAssembly
Blazor WebAssembly runs entirely on the client, where the .NET runtime and application code are downloaded to the browser and executed via WebAssembly. This approach removes the dependency on the server once the application has been loaded.
Advantages:
-
No dependency on the server once the application is loaded.
-
Offline support and full client-side execution.
Limitations:
-
Larger initial download size due to WebAssembly’s size.
-
Slower first load time, especially on slower networks.
React
React is a client-side framework by default but can be used with various architectures depending on project needs. It supports:
-
Client-Side Rendering (CSR): Standard approach for SPAs.
-
Server-Side Rendering (SSR): With frameworks like Next.js.
-
Static Site Generation (SSG): For SEO-friendly sites with pre-rendered content.
React’s flexibility allows developers to choose the architecture based on application requirements.
4. Tooling and Ecosystem
Blazor
Blazor benefits from the robust tooling available within the .NET ecosystem. This includes integration with Visual Studio and Visual Studio Code, built-in debugging tools, and access to NuGet packages. Blazor also benefits from the powerful build, test, and publish pipelines of ASP.NET Core.
Commonly used Blazor component libraries include:
-
MudBlazor: A Material Design component library for Blazor.
-
Radzen: A set of rich UI components.
-
Blazorise: Another UI library offering customizable components.
React
React boasts a massive ecosystem and tooling that extends far beyond just React itself. Key tools include:
-
Webpack, Vite, and Parcel for bundling and compiling.
-
React Router for navigation.
-
Redux or Recoil for state management.
-
Jest, Cypress, and React Testing Library for testing.
-
UI component libraries like Material UI, Ant Design, and Chakra UI.
React’s large ecosystem offers a wide variety of choices for developers and provides tools tailored to nearly every use case.
5. Performance
Blazor Server
Blazor Server generally performs well with fast initial loads. However, because of its reliance on server communication, performance can degrade with high network latency and many simultaneous users.
Advantages:
-
Instant load time for initial requests.
-
Minimal client-side resources needed.
Disadvantages:
-
High server-side load for large numbers of users.
-
Latency issues can affect user experience.
Blazor WebAssembly
Blazor WebAssembly provides full client-side execution. However, the first load can be slower compared to JavaScript-based frameworks due to the larger download size of WebAssembly and .NET runtime.
Advantages:
-
After the initial load, the performance is generally good.
-
Runs entirely in the browser without requiring constant server communication.
Disadvantages:
-
Larger initial payload and slower first load.
-
WebAssembly performance might not match native JavaScript for complex applications.
React
React is highly performant due to its virtual DOM and efficient diffing algorithm, which minimizes the number of updates needed. React can also leverage code splitting, lazy loading, and memoization techniques for further optimizations.
React can easily be integrated with SSR via Next.js, which improves performance for SEO-heavy applications.
6. Community and Support
Blazor
Blazor is growing steadily within the .NET community. As an official Microsoft product, it benefits from strong corporate support, regular updates, and integration with the broader .NET ecosystem. However, it is still maturing compared to JavaScript-based solutions.
Advantages:
-
Excellent Microsoft support.
-
Seamless integration with ASP.NET Core and other .NET libraries.
Disadvantages:
-
Smaller community compared to React.
-
Fewer third-party libraries and tools compared to JavaScript-based ecosystems.
React
React has a massive and vibrant community, with years of development and contributions. The number of resources available for React is staggering, and there is a high level of industry adoption.
Advantages:
-
Large, active community and corporate support.
-
Extensive resources, plugins, and libraries.
Disadvantages:
-
React’s ecosystem can be overwhelming due to its large number of tools and libraries.
7. Testing and Debugging
Blazor
Blazor supports debugging through Visual Studio (especially for Blazor Server). However, WebAssembly debugging in browsers is still evolving. Testing tools such as bUnit allow developers to unit test Blazor components, but the testing ecosystem is still catching up to that of JavaScript frameworks.
React
React provides robust testing support, with tools like Jest for unit testing, React Testing Library for component-level tests, and Cypress for end-to-end testing. React’s testing ecosystem is mature, and it’s well-suited for building complex and scalable applications.
Conclusion: When to Choose Blazor or React
When to Choose Blazor
-
If you’re already embedded within the .NET ecosystem and want to build full-stack applications using C#.
-
If you’re building enterprise applications where you need to leverage the power of ASP.NET Core, Entity Framework Core, or SignalR.
-
If your team is primarily skilled in C# and prefers a unified tech stack for both back-end and front-end.
When to Choose React
-
If you need to build highly interactive, dynamic web apps with rich user interfaces.
-
If you require access to a mature, massive ecosystem of third-party libraries, state management tools, and UI frameworks.
-
If you’re working on projects that demand client-side performance and flexibility with server-side rendering.
Final Thoughts
Choosing between Blazor and React ultimately depends on your team’s expertise, the requirements of your project, and the ecosystem you’re already invested in. Blazor shines for .NET developers looking for a seamless integration between client-side and server-side logic. On the other hand, React offers unrivaled flexibility and performance for projects that demand scalability, interactivity, and a rich toolchain.
Both are powerful in their own right, and the best choice comes down to the specific needs of your project. Whether you’re a die-hard C# developer building with Blazor or a JavaScript enthusiast crafting sleek SPAs with React, ASPHostPortal’s hosting environment is fully optimized for both.
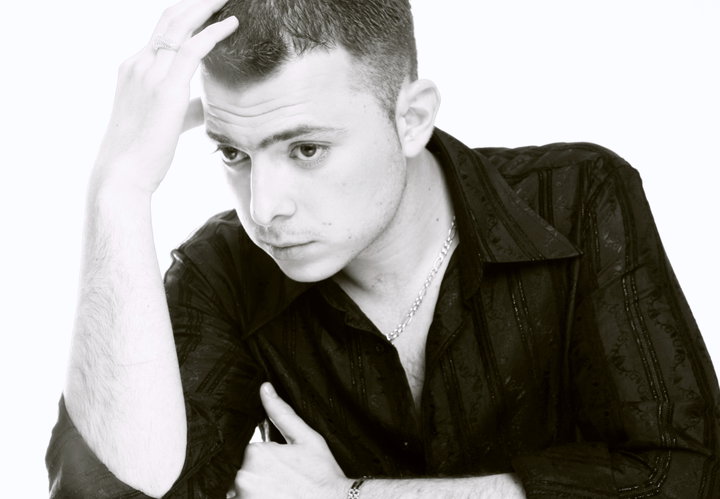
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.