As technology evolves, so do the methods used by cybercriminals to exploit vulnerabilities in websites. Protecting your ASP.NET website in 2025 requires staying updated with the latest security practices and adopting robust strategies to safeguard your application and data. This comprehensive guide explores the essential steps and tools you need to secure your ASP.NET website.
Why Website Security Matters
- Protect Sensitive Data: Websites often handle sensitive user information such as personal details, payment data, and login credentials. A breach can lead to financial and reputational damage.
- Maintain User Trust: Visitors are more likely to trust and engage with a secure website.
- Comply with Regulations: Laws like GDPR, CCPA, and others require websites to implement specific security measures.
- Prevent Downtime: Attacks like DDoS can render your website inaccessible, leading to lost revenue and reduced productivity.
1. Use HTTPS with SSL/TLS
Secure communication between your website and its users is non-negotiable. HTTPS, powered by SSL/TLS certificates, encrypts data during transmission.
Steps to Enable HTTPS:
- Obtain an SSL Certificate:
- Purchase one from a trusted Certificate Authority (CA) or use free certificates from providers like Let’s Encrypt.
- Install the SSL Certificate on your server.
- Enforce HTTPS.
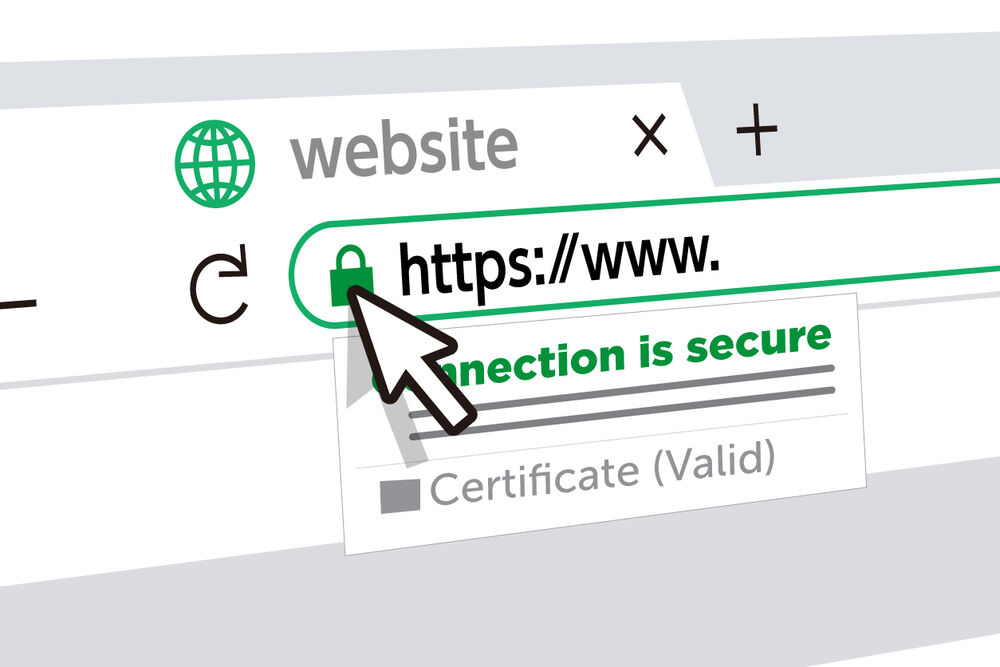
Benefits:
- Encrypts sensitive data.
- Prevents man-in-the-middle (MITM) attacks.
- Boosts SEO rankings (as Google prioritizes HTTPS websites).
2. Regularly Update Your Software
Outdated software is a prime target for attackers. Ensure all components of your application are up-to-date, including:
- ASP.NET Framework/Core: Upgrade to the latest stable version.
- Third-party Libraries: Monitor dependencies using tools like NuGet or Dependabot.
- Server Software: Keep your web server (e.g., IIS) updated with the latest security patches.
3. Implement Strong Authentication and Authorization
Weak authentication systems are a common entry point for attackers.
Best Practices:
- Enforce Strong Passwords: Use tools like ASP.NET Core Identity to implement password policies.
- Enable Multi-Factor Authentication (MFA): Add an extra layer of security by requiring a second form of verification.
- Role-Based Access Control (RBAC): Restrict access to sensitive resources using role-based permissions.
Example of Role-Based Authorization:
[Authorize(Roles = "Admin")]
public IActionResult AdminDashboard()
{
return View();
}
4. Protect Against SQL Injection
SQL injection is a common attack where malicious SQL queries are executed against your database.
Preventive Measures:
1. Use Parameterized Queries:
2. ORMs like Entity Framework: Automatically handle parameterization and reduce SQL injection risks.
3. Validate User Inputs: Sanitize and validate inputs before processing.
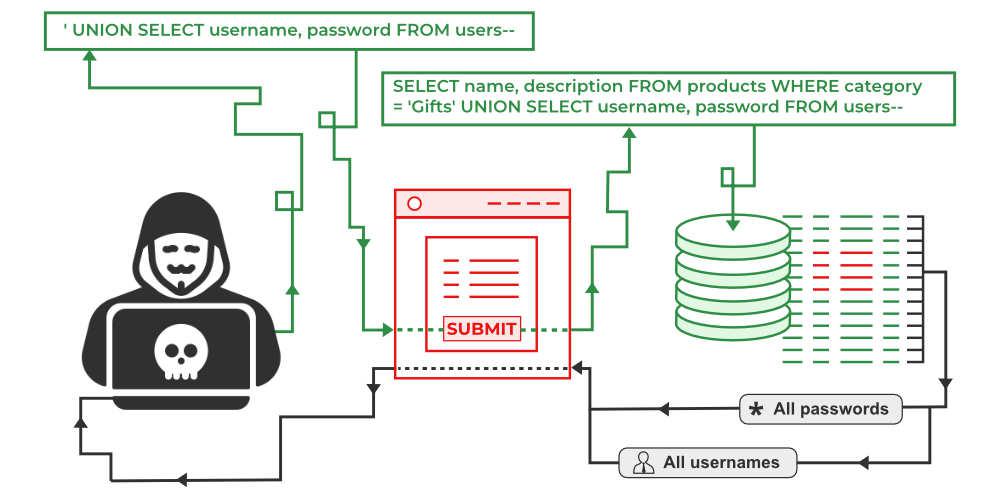
5. Secure Your APIs
APIs are often targeted by attackers to exploit vulnerabilities in web applications.
Security Measures:
- Use Authentication Tokens:
- Implement OAuth2.0 or JWT for secure token-based authentication.
- Rate Limiting:
- Prevent abuse by limiting the number of API requests per user.
- Encrypt API Traffic: Use HTTPS for secure API communication.
6. Protect Against Cross-Site Scripting (XSS)
XSS attacks occur when attackers inject malicious scripts into your application.
Mitigation Techniques:
- Output Encoding:
- Use the
HtmlEncode
method to encode user inputs before rendering.
- Content Security Policy (CSP):
- Configure a CSP header to control what resources can be loaded.
app.UseCsp(options => options
.DefaultSources(s => s.Self())
.Scripts(s => s.Self()));
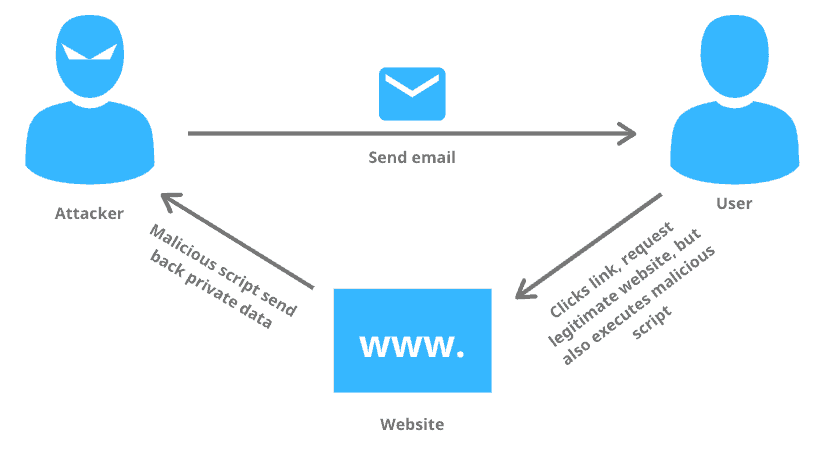
A Web Application Firewall (WAF) is a critical security tool designed to protect web applications by filtering and monitoring HTTP traffic between a web application and the internet. WAFs defend against common cyber threats such as SQL injection, cross-site scripting (XSS), and more. To maximize the effectiveness of a WAF, you need to follow best practices for its implementation and management.
WAFs come in three main types, and selecting the right one depends on your application architecture, hosting environment, and budget:
- Cloud-Based WAF: Ideal for applications hosted in the cloud. Examples include Cloudflare, AWS WAF, and Azure Application Gateway.
- Advantages: Easy deployment, scalability, and managed updates.
- Use Case: Applications requiring fast and global coverage.
- Hardware-Based WAF: Deployed on-premises, offering high performance for local infrastructure.
- Advantages: Complete control over deployment.
- Use Case: Large enterprises with dedicated data centers.
- Software-Based WAF: Deployed within the application environment, often as part of a DevOps workflow.
- Advantages: Flexibility and customization.
- Use Case: Companies adopting containerization or microservices.
Benefits of Using a WAF
- Protection Against Common Threats: Safeguards your application from OWASP Top 10 vulnerabilities.
- Improved Compliance: Helps meet security requirements for regulations like PCI DSS, GDPR, and HIPAA.
- Simplified Management: Many WAFs offer intuitive dashboards and analytics for easy configuration and monitoring.
8. Enable Logging and Monitoring
Logging and monitoring are crucial for detecting and responding to security incidents.
Tools:
- Application Insights: Provides real-time monitoring and analytics for ASP.NET applications.
- Serilog: A flexible logging library for structured logging in .NET.
Example of Logging:
var logger = LoggerFactory.Create(builder => builder.AddConsole()).CreateLogger<Program>();
logger.LogInformation("Application started.");
9. Protect Your Database
Databases are a common target for attackers.
Security Measures:
- Secure Database Credentials:
- Store sensitive credentials securely in Azure Key Vault or environment variables.
- Enable Database Encryption:
- Use Transparent Data Encryption (TDE) for SQL Server.
- Restrict Database Access:
- Use IP whitelisting and role-based permissions.
10. Conduct Regular Security Audits
Regular security assessments help identify vulnerabilities before attackers do.
Tools for Audits:
- OWASP ZAP: Open-source tool for finding vulnerabilities.
- Burp Suite: Professional tool for advanced web security testing.
11. Backup and Disaster Recovery
With the increasing risks of cyberattacks, accidental data loss, and hardware failures, having a robust backup and disaster recovery plan is essential. ASPHostPortal, a trusted hosting provider, goes above and beyond to offer state-of-the-art solutions for backup and disaster recovery, ensuring your website remains secure, available, and resilient even in the face of challenges.
Why Backup and Disaster Recovery Are Essential
- Safeguard Against Data Loss
Data loss can occur due to various reasons, such as server crashes, human errors, or malicious attacks. Regular backups act as a safety net, allowing you to restore your website to its previous state.
- Minimize Downtime
Downtime can lead to revenue loss, decreased customer trust, and a damaged reputation. Disaster recovery ensures rapid restoration of services, minimizing the impact on your business.
- Compliance with Regulations
Many industries require organizations to have secure backups to comply with data protection regulations like GDPR, HIPAA, or PCI DSS.
- Business Continuity
Backup and disaster recovery solutions are essential for maintaining operations during unforeseen events, ensuring business continuity.
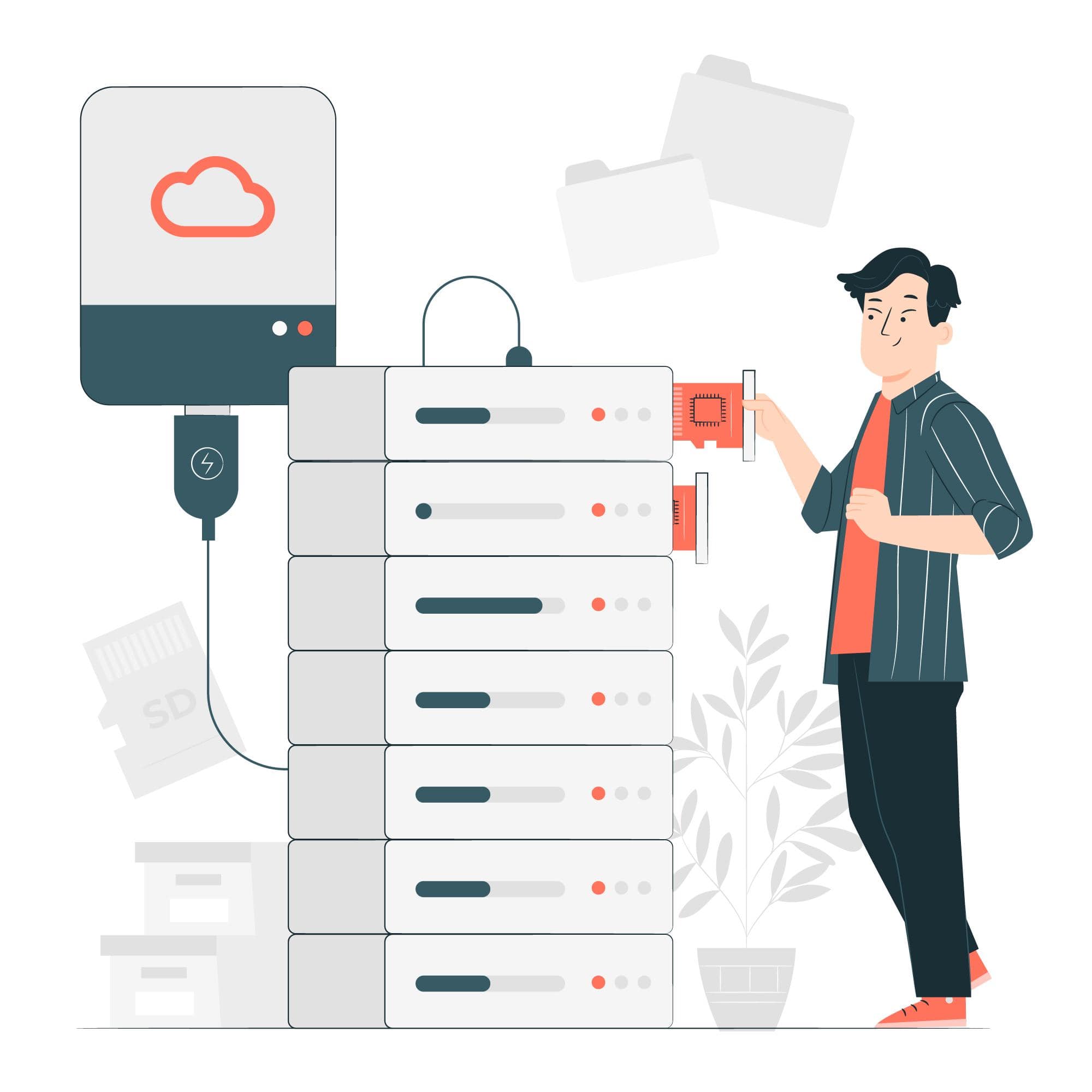
Backup Solutions Offered by ASPHostPortal
ASPHostPortal provides comprehensive backup features tailored to different needs.
1. Automated Daily Backups
ASPHostPortal offers automated daily backups to ensure your data is always up to date. These backups include:
- Website files
- Databases (MSSQL, MySQL)
- Email accounts
2. Custom Backup Options
Users can configure backup settings based on their requirements. Whether you need hourly, daily, weekly, or on-demand backups, ASPHostPortal provides flexibility.
3. Offsite Storage
To enhance data security, backups are stored offsite, ensuring they remain safe even if the primary server encounters issues.
4. Retention Period
ASPHostPortal allows users to specify retention periods, giving you the ability to restore data from a specific point in time.
5. One-Click Restore
The intuitive control panel offers a one-click restore feature, enabling you to recover your website or database quickly and efficiently.
12. Educate Your Team
Your team plays a crucial role in maintaining security.
Steps:
- Conduct regular training on secure coding practices.
- Stay updated on the latest security trends and threats.
- Encourage adherence to a security-first mindset.
Conclusion
Securing your ASP.NET website in 2025 involves a combination of proactive measures, advanced tools, and a commitment to continuous improvement. By implementing HTTPS, staying updated, protecting against common vulnerabilities, and educating your team, you can create a secure and reliable environment for your users. Hosting your application with providers like ASPHostPortal adds an extra layer of security, ensuring your website is protected against evolving cyber threats.
Stay vigilant, invest in security, and make your ASP.NET website a fortress in the digital landscape of 2025.
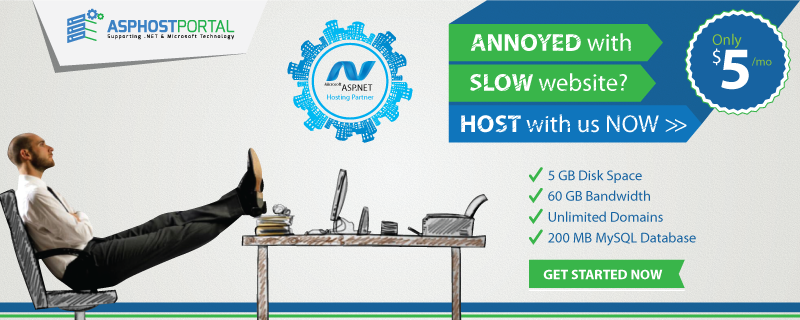
Javier is Content Specialist and also .NET developer. He writes helpful guides and articles, assist with other marketing and .NET community work