ASP.NET Core has become a leading framework for building modern, scalable, and high-performance web applications. However, even experienced developers can make mistakes that affect their application’s performance, security, or maintainability. This article explores the top 10 mistakes ASP.NET Core developers should avoid and provides actionable advice to improve your development workflow.
1. Ignoring Dependency Injection Best Practices
ASP.NET Core relies heavily on dependency injection (DI) to manage service lifetimes and promote modularity. However, developers often misuse it by:
- Registering services with incorrect lifetimes (e.g., using
Singleton
instead ofScoped
). - Overloading the DI container with unnecessary or heavy services.
- Using the DI container for tightly coupled services.
Solution:
Understand the different service lifetimes (Singleton
, Scoped
, Transient
) and their implications. Use DI for loosely coupled components only, and avoid overusing it for trivial dependencies.
2. Hardcoding Configuration Values
Hardcoding values such as connection strings, API keys, or environment-specific variables can lead to inflexible and insecure applications.
Solution:
Leverage the Options Pattern and the appsettings.json file.
3. Overlooking Middleware Order
Middleware in ASP.NET Core processes requests in a pipeline. Incorrect middleware ordering can lead to unexpected behavior or security vulnerabilities.
Example Mistake: Placing UseAuthentication()
after UseEndpoints()
, which prevents authentication logic from executing.
Solution:
Refer to Microsoft’s middleware ordering documentation and test your pipeline thoroughly to ensure proper execution.
4. Blocking Async Code with .Wait()
or .Result
Blocking asynchronous calls using .Wait()
or .Result
can lead to deadlocks and performance issues, especially in web applications where threads are a limited resource.
Solution:
Adopt an end-to-end asynchronous approach. Use async/await
keywords throughout the application and ensure that synchronous and asynchronous methods are not mixed.
5. Failing to Implement Proper Exception Handling
Uncaught exceptions can crash applications or expose sensitive data to users. Many developers forget to centralize exception handling or log critical errors.
Solution:
Use ASP.NET Core’s built-in Exception Middleware (UseExceptionHandler
) to log and handle exceptions gracefully. Customize error responses for production environments.
6. Not Optimizing Database Queries
Inefficient database queries, such as over-fetching data or using unoptimized joins, can slow down applications significantly.
Solution:
- Use Entity Framework Core features like
AsNoTracking
for read-only operations. - Implement pagination for large datasets.
- Profile and optimize SQL queries using tools like SQL Profiler or EF Core logging.
7. Ignoring Security Best Practices
Security oversights like using weak authentication mechanisms, not validating inputs, or exposing sensitive endpoints can make your application vulnerable.
Solution:
- Use ASP.NET Core’s built-in security features like Data Protection API, Authentication, and Authorization Middleware.
- Validate inputs to prevent SQL injection and other common vulnerabilities.
- Always use HTTPS for communication.
8. Overlooking Caching Strategies
Neglecting caching results in slower response times and increased server load. Some developers either don’t implement caching or use outdated methods.
Solution:
Leverage Distributed Cache, In-Memory Cache, or Response Caching Middleware in ASP.NET Core. For distributed systems, integrate with caching services like Redis or Memcached.
9. Poor Logging Practices
Logging is essential for debugging and monitoring. Developers often make mistakes such as logging too much data, omitting critical logs, or writing logs manually instead of using structured logging frameworks.
Solution:
Use Serilog, NLog, or ASP.NET Core’s default logging to manage logs effectively. Implement different log levels (e.g., Information, Warning, Error) and avoid logging sensitive information.
10. Not Using Health Checks for Monitoring
Many developers neglect to implement health checks to monitor application and service health in production environments.
Solution:
ASP.NET Core provides a built-in Health Checks API. Use it to monitor database connections, service availability, and other critical components. Integrate it with monitoring tools like Prometheus or Azure Monitor for real-time insights.
Conclusion
Avoiding these common mistakes in ASP.NET Core development will lead to better performance, improved security, and maintainable applications. By adhering to best practices such as proper middleware ordering, dependency injection, and security protocols, developers can build robust applications that meet modern user expectations.
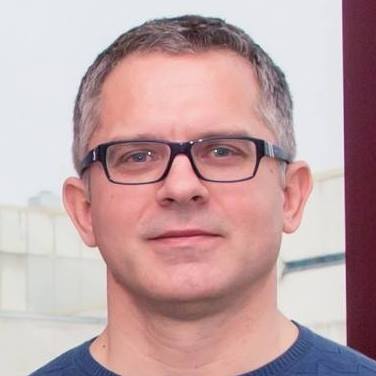
Javier is Content Specialist and also .NET developer. He writes helpful guides and articles, assist with other marketing and .NET community work