Improving the performance of a Blazor website is essential to provide a smooth user experience, especially given that Blazor relies on WebAssembly, a powerful but sometimes resource-intensive technology for client-side applications. Here’s a detailed guide to help you optimize Blazor’s performance using a combination of optimization techniques and tutorials.
1. Optimize Component Rendering and Minimize Component State
Example: Blazor renders UI components in response to state changes, which means frequent or unnecessary updates can cause performance lags.
Solution: Use ShouldRender()
to control when the component should render.
protected override bool ShouldRender() { // Render only if specific conditions are met return hasDataChanged; }
Explanation: By implementing ShouldRender
, you prevent Blazor from re-rendering components unnecessarily, saving resources and improving performance.
2. Use Asynchronous Data Loading
Loading data synchronously can block your UI, especially when dealing with large datasets or remote data sources. Loading data asynchronously allows Blazor to continue rendering while fetching data in the background.
Example:
Explanation: Loading data asynchronously ensures that components load faster and are responsive while waiting for data, improving the user experience.
3. Leverage Lazy Loading for Non-Essential Content
Lazy loading is a great way to defer loading non-essential resources or components until they are actually needed.
Example:
Explanation: Using lazy loading in Blazor applications reduces initial load time and improves the perceived speed of your website, as content loads progressively rather than all at once.
4. Optimize JavaScript Interoperability
In Blazor, JavaScript Interop (JSInterop) enables calling JavaScript from C# and vice versa, which is beneficial for performance but must be used judiciously.
Example: Avoid calling JavaScript frequently in a loop or render cycle, as it can slow down the app.
// Only call this once or sparingly await JS.InvokeVoidAsync("console.log", "Message from Blazor!");
Explanation: Too many calls to JavaScript can hinder Blazor’s performance due to the additional processing required to switch contexts between C# and JavaScript.
5. Minimize the Size of Blazor Application
Blazor applications involve downloading .NET assemblies to the client, which can slow down the initial load. Minimizing the size of these files can make a big difference.
Solution:
- Enable AOT (Ahead-of-Time) compilation.
- Strip out unnecessary libraries and dependencies.
Explanation: By reducing the download size of your application, Blazor loads faster on the client side, improving performance significantly.
6. Implement Server-Side Blazor if Client-Side Resources Are Limited
While Blazor WebAssembly runs on the client, Blazor Server operates on the server, only sending necessary data to the client. This reduces the amount of data transferred and improves performance, especially in environments with limited client resources.
Example: Migrate to Blazor Server by changing your project template to a Blazor Server App instead of a Blazor WebAssembly App.
Explanation: Blazor Server Apps work well in scenarios where real-time data updates are required and where client devices may have limited resources, improving responsiveness and performance.
7. Reduce HTTP Requests with Caching
Making repeated HTTP requests slows down applications and increases server load. Instead, use caching to save frequently accessed data locally.
Example: Store data in the browser’s local storage using JavaScript interop and only fetch new data if needed.
if (!IsDataInLocalStorage()) { Data = await Http.GetJsonAsync<DataType>("api/data"); SaveDataToLocalStorage(Data); }
Explanation: Caching data on the client allows you to avoid unnecessary requests and improves load times by serving data from the client’s storage rather than the server.
8. Use a Content Delivery Network (CDN) for Static Assets
Offload static assets like images, stylesheets, and scripts to a CDN to decrease load time and increase scalability.
Example: Store assets on a CDN and use those URLs in your Blazor components.
<img src="https://cdn.example.com/image.png" alt="Optimized image" />
Explanation: CDNs reduce server load and speed up asset delivery by caching static files closer to the user, making your Blazor app load faster.
9. Enable Compression on Server
Compressing files before sending them to the client can drastically reduce load times.
Example: Configure compression in ASP.NET Core for the server hosting your Blazor app.
public void ConfigureServices(IServiceCollection services) { services.AddResponseCompression(options => { options.MimeTypes = ResponseCompressionDefaults.MimeTypes.Concat( new[] { "application/octet-stream" }); }); }
Explanation: Enabling compression reduces the file sizes sent to the client, resulting in faster load times for the Blazor application.
10. Profile and Measure Performance Regularly
Use performance profiling tools to identify bottlenecks in your Blazor application.
Example: Use tools like the Chrome Developer Tools or Visual Studio’s Profiler to analyze load times and memory usage.
Explanation: Regular performance profiling helps detect slow-performing areas in your code and make necessary adjustments to enhance performance.
Conclusion
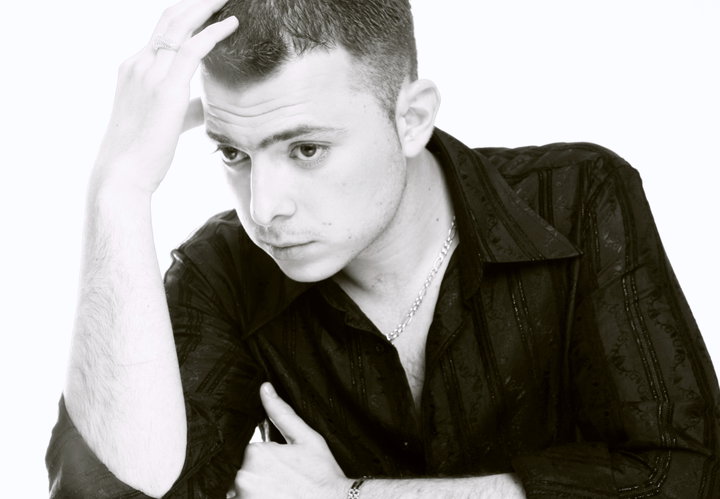
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.