Microsoft has just launched new ASP.NET 8 and they have been working hard to make Asp.net core the best framework ever. They actively listen to community and keep improving their features. The following are 5 Asp.net core features that you will love and I will describe it all for you on this article
1. Global Exception Handling
If you’re a seasoned ASP.net core developer, you’ve undoubtedly already put in place measures to log exceptions, catch them, respond to clients appropriately, and make sure that no important data is released into the wild. Numerous blog articles and nugget packages were made available over time to automate this procedure. Now, adding it to ASPnet Core on DNN8 requires little work.
The “IExceptionHandler” type added in Dotnet 8 makes this possible. An exception handler middleware was added to the ASPnet core in earlier versions of DotNet. However, this interface allows you to add some custom logic to handle exceptions in the background.
For your exception-handling logic, you must first implement this interface. You can implement as many as you like, and they will be carried out in registration order.
public interface IExceptionHandler { ValueTask<bool> TryHandleAsync(HttpContext httpContext, Exception exception, CancellationToken cancellationToken); }
Then, register it as follow. Where “T” is the type of your Exception handler class.
IServiceCollection.AddExceptionHandler<T>
2. Support for native AOT in Asp.net core
AOT: Ahead of Time Compilation
This is the process of compiling source code written in a high-level programming language into a lower-level language before it is executed.
JIT: Just in Time Compilation
This is the process of compiling a program while it is running. This has always been used in dotnet to translate any dotnet language into machine code when necessary. When a “.exe” or dll containing dotnet language code (such as C#, F#, etc.) is executed, the JIT compiler converts the CIL code into the native code of the underlying operating system.
With the release of Dotnet 7 last year, we could now compile and publish console applications as native AOT. We can now compile and release ASP.net core apps as native AOT in dotnet 8. However, as Microsoft notes here (https://devblogs.microsoft.com/dotnet/asp-net-core-updates-in-dotnet-8-preview-3/#asp-net-core-support-for-native-aot), the initial focus will be on cloud-native API applications.
What is altered by this?
Once you comprehend JIT and contrast it with AOT, the distinction will become evident immediately. Without the dotnet runtime installed on the device, the software you write could be deployed as a platform-specific package that is faster to launch, smaller in size, and uses less memory.
3. Add Authorization Policies Easily
Authorization policies require the writing of a lot of code to be implemented. particularly if you plan to build unique policy attributes. With Asp.net Core’s new feature in Dotnet 8, authorization policies can be easily created with fewer lines of code. The inclusion of IAuthorizationRequirementData makes this feasible. This has the benefit that the authorization policy’s requirements can also be specified in the attribute definition.
I’ll give you an example right away to show you how this is an improvement. You’ll quickly see why this is fantastic when you contrast it with the previous method of creating authorization policies. This is an example taken from a Microsoft repository. You will be able to clearly see the improvement in this demo.
[ApiController] [Route("api/[controller]")] public class GreetingsController : Controller { [MinimumAgeAuthorize(16)] [HttpGet("hello")] public string Hello() => $"Hello {(HttpContext.User.Identity?.Name ?? "world")}!"; } class MinimumAgeAuthorizeAttribute : AuthorizeAttribute, IAuthorizationRequirement, IAuthorizationRequirementData { public MinimumAgeAuthorizeAttribute(int age) => Age =age; public int Age { get; } public IEnumerable<IAuthorizationRequirement> GetRequirements() { yield return this; } } class MinimumAgeAuthorizationHandler : AuthorizationHandler<MinimumAgeAuthorizeAttribute> { private readonly ILogger<MinimumAgeAuthorizationHandler> _logger; public MinimumAgeAuthorizationHandler(ILogger<MinimumAgeAuthorizationHandler> logger) { _logger = logger; } // Check whether a given MinimumAgeRequirement is satisfied or not for a particular context protected override Task HandleRequirementAsync(AuthorizationHandlerContext context, MinimumAgeAuthorizeAttribute requirement) { // Log as a warning so that it's very clear in sample output which authorization policies // (and requirements/handlers) are in use _logger.LogWarning("Evaluating authorization requirement for age >= {age}", requirement.Age); // Check the user's age var dateOfBirthClaim = context.User.FindFirst(c => c.Type == ClaimTypes.DateOfBirth); if (dateOfBirthClaim != null) { // If the user has a date of birth claim, check their age var dateOfBirth = Convert.ToDateTime(dateOfBirthClaim.Value, CultureInfo.InvariantCulture); var age = DateTime.Now.Year - dateOfBirth.Year; if (dateOfBirth > DateTime.Now.AddYears(-age)) { // Adjust age if the user hasn't had a birthday yet this year age--; } // If the user meets the age criterion, mark the authorization requirement succeeded if (age >= requirement.Age) { _logger.LogInformation("Minimum age authorization requirement {age} satisfied", requirement.Age); context.Succeed(requirement); } else { _logger.LogInformation("Current user's DateOfBirth claim ({dateOfBirth}) does not satisfy the minimum age authorization requirement {age}", dateOfBirthClaim.Value, requirement.Age); } } else { _logger.LogInformation("No DateOfBirth claim present"); } return Task.CompletedTask; }
4. New Asp.net core metrics
In the past, you had to write neat code in conjunction with the telemetry provider’s SDK in order to incorporate telemetry and diagnostic features into your software. Often, you would have to rewrite the instrumentation code if you ever needed to switch vendors. There was an issue here, and it required attention.
System.Diagnostics is meant to handle this.An API for metrics was made. This was created in cooperation with Open Telemetry and offers telemetry cross-platform APIs. Adoption of open telemetry increases telemetry’s adaptability.
What connection does it, however, have to ASP.net Core?
You now see how important it is to establish an industry-wide standard for gathering metrics. Parts of the ASP.net core now have this. Kestrel, Hosting, and Signal-R are among them. Microsoft has provided us with an example source code that includes a Grafana dashboard reporting metrics that Prometheus collected from the ASP.net core application.
“Originally developed at SoundCloud, Prometheus is an open-source systems monitoring and alerting toolkit. Prometheus has been adopted by numerous businesses and organizations since its launch in 2012, and it boasts a vibrant developer and user community.
5. Better Debugging Experience
It’s now more enjoyable to use your source code to play detective. On dotnet 8, locating pertinent and crucial information about types like the HttpContext and WebApplication is much simpler. On the debug window, even IConfiguration values are easily visible. This enhancement is shown in this picture.
Conclusion
So, I have explained 5 best Asp.net 8 features for you. Which features do you like it? FYI, ASPHostPortal already support Asp.net 8 on our hosting environment.
ASPHostPortal is one of the best alternatives for your ASP.NET hosting, offering:
- Better pricing and value for money
- Feature-rich hosting plans
- 99.9% uptime guarantee
- Enhanced security features
- Global data center locations
- Multilingual customer support
Whether you’re a seasoned web developer, a personal blogger, or a business owner, we’ve kept you in mind when designing our hosting services.
We provide everything you need, from developer-made tools like Git integration and access managers for user management to basic features like a free domain name, unlimited bandwidth, and free SSL.
Make the switch to ASPHostPortal right now to see the difference for yourself.
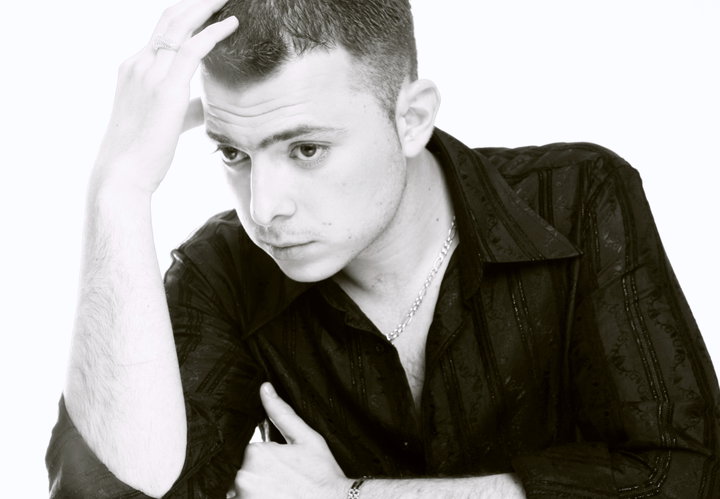
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.