In previous article, we have written article about how to implement session in ASP.NET Core. In this article, we will give some insight about session management in MVC.
What is a Session?
In a stateless online environment, a session is a means for preserving state between requests. It enables you to keep track of information about a user’s interactions with your application, such as preferences, products in their shopping cart, or level of authentication. An exclusive session identification is used to link the user with their data, which is saved on the server.
Session use cases
- User authentication: After a successful login, save the user’s credentials to keep them in an authenticated state throughout different requests.
- Shopping carts: As customers shop in your online store, keep track of the things they have placed to their cart.
- Personalization: Keep user preferences in order to give them a personalized experience while they are there.
Session Management in MVC
Depending on the intended behavior and storage options, MVC applications can manage sessions in a variety of ways.
Session state
A built-in technique in ASP.NET MVC for maintaining session data is called session state. It keeps information in a key-value dictionary so you can access and change session data conveniently throughout your application.
Session storage options
- In-process: the application’s memory is used to store session data. Although it is the default choice, it might not be appropriate for dispersed situations or large applications.
- State server: improves scalability by storing session data in a different process or server.
- SQL Server: improves durability and availability by storing session data in a SQL Server database.
Implementing Session in MVC
In MVC applications, session management can be implemented in a variety of methods.
Using HttpSessionStateBase
HttpSessionStateBase is the standard session object in ASP.NET MVC. You can access it using the Session
property of the Controller
class. This makes it simple to save, retrieve, and delete session data using key-value pairs.
// Store a value in the session Session["username"] = "JohnDoe"; // Retrieve a value from the session string username = (string)Session["username"]; // Remove a value from the session Session.Remove("username");
Custom session objects
A different strategy is to build unique session objects that include your session data. Your code will become more organized and easier to maintain as a result. To work with the session, you can construct a new class for storing session data and using extension methods:
public class UserSession { public int UserId { get; set; } public string Username { get; set; } } public static class SessionExtensions { public static void SetUserSession(this HttpSessionStateBase session, UserSession userSession) { session["UserSession"] = userSession; } public static UserSession GetUserSession(this HttpSessionStateBase session) { return (UserSession)session["UserSession"]; } }
TempData
Another tool in ASP.NET MVC that lets you save data transiently in between requests is called TempData
. It is appropriate for situations like redirecting after form submission because it leverages session storage below but only supports one request at a time.
// Store a value in TempData TempData["message"] = "Your data has been saved."; // Retrieve a value from TempData string message = (string)TempData["message"];
Session Security
It’s critical to take security issues like session fixation and session hijacking into account while working with sessions.
Session fixation
Once a user has been authenticated, an attacker can hijack their session by tricking them into using a session ID they already know. This is known as session fixation. After a successful login, regenerate the session ID to avoid session fixation:
Session.Abandon(); Response.Cookies.Add(new HttpCookie("ASP.NET_SessionId", ""));
Session hijacking
When a hacker obtains a user’s session ID and uses it to assume the user’s identity, this is known as session hijacking. Follow these best measures to prevent session hijacking:
- Encrypt data sent between the client and server using HTTPS.
- To prohibit access via JavaScript, set the session cookies’ HttpOnly attribute.
- Implement appropriate authentication and access controls.
Performance Considerations
It’s crucial to take into account the following elements while managing sessions because they can affect how well your application performs:
Scalability
You might need to scale your session storage as your application expands to cope with the added traffic. When working with distributed or big applications, take into account employing state servers or SQL Server for session storage.
Session timeouts
By default, after 20 minutes of inactivity, session data is immediately deleted. In the configuration file for your application, you can change the session timeout value. Longer timeouts should be used with caution since they can use up more server resources.
Alternatives to Session in MVC
In MVC applications, there are alternatives to sessions, including ViewState, cookies, and application state.
- ViewState: stores information on the client side in a page’s hidden field. It works well for little amounts of data, however it might not be the best option for sensitive data.
- Cookies: tiny text files are used to store data on the client side. Cookies have a maximum size and can be turned off by the user.
- Application state: data is kept on the server side and is available to all users. In contrast to user-specific data, it is helpful for storing global data.
Conclusion
To preserve state over numerous requests, session management is a crucial component of creating MVC applications. In this post, we looked at several session management implementation strategies for MVC apps, security issues, performance issues, and session replacements. You can develop web applications that are more reliable and user-friendly by comprehending these concepts.
Get your ASP.NET hosting as low as $1.00/month with ASPHostPortal. Our fully featured hosting already includes
- Easy setup
- 24/7/365 technical support
- Top level speed and security
- Super cache server performance to increase your website speed
- Top 9 data centers across the world that you can choose.
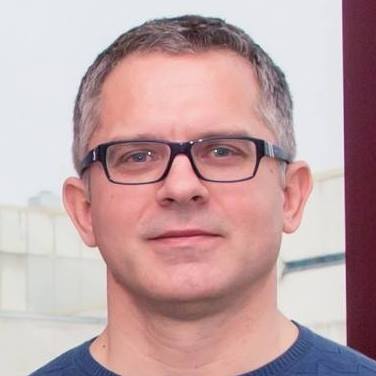
Javier is Content Specialist and also .NET developer. He writes helpful guides and articles, assist with other marketing and .NET community work