If you host your site with shared hosting, you won’t be able to access the server. So, the question now is how can you restart your Asp.net core application if there is a problem? In this article, we will advise you how to restart your ASP.NET Core application programatically. Let’s get started!
IApplicationLifetime
With ASP.NET Core, there is an interface called IApplicationLifetime that can deal with start-up and shutdown events. It offers 3 Cancellation Token, enabling us to use Action Delegate to manage the start and stop events for the ASP.NET Core website:
public void Configure(IApplicationBuilder app, IHostingEnvironment env, IApplicationLifetime appLifetime) { appLifetime.ApplicationStarted.Register(() => { _logger.LogInformation("Moonglade started."); }); appLifetime.ApplicationStopping.Register(() => { _logger.LogInformation("Moonglade is stopping..."); }); appLifetime.ApplicationStopped.Register(() => { _logger.LogInformation("Moonglade stopped."); }); // ... Other code }
Per Microsoft document:
Cancellation Token | Triggered when… |
---|---|
ApplicationStarted | The host has fully started. |
ApplicationStopped | The host is completing a graceful shutdown. All requests should be processed. Shutdown blocks until this event completes. |
ApplicationStopping | The host is performing a graceful shutdown. Requests may still be processing. Shutdown blocks until this event completes. |
Killing the Website
In addition to these three events, the IApplicationLifetime interface has a method called StopApplication() that allows the user to terminate an active ASP.NET Core application. ApplicationStopped and ApplicationStopping events are fired sequentially when a website is closed.
We can use this technique to restart our website.
Implement Restart Functionality
We can restart a website with the most ease by going to a specific URL. Consider the following ASP.NET Core MVC application:
Inject an IApplicationLifetime class into the Controller:
public class AdminController : Controller { IApplicationLifetime applicationLifetime; public AdminController(IApplicationLifetime appLifetime) { applicationLifetime = appLifetime; } // ... }
And use an Action to invoke the StopApplication() method:
[HttpGet("blow-me-up")] public IActionResult BlowMeUp() { applicationLifetime.StopApplication(); return new EmptyResult(); }
Now, when I access the URL “blow-me-up“, the website will shut down itself:
And I can see the steps in log file:
It is simple to restart the application. Under IIS, the webpage will reload upon receiving the subsequent request. Which simply indicates that you should open the website in your browser once more to make it start up.
Conclusion
We hope that article can help you to restart your Asp.net core application in case you need it. To get solutions from other website errors, don’t forget to read other content from the ASPHostPortal blog.
Are you looking for an affordable and reliable Asp.net core hosting solution?
Get your ASP.NET hosting as low as $1.00/month with ASPHostPortal. Our fully featured hosting already includes
- Easy setup
- 24/7/365 technical support
- Top level speed and security
- Super cache server performance to increase your website speed
- Top 9 data centers across the world that you can choose.
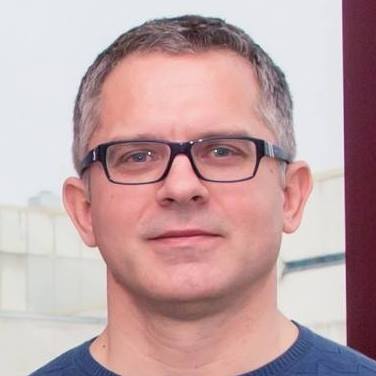
Javier is Content Specialist and also .NET developer. He writes helpful guides and articles, assist with other marketing and .NET community work