Creating background tasks in ASP.NET Core can be an essential part of web development, particularly for performing operations that don’t need direct user interaction, such as sending emails, processing data, or cleaning up resources. In this tutorial, we’ll explore how to schedule background tasks in ASP.NET Core using IHostedService
, along with an example of setting up a simple task scheduler.
1. Understanding Background Tasks in ASP.NET Core
ASP.NET Core provides two primary methods for implementing background tasks:
- Hosted Services (
IHostedService
): This interface allows you to run background tasks independently of your application’s main pipeline. Hosted services start automatically when the application starts and can be set to run continuously, on-demand, or at specific intervals. - Task Scheduling Libraries: Libraries like Quartz.NET provide more advanced scheduling capabilities, making it possible to run tasks at specific intervals, dates, or based on custom triggers.
In this tutorial, we’ll focus on implementing background tasks using the IHostedService
interface and configuring a time interval using a Timer
.
2. Setting Up the Project
1. Create a New ASP.NET Core Project
- Start by creating a new ASP.NET Core project in Visual Studio or through the command line.
dotnet new webapi -n BackgroundTaskExample cd BackgroundTaskExample
2. Install Required Packages
- If you plan on using dependency injection within your background task, ensure all necessary packages are installed.
3. Add a New Hosted Service Class
3. Implementing a Background Task Using IHostedService
The IHostedService
interface defines two methods:
StartAsync
: Runs when the application starts.StopAsync
: Runs when the application is shutting down.
Here’s how to create a background task using these methods.
Step 1: Create the Hosted Service Class
Add a new class called TimedHostedService
in the project.
using System; using System.Threading; using System.Threading.Tasks; using Microsoft.Extensions.Hosting; using Microsoft.Extensions.Logging; public class TimedHostedService : IHostedService, IDisposable { private readonly ILogger<TimedHostedService> _logger; private Timer _timer; public TimedHostedService(ILogger<TimedHostedService> logger) { _logger = logger; } public Task StartAsync(CancellationToken cancellationToken) { _logger.LogInformation("Timed Background Service is starting."); // Schedule the background task to run every 5 minutes _timer = new Timer(DoWork, null, TimeSpan.Zero, TimeSpan.FromMinutes(5)); return Task.CompletedTask; } private void DoWork(object state) { _logger.LogInformation("Timed Background Service is working. {Time}", DateTimeOffset.Now); // Your background task code here, e.g., send an email, process data, etc. } public Task StopAsync(CancellationToken cancellationToken) { _logger.LogInformation("Timed Background Service is stopping."); _timer?.Change(Timeout.Infinite, 0); return Task.CompletedTask; } public void Dispose() { _timer?.Dispose(); } }
In this code:
- Timer: The
_timer
is used to specify the interval for running theDoWork
method. In this example, it runs every 5 minutes, but you can adjust the interval. - Logger:
_logger
is used to log information about the background service’s state, which is helpful for monitoring.
Step 2: Register the Hosted Service
Now that we have our background task class, we need to register it in the Startup.cs
or Program.cs
file to ensure it runs when the application starts.
If you’re using ASP.NET Core 6+, register the service in Program.cs
like this:
using Microsoft.Extensions.DependencyInjection; using Microsoft.Extensions.Hosting; var builder = WebApplication.CreateBuilder(args); // Register the background service builder.Services.AddHostedService<TimedHostedService>(); var app = builder.Build(); app.Run();
In older versions of ASP.NET Core, you can register the service in Startup.cs
within ConfigureServices
:
public void ConfigureServices(IServiceCollection services) { services.AddHostedService<TimedHostedService>(); }
4. Testing the Background Task
Run the application. You should see logging messages indicating that the background task is starting and working. The DoWork
method will log a message every 5 minutes.
5. Using Dependency Injection in Background Services
In real-world applications, background tasks often require dependencies such as database connections or external services. Dependency injection makes it easy to integrate these services.
To use dependency injection in your TimedHostedService
, update the constructor to accept other dependencies, and register them in Program.cs
or Startup.cs
:
public class TimedHostedService : IHostedService, IDisposable { private readonly ILogger<TimedHostedService> _logger; private readonly IServiceProvider _serviceProvider; private Timer _timer; public TimedHostedService(ILogger<TimedHostedService> logger, IServiceProvider serviceProvider) { _logger = logger; _serviceProvider = serviceProvider; } private void DoWork(object state) { using (var scope = _serviceProvider.CreateScope()) { var myDependency = scope.ServiceProvider.GetRequiredService<MyDependency>(); myDependency.ExecuteTask(); } _logger.LogInformation("Timed Background Service is working. {Time}", DateTimeOffset.Now); } }
In this example:
IServiceProvider
: Allows the service to request dependencies through a scoped service provider.CreateScope
: Ensures each background task instance has its own dependencies without conflicting with other services.
6. Advanced Scheduling with Quartz.NET
For tasks that require more complex scheduling, consider using Quartz.NET, an open-source scheduling library with advanced functionality.
To implement Quartz.NET:
1. Install the Quartz.NET package:
dotnet add package Quartz.Extensions.Hosting
2. Configure and register a job with Quartz in your application.
3. Set specific triggers and schedules, such as CRON expressions, to achieve finer control over task timing.
7. Final Thoughts
Background tasks are crucial for applications that require regular, unattended operations. ASP.NET Core’s IHostedService
provides a simple way to create scheduled tasks, while libraries like Quartz.NET enable more detailed scheduling configurations. ASPHostPortal, as a hosting provider, offers an optimized ASP.NET Core hosting environment, supporting applications that include background services like these, and provides robust security and performance monitoring tools.
Example of Practical Use Cases
- Database Cleanup: Use a background task to regularly clean up expired data.
- Email Notifications: Schedule periodic email notifications to users based on their activity or system events.
- Data Syncing: Sync data from external services at regular intervals.
Conclusion
By using ASP.NET Core’s IHostedService
, you can implement and manage background tasks efficiently. From email notifications to database maintenance, these tasks can be automated to improve user experience and application performance. With ASPHostPortal’s robust hosting solutions, you can confidently run and scale your ASP.NET Core applications with background services for added functionality and improved user engagement.
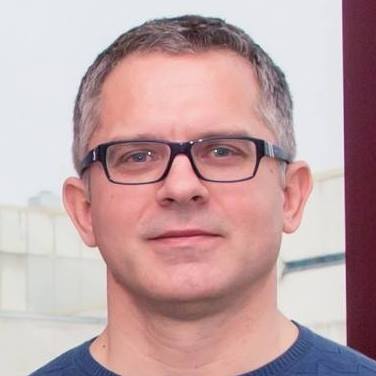
Javier is Content Specialist and also .NET developer. He writes helpful guides and articles, assist with other marketing and .NET community work