URLs are what define the web. The Url
property of ASP.NET Core Controllers, Razor Pages, and Razor views provides access to the UrlHelper
. The UrlHelper
can generate URLs for MVC actions, pages, and routes. Have a look at this C# expression:
Url.Action(action: "Home", controller: "Privacy")
The result of this expression will be a URL string pointing to the Privacy
action on the HomeController
: "/Home/Privacy"
.
This is only the absolute path, but it is a valid URL and should function properly. However, you may want to get the absolute URL, which includes the scheme, hostname or domain name, and port. For this purpose, the UrlHelper’s methods include overloads for specifying the protocol
, which is the scheme, and the host
, which is the hostname or domain name. Have a look at this C# expression:
Url.Action(action: "Home", controller: "Privacy", values: null, protocol: "https", host: "your-website")
By specifying the protocol and host, the result is now "https://your-website/Home/Privacy"
.
If you omit the host parameter but keep the protocol
parameter, the result will still be an absolute URL, because the host parameter will be the host used for the current HTTP request.
Url.Action(action: "Home", controller: "Privacy", values: null, protocol: "https")
The result will be something like "https://localhost:7184/Home/Privacy"
. This URL will be generated using the same host and port that were used to make the HTTP request.
In ASP.NET Core, the Scheme
property on the HttpRequest
can be used to generate a URL with the same scheme and host as the current request.
Url.Action(action: "Privacy", controller: "Home", values: null, protocol: Request.Scheme)
You could also pass Request.Host
as the host
parameter, but you don’t have to because it already uses the host
from the current HTTP request when you specify the protocol
but not the host parameter.
What if you don’t have access to the UrlHelper
or need to create a URL in a format that the UrlHelper
does not support?
You can also use the properties of the HttpRequest
to generate the absolute base URL for the current HTTP request. The following code creates an absolute URL for the absolute path “/your/custom/path”:
$"{Request.Scheme}://{Request.Host}{Request.PathBase}/your/custom/path"
The result of this expression looks like "https://localhost:7184/your/custom/path"
.
To get the full absolute URL, including the current absolute path, you can use the following C# expression:
$"{Request.Scheme}://{Request.Host}{Request.PathBase}{Request.Path}"
What if there’s no HTTP request?
If you’re not handling any HTTP requests in your code, such as when doing background processing with a BackgroundWorker
, you’ll need to get the scheme and host it another way. You could either store your desired scheme and host in your configuration, or combine them into a single property and store it there. Once configured, extract it from the configuration and use it to generate your absolute URL.
Conclusion
By default, UrlHelper
methods generate absolute paths, which differ from full absolute URLs in that they do not include the scheme, hostname, or port number. However, the UrlHelper
methods include overloads that allow you to generate the full absolute URL.
You can also manually construct the absolute URL using the HttpRequest
properties or by storing the desired scheme and hostname in configuration and then extracting it.
In most cases, using an absolute path will suffice and save some characters in your HTML, but absolute URLs are occasionally required, such as for technical SEO and social meta tags.
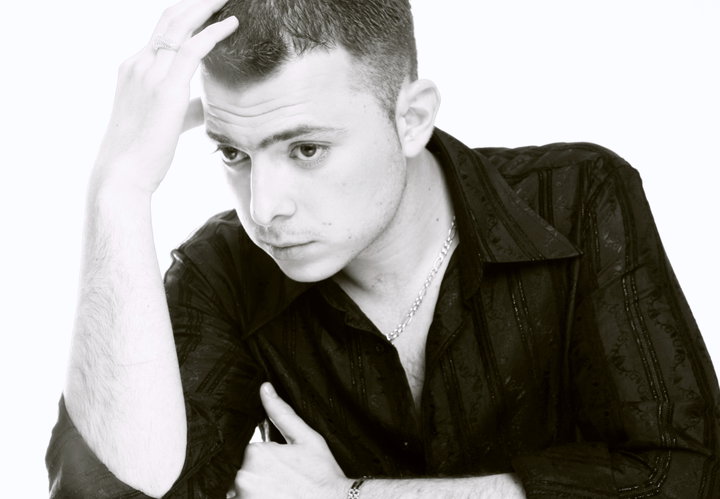
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.