In today’s world, QR codes are found in everything from product packaging and marketing campaigns to mobile payments and ticket validation. As a full-stack developer, you can create a plethora of engaging and convenient user experiences by integrating QR code functionality into your ASP.NET Core applications.
We’ll go deep into ASP.NET Core QR code implementation using C# and the potent QRCoder library in this extensive tutorial. This post will provide you the knowledge, code samples, and best practices you need to confidently build strong features powered by QR codes, regardless of your level of experience with them.
Understanding QR Codes
Prior to delving into the implementation specifics, let’s pause and examine the definition and operation of QR codes.
I abbreviate QR as “Quick Response.” Two-dimensional barcodes known as QR codes are capable of encoding a wide range of data, including text, URLs, contact details, and more. The Japanese company Denso Wave created them in 1994 so they could track the production of car parts.
QR Code Structure
Black and white squares arranged in a square grid on a white background make up a QR code. The particular pattern of these squares represents the encoded data. Because QR codes have integrated error correction, they can still be scanned and decoded even if a portion of the code is broken or obscured.
A QR code’s structure consists of the following components:
- Finder patterns: The QR code has three unique square patterns at its corners that are used to help with orientation and code location.
- Alignment patterns: smaller square patterns that aid in redistributing the code’s angle and size to the scanner.
- Timing patterns: Modules that alternate between black and white that specify the dimensions of the data matrix.
- Data and error correction keys: the actual encoded data as well as redundancy data for error fixing.
QR Code Versions And Capacities
Version 1 (21 x 21 modules) and version 40 (177 x 177 modules) are the various versions of QR codes. The data capacity varies among versions based on the error correction level and encoding mode selected.
Version | Modules | Capacity (Numeric) | Capacity (Alphanumeric) | Capacity (Binary) |
---|---|---|---|---|
1 | 21×21 | 41 | 25 | 17 |
2 | 25×25 | 77 | 47 | 32 |
3 | 29×29 | 127 | 77 | 53 |
… | … | … | … | … |
40 | 177×177 | 7,089 | 4,296 | 2,953 |
Error Correction Levels
Even if a section of the code is damaged, QR codes can still be decoded thanks to their four levels of error correction support. The tiers are:
- L (Low): Recovers up to 7% damage
- M (Medium): Recovers up to 15% damage
- Q (Quartile): Recovers up to 25% damage
- H (High): Recovers up to 30% damage
Higher error correction levels make the QR code more robust and increase data redundancy, but they also make the code larger.
Implementing QR Codes In ASP.NET Core
Now that we have a firm grasp on QR codes, let’s use the QRCoder library to implement them in an ASP.NET Core application.
Installing QRCoder
Installing the QRCoder NuGet package in your ASP.NET Core project will get you started. Use the Package Manager Console to accomplish this:
Install-Package QRCoder
Alternatively, you can use the dotnet CLI:
dotnet add package QRCoder
Generating QR Code Images
The QRCode
classes and QRCodeGenerator
are the central components of QRCoder. An introductory example of creating a QR code image from a text string is provided here:
using QRCoder;
using System.Drawing;
string data = "https://example.com";
QRCodeGenerator qrGenerator = new QRCodeGenerator();
QRCodeData qrCodeData = qrGenerator.CreateQrCode(data, QRCodeGenerator.ECCLevel.Q);
QRCode qrCode = new QRCode(qrCodeData);
Bitmap qrCodeImage = qrCode.GetGraphic(20);
This code does the following:
- Creates a new instance of
QRCodeGenerator
- Specifies the error correction level (
ECCLevel
) and uses theCreateQrCode
method to generate QR code data from theinput
data string. - Creates a
QRCode
object from theQRCodeData
- Using
GetGraphic
, renders the QR code as a bitmap image with the specified pixel size.
Before rendering the QRCode
object, you can set different properties to alter how the generated code looks. For instance:
qrCode.BackgroundColor = Color.White;
qrCode.ForegroundColor = Color.FromArgb(0, 123, 255);
qrCode.IconScale = 0.2f;
qrCode.IconImage = Image.FromFile("logo.png");
You can add a logo overlay, change the foreground and background colors, and modify the logo’s size and placement using these settings.
QR Code Generation in ASP.NET Core Controllers
You can use the previous example’s methodology to create QR codes in your ASP.NET Core controllers. This is an example of a controller action that uses a URL parameter to create a QR code image:
using Microsoft.AspNetCore.Mvc;
using QRCoder;
using System.Drawing;
using System.Drawing.Imaging;
[HttpGet]
public IActionResult GenerateQRCode(string url)
{
QRCodeGenerator qrGenerator = new QRCodeGenerator();
QRCodeData qrCodeData = qrGenerator.CreateQrCode(url, QRCodeGenerator.ECCLevel.Q);
QRCode qrCode = new QRCode(qrCodeData);
Bitmap qrCodeImage = qrCode.GetGraphic(20);
using (MemoryStream ms = new MemoryStream())
{
qrCodeImage.Save(ms, ImageFormat.Png);
return File(ms.ToArray(), "image/png");
}
}
Using the URL
parameter as input, this action method creates a QR code image, sets the error correction level to Q (25% recovery), and outputs the image as a PNG file.
In order to initiate this action, send a GET request to the relevant URL with the desired data passed as a query string parameter:
https://yourapp.com/qrcode/generate?url=https://example.com
Reading QR Codes From Images
It is also possible to read QR codes from bitmap images using QRCoder. This is an illustration of how to decode a QR code in an ASP.NET Core controller using an uploaded image file:
using Microsoft.AspNetCore.Http;
using Microsoft.AspNetCore.Mvc;
using QRCoder;
using System.Drawing;
[HttpPost]
public IActionResult ReadQRCode(IFormFile file)
{
using (var stream = file.OpenReadStream())
using (var bitmap = new Bitmap(stream))
{
QRCodeDetector qrDetector = new QRCodeDetector();
QRCodeData qrCodeData = qrDetector.Detect(bitmap);
if (qrCodeData != null)
{
string decodedData = qrCodeData.GetData();
return Content($"Decoded QR code data: {decodedData}");
}
else
{
return Content("No QR code found in the uploaded image.");
}
}
}
Using the QRCodeDetector
class from QRCoder, this action method takes an uploaded image file, tries to identify and decode a QR code, and then outputs the decoded text.
Performance Considerations
Performance is a critical consideration when creating or reading QR codes in ASP.NET Core applications, particularly when handling a large number of requests.
It can take a lot of resources to generate QR code images on the fly, especially if you’re using larger image sizes or higher error correction levels. Take into consideration the following tactics to maximize performance:
- Cache produced images of QR codes to prevent processing twice. The built-in caching features of ASP.NET Core, such as
IDistributedCache
andMemoryCache
, can be used to store and retrieve previously created images. - Reduce the response time of your web requests by handling QR code generation tasks asynchronously using background processing or queuing mechanisms.
- Depending on your unique use case, optimize the size and degree of error correction of your QR codes. Processing times and memory usage are increased with higher error correction settings and larger image sizes.
In comparison to generation, reading QR codes from images typically has less of an impact on performance. However, you might need to think about using techniques like parallel processing or assigning the task to background services if you’re processing a lot of images at once.
Advanced Integration Concepts And Use Cases
Numerous opportunities exist for improving user experiences and expediting procedures with QR codes. To get you thinking, here are some sophisticated use cases and integration concepts:
- Dynamic QR Codes: Create QR codes that contain dynamic data, like encrypted payloads, time-sensitive tokens, or user-specific information. This enables you to design safe and customized QR code experiences.
- Geographic Tagging: To enable location-based services or give users more context when scanning the codes, encode geographic coordinates or location identifiers in QR codes.
- Multi-Factor Authentication: Include QR codes in your workflow for multi-factor authentication. Create a QR code, for instance, that users can scan with a mobile app to finish the authentication process. The code could contain a challenge token or a one-time password.
- Contactless Payments: To enable contactless payments, incorporate QR code scanning functionality into your ASP.NET Core application. To start safe financial transactions, users can scan the QR codes that are displayed on their devices.
- Augmented Reality Integration: To create immersive experiences, combine augmented reality technologies with QR codes. The act of scanning a QR code can cause interactive elements or virtual content to overlay on to the physical world.
Error Handling And Logging
It’s essential to handle errors politely and log pertinent data for debugging and monitoring when adding QR code functionality to your ASP.NET Core application. Take into account these recommended practices:
- To capture and manage exceptions that might arise during the creation or scanning of QR codes, use try-catch blocks. Give users clear error messages and record comprehensive error data for later analysis.
- Use a reliable logging framework, such as Serilog or NLog, to implement logging. Record significant occurrences, like successfully generated QR codes, scans, and any problems that arose, together with pertinent metadata, such as timestamps and input parameters.
- To make searching through and analyzing log data easier, use structured logging techniques. Incorporate properties into your log messages, such as request IDs, user IDs, and QR code attributes.
- Keep an eye on application logs and configure alerts for serious mistakes or irregularities pertaining to the functionality of QR codes. By doing this, you can proactively find problems and fix them before they affect users.
Testing QR Code Functionality
You must write thorough unit tests to guarantee the accuracy and dependability of your QR code implementation. When testing ASP.NET Core’s QR code functionality, keep the following important factors in mind:
- Create QR codes using various input formats, then confirm that the resulting images have the expected features and contents.
- Use a range of legitimate and illegitimate images, with varying sizes, degrees of error correction, and damage scenarios, to test scanning QR codes. Make sure that valid QR codes are correctly decoded during the scanning process, and that corrupted or invalid codes are handled with grace.
- Check your QR code generation and scanning processes’ scalability and performance under various load scenarios. Measure response times and resource consumption by simulating high-traffic scenarios with load testing tools such as Apache JMeter or Loader.io.
- Verify the integration of your application’s QR code functionality with other features like user authentication, database storage, and third-party services. Verify that the error-handling and data flow mechanisms function as intended.
You can be sure that your QR code implementation is dependable and strong by taking care of these testing details.
Conclusion
We have covered all there is to know about QR codes in this extensive guide, including how to use the QRCoder library and C# to incorporate them into ASP.NET Core applications. In addition to providing thorough code samples for creating and reading QR codes in ASP.NET Core controllers, we have also covered the fundamentals of QR code structure, versions, and error correction levels.
In order to guarantee a strong and dependable QR code implementation, we’ve also covered performance considerations, advanced use cases, best practices for handling errors, and testing techniques.
Adding QR code functionality to your ASP.NET Core applications gives you, as a full-stack developer, a plethora of creative and practical user experience options. QR codes act as a link between the real and virtual worlds, whether you’re developing an augmented reality app, a contactless payment system, or a mobile ticketing system.
So feel free to experiment in your ASP.NET Core projects using QR codes. Use the knowledge in this guide and the capabilities of QRCoder to create incredible applications that meld the virtual and physical worlds together. Have fun with coding!
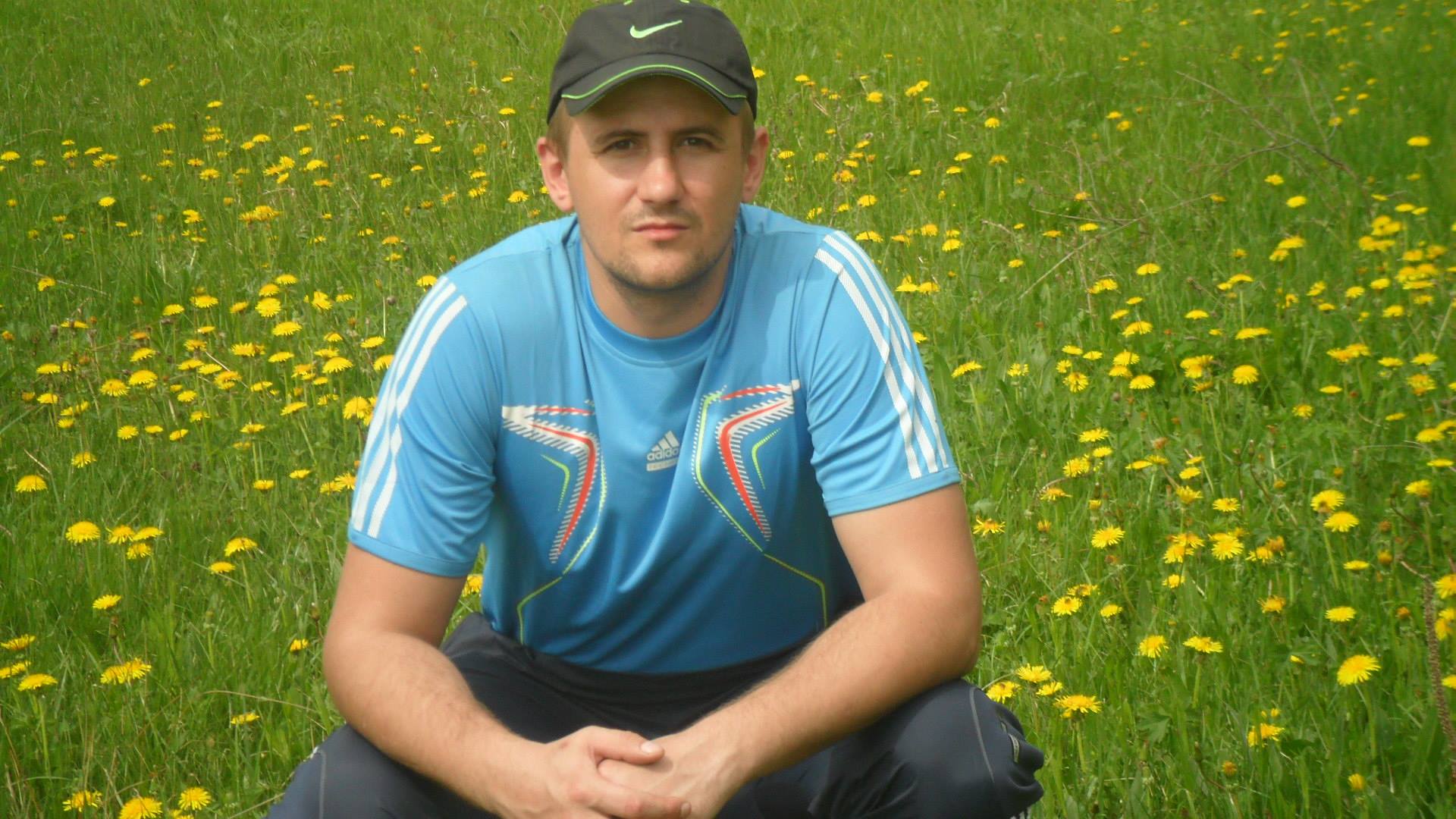
Yury Sobolev is Full Stack Software Developer by passion and profession working on Microsoft ASP.NET Core. Also he has hands-on experience on working with Angular, Backbone, React, ASP.NET Core Web API, Restful Web Services, WCF, SQL Server.