This post shows you how to handle Application Shutdown in ASP.NET Core by looking at the handling of flushing the application insights messages when asp.net core exits.
There are instances where you must do some logic after an ASP.NET Core web application terminates. Using logging as an example, you must flush the most recent message into the log (Application Insights). In that situation, adding an event to the Configure
method is necessary. I provide a brief illustration of how to achieve this in this post.
Register the Shutdown event
The Configure
in the Startup
class can have the following parameters:
IApplicationBuilder
IHostingEnvironment
ILoggerFactory
IApplicationLifetime
You can set up an event that is started when an application terminates using the IApplicationLifetime
interface:
public
class
Startup
{
public
void
Configure(IApplicationBuilder app, IApplicationLifetime applicationLifetime)
{
applicationLifetime.ApplicationStopping.Register(OnShutdown);
}
private
void
OnShutdown()
{
//this code is called when the application stops
}
}
The OnShutdown
method will now be called when the application closes due to new wiring.
Apply to Application Insights
Messages are buffered by default in Application Insights so they can be sent in bulk to the server. If you don’t flush your log, there’s a potential that you won’t see messages after your program shuts down. You need to flush buffer to fix this.
public
class
Startup
{
private
TelemetryClient _telemetryClient;
public
Startup(IHostingEnvironment env)
{
...
_telemetryClient =
new
TelemetryClient()
{
InstrumentationKey = Configuration[
"ApplicationInsights:InstrumentationKey"
],
};
...
}
public
void
Configure(IApplicationBuilder app, IApplicationLifetime applicationLifetime)
{
applicationLifetime.ApplicationStopping.Register(OnShutdown);
...
}
private
void
OnShutdown()
{
_telemetryClient.Flush();
//Wait while the data is flushed
System.Threading.Thread.Sleep(1000);
}
}
You won’t ever again forget to log a critical message with the help of these few lines of code.
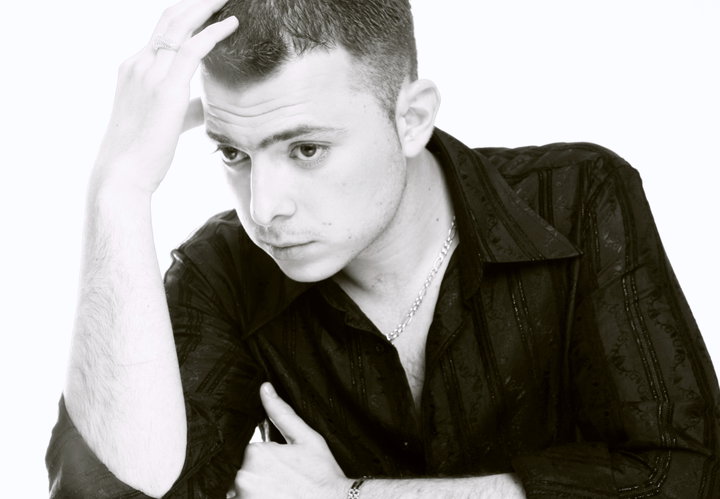
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.