It’s a fairly common issue – you need to get the MIME type for a given file extension. Maybe you need to set this properly for an email attachment, or it’s being set as part of uploading to Azure Blob Storage (note: weird stuff can happen if you don’t set the MIME type properly for Azure Blob Storage!).
In .NET Framework (4.5+) you could’ve used the MimeMapping.GetMimeMapping() method, but this isn’t part of .Net Core.
A Native .Net Core Solution
.Net Core introduced the class FileExtensionContentTypeProvider
, which is basically a wrapper around a mapping between file extension and content (MIME) type.
It can be used as follows:
using Microsoft.AspNetCore.StaticFiles; public string GetMimeTypeForFileExtension(string filePath) { const string DefaultContentType = "application/octet-stream"; var provider = new FileExtensionContentTypeProvider(); if (!provider.TryGetContentType(filePath, out string contentType)) { contentType = DefaultContentType; } return contentType; }
Adding Custom MIME Type Mappings
The in-built mappings may not support all the file extensions you need. Fortunately, you can add additional mappings like so:
var provider = new FileExtensionContentTypeProvider();
if (!provider.Mappings.TryGetValue(".csv", out var _)) { // Add new MIME mapping provider.Mappings.Add(".csv", "text/csv"); } else { // Replace existing MIME mapping as it already exists provider.Mappings[".csv"] = "text/csv"; }
Note that the ‘.’ needs to prefix any file extensions added.
Bonus: Let’s Make this an Extension Method
There are not many methods on FileExtensionContentTypeProvider
– it seems to jut be a thin wrapper around the dictionary of mappings. In the interests of creating a richer API, here’s an extension method to add or update a mapping inspired by the code above:
using Microsoft.AspNetCore.StaticFiles; ... public static void AddOrUpdateMapping( this FileExtensionContentTypeProvider provider, string fileExtension, string contentType) { if (!provider.Mappings.TryGetValue(fileExtension, out var _)) { provider.Mappings.Add(fileExtension,contentType); } else { provider.Mappings[fileExtension] = contentType; } }
Summary
When looking up MIME types in .Net Core, consider using the FileExtensionContentTypeProvider
class.
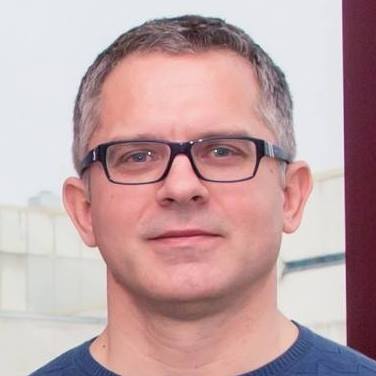
Javier is Content Specialist and also .NET developer. He writes helpful guides and articles, assist with other marketing and .NET community work