In previous article, we have explained how to fix error 502.5 process failure and also ANCM In-Process Start Failure ASP.NET Core. In this article tutorial, we will advise how to fix 404 error that you can find when deploying ASP.NET Core MVC project.
Assume that you have installed Visual Studio 2019 and you create ASP.NET Core MVC application here. When you execute the ASP.NET Core MVC project we’ve created in the preceding section, you will see the home page of the application together with the welcome message as shown below.
Now let’s try to browse a web page that doesn’t exist. To do this, type http://localhost:6440/welcome in the address bar of your browser while the application is in execution. When the ASP.NET Core MVC engine fails to locate the resource for the specified URL, a 404 error will be returned and you will be presented with the following error page. That’s not very elegant, is it?
Steps to Fix 404 Error Message
There are several ways in which you can improve on this generic error page. A simple solution is to check for the HTTP status code 404 in the response. If found, you can redirect the control to a page that exists. The following code snippet illustrates how you can write the necessary code in the Configure method of the Startup class to redirect to the home page if a 404 error has occurred.
app.Use(async (context, next) => { await next(); if (context.Response.StatusCode == 404) { context.Request.Path = "/Home"; await next(); } });
Now if you execute the application and try to browse the URL http://localhost:6440/welcome, you will be redirected to the home page of the application.
The complete code of the Configure method is given below for your reference.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { if (env.IsDevelopment()) { app.UseDeveloperExceptionPage(); } else { app.UseExceptionHandler("/Home/Error"); } app.Use(async (context, next) => { await next(); if (context.Response.StatusCode == 404) { context.Request.Path = "/Home"; await next(); } }); app.UseStaticFiles(); app.UseRouting(); app.UseAuthorization(); app.UseEndpoints(endpoints => { endpoints.MapControllerRoute( name: "default", pattern: "{controller=Home}/{action=Index}/{id?}"); }); }
Use UseStatusCodePages middleware in ASP.NET Core MVC
A second solution for handling 404 errors in ASP.NET Core is by using the built-in UseStatusCodePages middleware. The following code snippet shows how you can implement StatusCodePages in the Configure method of the Startup class.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env) { app.UseStatusCodePages(); //Other code }
Now when you execute the application and browse to the non-existent resource, the output will be similar to Figure 3.
Use UseStatusCodePagesWithReExecute middleware in ASP.NET Core MVC
You can take advantage of the UseStatusCodePagesWithReExecute middleware to handle non-success status codes in cases where the process of generating the response has not been started. Hence this middleware will not handle HTTP 404 status code errors — rather, when a 404 error occurs the control will be passed to another controller action to handle the error.
The following code snippet illustrates how you can use this middleware to redirect to another action method.
app.UseStatusCodePagesWithReExecute("/Home/HandleError/{0}");
Here’s what the action method would look like.
[Route("/Home/HandleError/{code:int}")] public IActionResult HandleError(int code) { ViewData["ErrorMessage"] = $"Error occurred. The ErrorCode is: {code}"; return View("~/Views/Shared/HandleError.cshtml"); }
I leave it to you to create the HandleError view to display the error message.
Finally, you might want to create views specifically for an error code. For example, you might create views such as Home/Error/500.cshtml or Home/Error/404.cshtml. You could then check the HTTP error code and redirect to the appropriate error page.
Yet another way of handling page not found errors is by using a custom view and setting the error code appropriately. When an error occurs in your application, you could redirect the user to the appropriate error page and display your custom error message describing the error.
Summary
Well, above tutorial is steps to debug 404 error that you might find when deploying ASP.NET Core MVC. If you find this tutorial interesting, you can share it. Thank you.
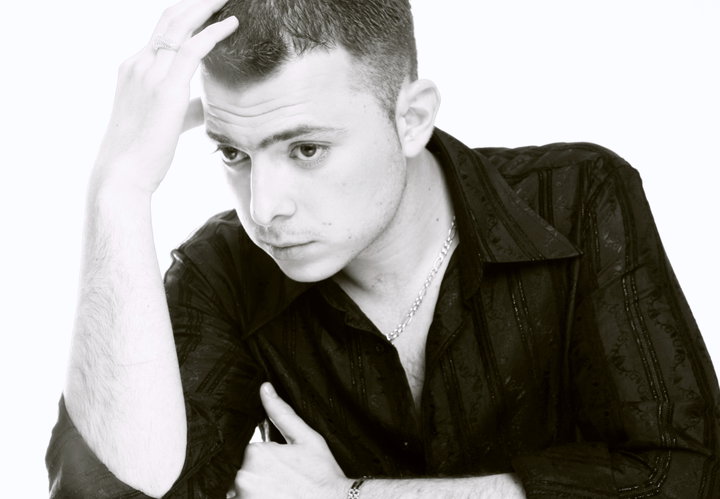
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.