The maximum request size is constrained by default when making bulky HttpPost requests, like uploading files or loading documents into the Document Viewer. For ASP.NET Core and ASP.NET applications to be ready to handle more requests, a few configurations must be made.
Additionally, the hosting server varies based on whether you use IIS or.NET Kestrel. The required configurations for the two hosting servers will be covered in this article.
ASP.NET Core
The maximum request size for applications built with ASP.NET Core is limited by default to 30MB. The ConfigureServices method in the Program.cs file sets this limit. You can set up the services that the application will use using the ConfigureServices method. The application’s request pipeline is configured in the Configure method, which is called before the ConfigureServices method.
Kestrel
You can set the Kestrel server options using the Configure<KestrelServerOptions>
; method, which will raise the maximum request size. The IServiceCollection
interface, which is the collection of services that the application will use, is where the Configure method is called.
An illustration of how to raise the maximum request size to about 8.59 GB can be found here:
builder.Services.Configure<KestrelServerOptions>(options => { options.Limits.MaxRequestBodySize = int.MaxValue; });
IIS
If you are hosting an ASP.NET Core application in IIS, you must configure the maximum request size in web.config. The web.config file is an XML file containing application configuration settings. The web.config file is located in the application’s root directory.
Here is an example of how to increase the maximum request size to the maximum value:
<system.webServer> <security> <requestFiltering> <requestLimits maxAllowedContentLength="4294967295" /> </requestFiltering> </security> </system.webServer>
ASP.NET
The maximum request size for ASP.NET applications is limited to 4 MB by default. This limit is set by your web.config file.
IIS Request Filtering
At the IIS level, the Request Filtering module is used to restrict the amount of data that IIS accepts. Increase the maxAllowedContentLength value, which determines the size of the POST buffer in bytes.
The default is 30000000 bytes (28.6 megabytes). The maximum value is 4294967295 bytes (4 gigabyte).
Here’s an example of increasing the maximum request size:
<system.webServer> <security> <requestFiltering> <requestLimits maxAllowedContentLength="4294967295" /> </requestFiltering> </security> </system.webServer>
HttpRuntime maxRequestLength
ASP.NET has its own option for limiting the size of uploads and requests. Use the maxRequestLength property of the httpRuntime element.
The default size is 4096 kilobytes (4 MB). The maximum value is approximately 82 terabytes (2,147,483,647 kilobytes). The following setting specifies a maximum size of 500 megabytes.
The request length can be defined as follows:
<system.web> <httpRuntime targetFramework="4.8" maxRequestLength="1048576" executionTimeout="120" /> </system.web>
These settings enable you to handle larger requests in your ASP.NET Core and ASP.NET applications.
Conclusion
When making large HttpPost requests, such as uploading files or loading documents into the Document Viewer, the maximum request size is set by default. Several configurations are required in ASP.NET Core and ASP.NET applications to prepare them to handle larger requests.
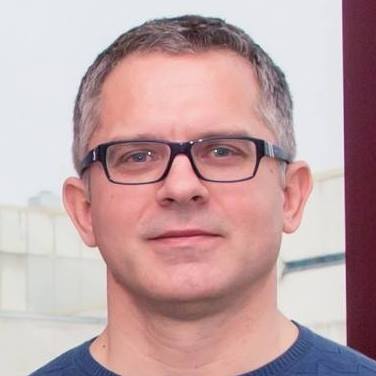
Javier is Content Specialist and also .NET developer. He writes helpful guides and articles, assist with other marketing and .NET community work