Code first migrations in Entity Framework has existed since Entity Framework 4.3. But migrations are still accessed from the package manager console and it’s not always easy to remember the commands and the parameters that go with them. This post will provide you with a quick reference for the most commonly used command names and parameters. This is not a complete reference guide. If you want to go deeper you can use get-help in package manager console.
get-help [command name] -detailed get-help [command name] -examples get-help [command name] -full example get-help enable-migrations -detailed
In code first migrations there are four commands
- enable-migrations
- add-migration
- update-database
- get-migrations
Enable-Migrations
The enable-migrations command not surprisingly enables migrations for your default project. The command by default creates a Configuration.cs file in a folder called Migrations.
enable-migrations
Parameters
-ContextTypeName
If your project contains multiple DbContext you can specify which one you want to enable migrations for.
-MigrationsDirectory
If you don’t want to have the default Migrations folder you can specify your own. This is especially handy if your project has multiple DbContext.
enable-migrations -ContextTypeName MyCoolContext -MigrationsDirectory MyCoolMigrations
-ProjectName
If your migrations should not be placed in your default project you can utilize the -projectname parameter
enable-migrations -ProjectName MyCoolMigrationProject
-EnableAutomaticMigrations
By default automatic migrations are turned off. If you need automatic migrations to be turned on you can use this flag. This command has an alias called -Auto.
enable-migrations -EnableAutomaticMigrations enable-migrations -Auto
-Force
You can only enable migrations once for each DbContext. If you need to overwrite your existing migration configuration you can use -Force. Be aware the all changes you did to your Configuration.cs file will be deleted.
enable-migrations -Force
Add-Migration
The add-migration command is one of the key commands in code first migrations. When you make changes to your domain model and need them added into your database you create a new migration. This is done with the Add-Migration command. In it’s simplest form you need only to provide a migration name.
Add-Migration MyCoolMigration Add-Migration -Name MyCoolMigration
The Add-Migration command scaffolds your changes into a cs file. This cs file is placed in the same folder as the configuration file for the DbContext you are targeting.
Parameters
-ProjectName
If your migrations should not be placed in your default project you can utilize the -projectname parameter
Add-Migration -Name MyCoolMigration -ProjectName MyCoolMigrationProject
-ConfigurationTypeName
If you have multiple DbContext in your project you will need to indicate which is going to have the database update. This can be done with -ConfigurationTypeName. The ConfigurationTypeName is the name of your Configuration class in your migration folder.
Add-Migration -Name MyCoolMigration -ConfigurationTypeName MyCoolNameSpace.Configuration
IgnoreChanges
If you have a baseline database schema which is compatible with your domain model you can decide not to start your migrations from scratch. For this you can use the -IgnoreChanges parameter. Be aware that all new developers will need to have the baseline database schema to get started since migrations will not create database from scratch.
Add-Migration -Name MyCoolMigration -IgnoreChanges
-Force
If you want to re-scaffold an existing migration you will have to use the -Force parameter. However you can only re-scaffold if the migration is not yet applied to database. Else you will need to revert back to the migration previous to the one you want to re-scaffold.
Add-Migration -Name MyCoolExistingMigration -Force
Update-database
The update-database command is exactly what it’s name says. If you call the command with no parameters you will get all migrations needed to update your database to latest migration.
Update-database
Parameters
-ProjectName
The name of the project containing your migrations. The parameter is only needed if the migrations are not in default project.
Update-database -ProjectName MyCoolMigrationProject
-ConfigurationTypeName
If you have multiple DbContext in your project you will need to indicate which is going to have the database update. This can be done with -ConfigurationTypeName. The ConfigurationTypeName is the name of your Configuration class inside your migration folder.
Update-Database -ConfigurationTypeName MyCoolNameSpace.Configuration
-TargetMigration
Used if you need to update to another migration than the latest. This is usually if you need to rollback your database to an older migration. Simply do an update-database and specify the target migration name.
Update-database -TargetMigration MyCoolMigration
-Script
If you need a script you can apply to another database you can specify the -script flag. This will create a SQL script instead of running the update on the current context. This is usually needed for updating other environments like test and production.
Update-database -Script
-SourceMigration
The SourceMigration parameter is only for generating scripts. It can only be used if the -script parameter is used as well. You use it for specifying which migration your target database is running.
Update-database -Script -SourceMigration MyCoolMigration
SourceMigration can be used with TargetMigration as well if you don’t want to update your database to latest migration.
Update-database -Script -SourceMigration MyCoolMigration -TargetMigration MyEvenCoolerMigration
Get-Migrations
The get-migrations command can be used for getting a list of all the migrations currently applied to the database.
Parameters
-ProjectName
If your migrations are not placed in your default project you can utilize the -projectname parameter
Get-Migrations -ProjectName MyCoolMigrationProject
-ConfigurationTypeName
If you have multiple DbContext in your project you will need to indicate which one you want the list of applied migrations for. The ConfigurationTypeName is the name of your Configuration class in your migration folder.
Get-Migrations -ConfigurationTypeName MyCoolNameSpace.Configuration
Additional parameters
Common parameters are supported by all the migration commands. I use the -verbose command quite a bit. This will show the SQL that’s applied to database.
Update-Database -verbose
Conclusion
I hope you enjoy this short tutorial!
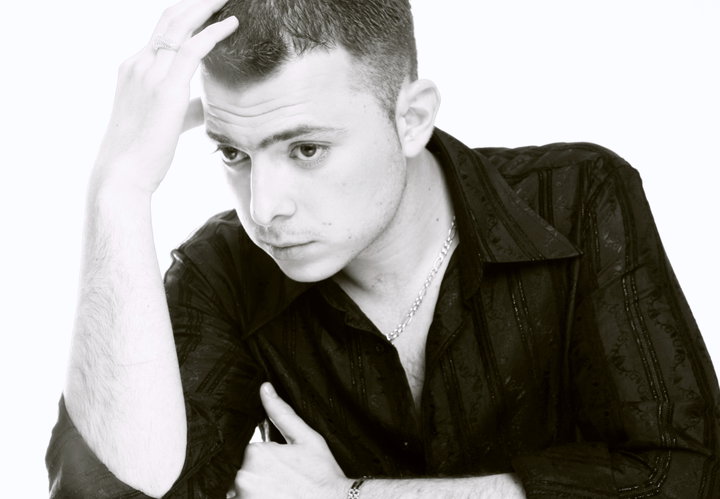
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.