We have checked that there are many users have problem in redirect their HTTP to HTTPS. In this article, we will explain how to redirect http to https in ASP.NET Core.
URL rewriting is the concept of changing the currently executing URL and pointing it at some other URL to continue processing the current request or redirecting to an external URL.
Migrating from HTTP to HTTPS in a IIS / ASP.NET environment
Google is urging more and more webmasters to move their sites to HTTPS for security reasons. Please make sure that you install your SSL on your website first. You can get FREE SSL from Let’s Encrypt. And if you host your site with us, we do support this FREE SSL and you can install directly via our control panel. Or we also offer paid SSL certificate start from $48/year.
Once you have purchased SSL, then we will generate CSR for you. You will get .cer file that we need to install it directly via IIS. But for Let’s Encrypt, you can just install it directly via our control panel. Assume that you have setup SSL via IIS and you want to redirect your http to https.
How to Redirect HTTP to HTTPS
1. Modify Your Web.Config file
You can use URL Rewrite to redirect http to https. Find your web.config file and add the following markup to the file;
<rewrite> <rules> <rule name="CanonicalHostNameRule"> <match url="(.*)" /> <conditions> <add input="{HTTP_HOST}" pattern="^www\.domain\.com$" negate="true" /> </conditions> <action type="Redirect" url="http://www.domain.com/{R:1}” /> </rule> </rules> </rewrite>
Make sure you replace “domain.com” above with your domain name. Now open up the Startup.cs file, add a using Microsoft.AspNetCore.Rewrite; directive at the top and enter the following code in Configure method:
app.UseRewriter(new RewriteOptions() .AddIISUrlRewrite(env.ContentRootFileProvider, "RedirectToWwwRule.xml") .AddRedirectToHttps() );
What this does is redirect the non-www version of the URL to the www version and redirect HTTP requests to HTTPS. You could also have removed the .AddRedirectToHttps() method and included it the URL Rewrite rule in the XML file and that would have worked too.
2. Regular Expressions and HTTPContext.Request.Path
This is the second method to perform redirection. Add the following code to the Startup.cs file:
app.UseRewriter(new RewriteOptions() .AddRedirectToWww() .AddRedirect("^hello$", "hai") .AddRedirectToHttps() );
With above code, it will redirect your http://www.domain.com/hello to https://www.domain.com/hai
Note that the AddRedirect() method evaluates the regular expression against the HttpContext.Request.Path, so if you need something more complicated, you’ll need to use the first or last method.
3. Adding a Rule using Class
This is last method that you can use to redirec http to https. Right click on your project, select Add -> New item… and add a class named RedirectToWwwRule.cs. Replace the entire class with this code:
using Microsoft.AspNetCore.Http; using Microsoft.AspNetCore.Http.Extensions; using Microsoft.AspNetCore.Rewrite; using System; namespace URLRewriteSample { public class RedirectToWwwRule : IRule { public virtual void ApplyRule(RewriteContext context) { var req = context.HttpContext.Request; if (req.Host.Host.Equals("domain.com", StringComparison.OrdinalIgnoreCase)) { context.Result = RuleResult.ContinueRules; return; } if (req.Host.Value.StartsWith("www.", StringComparison.OrdinalIgnoreCase)) { context.Result = RuleResult.ContinueRules; return; } var wwwHost = new HostString($"www.{req.Host.Value}"); var newUrl = UriHelper.BuildAbsolute(req.Scheme, wwwHost, req.PathBase, req.Path, req.QueryString); var response = context.HttpContext.Response; response.StatusCode = 301; response.Headers[Microsoft.Net.Http.Headers.HeaderNames.Location] = newUrl; context.Result = RuleResult.EndResponse; } } }
Then add the following code to your Startup.cs file:
var options = new RewriteOptions(); options.AddRedirectToHttps(); options.Rules.Add(new RedirectToWwwRule()); app.UseRewriter(options);
Summary
We hope above tutorial help you to redirect your http to https. URL Rewrite in ASP.NET Core is easy and simple. In next tutorial, we will discuss more about HTTPS and other interesting ASP.NET Core tutorial.
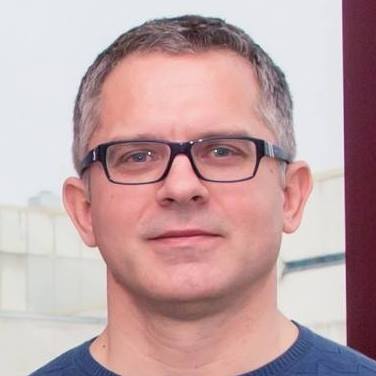
Javier is Content Specialist and also .NET developer. He writes helpful guides and articles, assist with other marketing and .NET community work