In this article, we are going to learn how to send email in ASP.NET Core. We are going to start with the simple project creation and creating a basic email configuration. We will create service only for email according to S in SOLID, what define “single responsibility”. What is more I will show you how to use dependency Injection in ASP.NET Core.
At the beginning we have to create new project.
As I said earlier we will use ASP.NET Core Web Api, so we have to choose this:
Before we start crete logic we have to add to our appsetting.json some settings for our email client. In appsetings.json file:
In this file we will define our email, password, host and port. It is neccessary because our application have to connect to our email and send message though him.
{ "Logging": { "IncludeScopes": false, "Debug": { "LogLevel": { "Default": "Warning" } }, "Console": { "LogLevel": { "Default": "Warning" } } }, "Email": { "Email": "[email protected]", "Password": "password", "Host": "host", "Port": "port" } }
For example for gmail Port is 587 and host: “smtp.gmail.com”
If We add email setting to appsetting.json file, we have to create new folder with 1 interface and 1 class. I named this folder: Services and in this folder is interface called IEmailSender and class EmailSender. I created interface because I want to show you Dependency Injection.
My project structure in this moment:
In IEmailSender we have to just define one method:
using System.Threading.Tasks; namespace Email.Services { public interface IEmailService { Task SendEmail(string email, string message); } }
This method will take 3 strings:email, subject and message for our email.
In EmailSender class we have to implement our Interface and his method.
using System.Net; using System.Net.Mail; using System.Threading.Tasks; using Microsoft.Extensions.Configuration; namespace Email.Services { public class EmailService : IEmailService { private readonly IConfiguration _configuration; public EmailService(IConfiguration configuration) { _configuration = configuration; } public async Task SendEmail(string email, string subject, string message) { using (var client = new SmtpClient()) { var credential = new NetworkCredential { UserName = _configuration["Email:Email"], Password = _configuration["Email:Password"] }; client.Credentials = credential; client.Host = _configuration["Email:Host"]; client.Port = int.Parse(_configuration["Email:Port"]); client.EnableSsl = true; using (var emailMessage = new MailMessage()) { emailMessage.To.Add(new MailAddress(email)); emailMessage.From = new MailAddress(_configuration["Email:Email"]); emailMessage.Subject = subject; emailMessage.Body = message; client.Send(emailMessage); } } await Task.CompletedTask; } } }
At the begining we have have constructor with take IConfiguration Interface as a parameter, and a private variable IConfiguration _configuration. It means that when we create new instance of EmailService we have initialize IConfiguration object, thanks him we can read our email settings.
Then we create method SendEmail with has 3 parameters, like in previous Interface. In this method we will use SmtpClient, it is library from ASP.NET Core for sending email. In this using we define our credentials for email – it is taken from our settings file. When we change our password or email we dont have to change code, just change our settings and everything will be working. Then we setting parameters to our client, and at the end we will send our email asynchronously.
Ok, we have all necessary methods, no we can create main method in our controller responsible for sending message.
Now we have to prepare our Controller for send email message, everything what we have to do is inject our IEmailSender in constructor.
private readonly IEmailService _emailService; public AccountController(IEmailService emailService) { _emailService = emailService; }
in Controller we will create new POST method:
[HttpPost] [Route("account/send-email")] public async Task<IActionResult> SendEmailAsync([FromUri]string email, string subject, string message) { await _emailService.SendEmail(email, subject, message); return Ok(); }
Last thing what left is telling our application that when we use IEmailService we want to use EmailService methods. Everything what we have to do is change our startup.cs file:
in this way:
public void ConfigureServices(IServiceCollection services) { services.AddMvc(); services.AddTransient<IEmailService, EmailService>(); }
Conclusion
We did a great job here. Now we have a fully functional Web API service that we can use in our projects to send email messages.
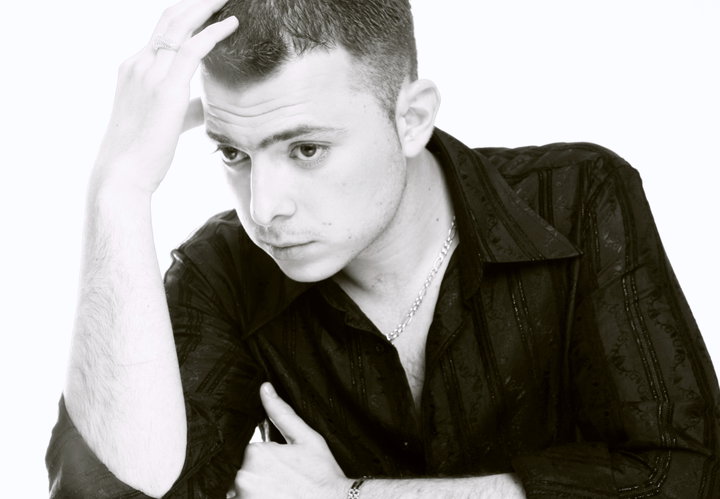
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.