Previously, we have written tutorial about how to redirect http to https in ASP.NET Core. In this article we will discuss futher again how to use URL Rewriting Middleware in ASP.NET Core. We assume that you have built your ASP.NET Core project. When you create your new ASP.NET project, please ensure that you un-tick boxes “Enable Docker Support” and “Configure for HTTPS” as we won’t be using those features.
Installing URL Rewrite Middleware via NuGet
When you finished create your ASP.NET Core project, you should have Microsoft.AspNetCore.Rewrite package installed in your project. Please make sure you install NuGet Package Manager inside the Visual Studio 2019 IDE or you can install this package via .NET CLI
dotnet add package Microsoft.AspNetCore.Rewrite
URL redirection and URL Rewriting
URL redirection and URL rewriting are different. URL redirection asks the client to access the resource using another URL. The client would then update the URL as appropriate and access the resource using the new URL.
In other hand, URL rewriting is a server-side operation. In URL rewriting, the rewritten URL is not sent back to the client; the client is never aware that the resource resides in a different location than the one the client requested.
Setup URL Redirection in ASP.NET Core
You can configure URL rewriting and URL redirection in the Startup class. In the Configure method of the Startup.cs file, add the following code to redirect HTTP requests to HTTPS.
app.UseRewriter(new RewriteOptions() .AddRedirectToHttps());
Setup URL Rewrite in ASP.NET Core
The following is an example of URL Rewriting
var rewrite = new RewriteOptions() .AddRewrite("api/values", "api/default", true); app.UseRewriter(rewrite);
When you execute the application, you’ll observe that the HttpGet method of the DefaultController is called but the web browser shows this URL:
http://localhost:52137/api/values
You can also specify the rules using regex expressions as shown in the code snippet given below.
var rewrite = new RewriteOptions() .AddRewrite(@"^Home/User?Code=(\d+)", "Home/$1", true); app.UseRewriter(rewrite);
How to Create a custom URL Rewriting Rule in ASP.NET Core
To work with complex rules, you can create a custom rule as well. To do this, you need to create a class that extends the IRule interface and implements the ApplyRule method as shown in the code snippet given below.
public class CustomRule : Microsoft.AspNetCore.Rewrite.IRule { public void ApplyRule(RewriteContext context) { throw new NotImplementedException(); } }
The following code snippet illustrates how the ApplyRule method can be used. Note that the ApplyRule method shown here is for illustrative purposes only. You can change it as per your own requirements.
public class CustomRule : Microsoft.AspNetCore.Rewrite.IRule { public void ApplyRule(RewriteContext context) { var request = context.HttpContext.Request; var host = request.Host; if (host.Host.Contains("localhost", StringComparison.OrdinalIgnoreCase)) { if(host.Port == 80) { context.Result = RuleResult.ContinueRules; return; } } var response = context.HttpContext.Response; response.StatusCode = (int)HttpStatusCode. BadRequest; context.Result = RuleResult.EndResponse; } }
How to Add a Custom Role in ASP.NET Core
You can add your custom rule to the rules collection in the Configure method as shown in the code snippet given below.
public void Configure(IApplicationBuilder app, IHostingEnvironment env) { var options = new RewriteOptions(); options.Rules.Add(new CustomRule()) app.UseRewriter(options); }
URL rewriting decouples the address from the underlying resource. You can take advantage of URL rewriting to provide user-friendly URLs that are simpler and easier to remember, as well as more meaningful to search engines.
Summary
We hope above tutorial help can help you differentiate between URL Redirection and URL Rewrite. Stay tune in next tutorial!
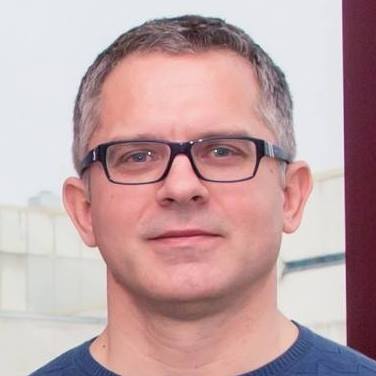
Javier is Content Specialist and also .NET developer. He writes helpful guides and articles, assist with other marketing and .NET community work