Because of its simpler, lighter architecture and cross-platform support, ASP.NET Core is growing in popularity for creating online apps. These high-traffic ASP.NET Core applications operate in a load-balanced multi-server environment. In reality, it’s normal to see web farms with 10 to 20 servers, and some are considerably bigger.
Your application tier is particularly scalable if you have a multi-server load-balanced deployment since you can add more servers as your transaction traffic rises. This makes it possible for your ASP.NET Core application to easily handle high transaction loads. Your ASP.NET Core application is slowed down by a persistent performance barrier.
Your database and data storage are the bottleneck for ASP.NET Core performance because they are unable to manage high loads like your application tier can. You can increase the number of servers in the web farm’s application-tier, but not its database-tier. The two methods of data storage that slow down ASP.NET Core apps’ performance are listed below.
- Database Server (SQL Server)
- ASP.NET Core Sessions
Distributed Cache Help to Boost Your ASP.NET Core Performance
Your best option is to employ a distributed cache like NCache to eliminate these data storage performance issues. The in-memory distributed cache for.NET called NCache is substantially quicker than the database. Because NCache allows you to create a cluster of cache servers and add more servers to the cluster as your transaction demands rise, it is linearly scalable unlike your database.
By caching application data, NCache enables you to cut down on costly database trips by approximately 80%. As a result, the database will handle both reads and writes significantly more quickly and won’t be a performance bottleneck any longer.
App Data Caching Using IDistributedCache in ASP.NET Core
Before ASP.NET Core, the previous version of ASP.NET offered a standalone ASP.NET Cache that didn’t work in situations with several servers. Now that third-party distributed caches may be smoothly plugged in, ASP.NET Core has provided the IDistributedCache interface as a pretty simple distributed caching standard API that you can program against.
An illustration of the IDistributedCache interface in use is shown here:
IDistributedCache _cache; ... private byte[] LoadCustomer(string custId) { string key = "Customers:CustomerID:" + custId; // is the customer in the cache? byte[] customer = _cache.Get(key); if (customer == null) { // the cache doesn't have it. so load from DB customer = LoadFromDB(key); // And, cache it for next time _cache.Set(key, customer); } return customer; }
You can integrate NCache’s provider for IDistributedCache
into your ASP.NET Core apps. You can avoid changing any NCache-specific code in this way.
Here is an illustration of the IDistributedCache
interface; each of these methods also has an Async overload, for your reference.
How to Setup NCache as IDistributedCache Provider
Here is how you setup NCache as your IDistributedCache
provider in your ASP.NET Core Startup
class.
public class Startup
{
...
public void ConfigureServices (IServiceCollection services)
{
...
services.AddNCacheDistributedCache();
...
}
...
}
Reasons to Use NCache API Over IDistributedCache
Use the IDistributedCache
interface if your caching requirements are simple and you want the flexibility of switching the distributed caching vendor effortlessly. You can easily switch your caching vendor thanks to it. But contrast that with the price of lacking many sophisticated caching options.
Utilizing NCache API straight from your ASP.NET Core application is an additional choice. The ASP.NET Cache API from the past is pretty similar to NCache API. It has a ton of free features that let you make the most of an enterprise-grade distributed cache.
Keep in mind that your application will benefit from improved performance and scalability the more data you can cache. Additionally, you’re frequently limited to caching read-only or simple data without the deeper caching options. Continue reading to learn more about the many NCache caching features that you are missing if you use the IDistributedCache
provider.
Storing ASP.NET Core Sessions in Distributed Cache
The earlier version of ASP.NET offered an ASP.NET Session State Provider architecture that permitted third-party session storage providers to plug-in before ASP.NET Core. Similar plug-and-play functionality is offered by ASP.NET Core sessions for connecting external storage providers. The two methods for using NCache as ASP.NET Core session storage are as follows:
Utilize NCache for ASP.NET Core Sessions via IDistributedCache
You don’t need to do anything else once you configure NCache as an IDistributedCache provider for ASP.NET Core; NCache will automatically take over as the session storage option. But be aware that this implementation has fewer features than the previous (ASP.NET Session State before ASP.NET Core) version.
The default ASP.NET Core Sessions implementation lacks a number of features, including the following:
- Session locking: Session locking is not available in ASP.NET Core. Even the earlier ASP.NET Session State had offered this.
byte[]
array for custom objects: Before you can store any custom objects in the session, ASP.NET Core compels you to convert them all into byte arrays. Custom objects are supported by even older ASP.NET Session State.
Use NCache as ASP.NET Core Sessions Provider
NCache has created its own ASP.NET Core Sessions provider to circumvent the IDistributedCache
provider’s default implementation of ASP.NET Core Sessions. Compared to the default implementation, this one has many more features.
You set it up in your Startup
class as follows.
public class Startup { ... public void Configure(IApplicationBuilder app, IHostingEnvironment env) { ... app.UseNCacheSession(); ... } ... }
You can specify ASP.NET Core Session configurations for the above in appsettings.json
file as following:
{ ... "NCacheSessions": { ... "CacheName": "demoCache", "EnableLogs": "True", "RequestTimeout": "90", "EnableDetailLogs": "False", "ExceptionsEnabled": "True", "WriteExceptionsToEventLog": "False" } ... }
Final Verdict
As IDistributedCache
providers, Microsoft offers two choices. One is Redis, and the other is SQL Server. Both alternatives are inferior to NCache. NCache is significantly faster and more scalable than SQL Server. The following are additional reasons why NCache is superior to Redis:
- Native .NET: Because NCache is entirely native to.NET, it integrates flawlessly into your.NET application stack. Redis, on the other hand, is not a native.NET cache and has a Linux origin.
- Faster than Redis: Due to NCache’s Client Cache feature, which significantly improves performance, NCache is actually faster than Redis.
- More Features: Redis lacks a number of crucial distributed cache features that NCache does.
Are you looking for an affordable and reliable Asp.net core hosting solution?
Get your ASP.NET hosting as low as $1.00/month with ASPHostPortal. Our fully featured hosting already includes
- Easy setup
- 24/7/365 technical support
- Top level speed and security
- Super cache server performance to increase your website speed
- Top 9 data centers across the world that you can choose.
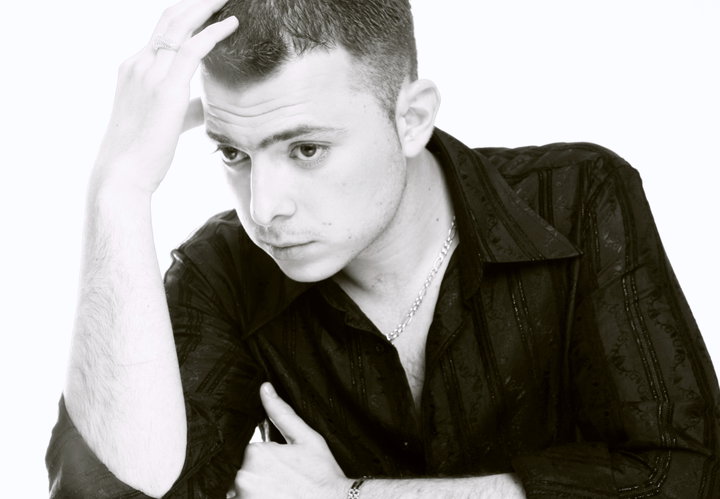
Andriy Kravets is writer and experience .NET developer and like .NET for regular development. He likes to build cross-platform libraries/software with .NET.