What is Angular and ASP.NET Core?
Angular Overview
ASP.NET Core Overview
Comparison Focus
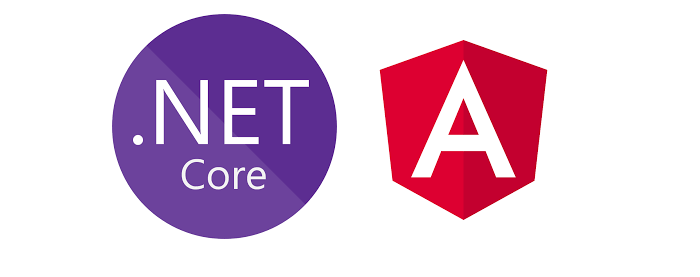
Direct Comparison: Angular vs. Blazor with ASP.NET Core
Language and Development
-
Angular: Uses Typescript/JavaScript, appealing to developers familiar with JavaScript ecosystems. It has a steep learning curve due to its comprehensive features, supported by tools like Angular CLI.
-
Blazor: Uses C#, ideal for .NET developers, offering a familiar environment with Visual Studio integration. It simplifies development for teams already using .NET, with options for WebAssembly (client-side) or server-side rendering.
Performance
-
Angular: Compiled to JavaScript, runs client-side, suitable for typical web apps but may lag in computationally intensive tasks.
-
Blazor: WebAssembly mode compiles C# to run in the browser, potentially offering better performance for heavy computations, though initial load times might be longer due to WebAssembly download.
Community and Ecosystem
-
Angular: Boasts a large, mature community with numerous third-party libraries, perfect for projects needing extensive JavaScript tools.
-
Blazor: Growing community within the .NET ecosystem, smaller than Angular’s, but benefits from Microsoft’s support and .NET resources.
Integration with Back-end
-
Angular: Integrates with ASP.NET Core via RESTful APIs, requiring separate front-end and back-end management.
-
Blazor: Tight integration with ASP.NET Core, allowing shared code and easier communication, enhancing full-stack .NET development.
When to Choose
-
Choose Angular if your team prefers JavaScript, needs extensive libraries, or works on projects with complex front-end requirements.
-
Choose Blazor if your team is .NET-focused, prefers C#, or wants a unified language for front-end and back-end, especially for performance-critical applications.
Surprising Detail: Language Shift
Survey Note: Detailed Comparison of Angular and ASP.NET Core (Including Blazor)
Introduction
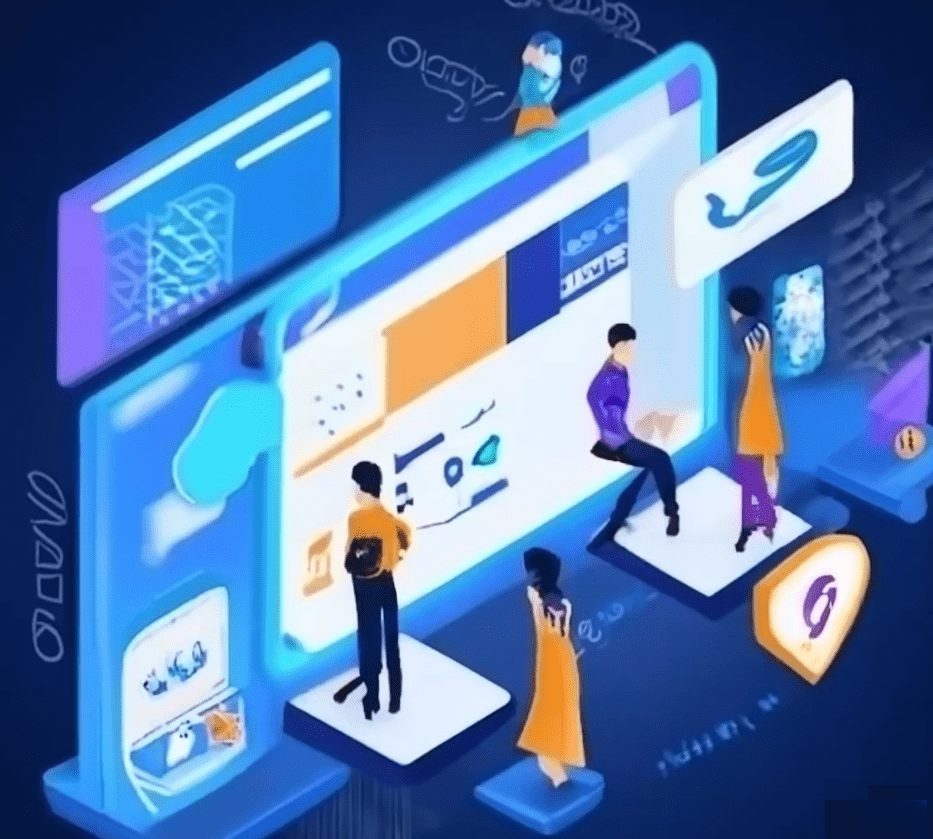
Background and Definitions
Angular, launched by Google, is a component-based framework for building scalable web applications. It uses Typescript, enhancing JavaScript with static typing, and includes features like dependency injection, routing, and two-way data binding. Its ecosystem is vast, supported by a large community, making it ideal for complex, large-scale projects. For more details, visit the Angular Official Website.
ASP.NET Core is an open-source, cross-platform framework for building web applications, focusing on server-side development using C#. It supports multiple paradigms, including RESTful APIs, and includes front-end frameworks like Razor Pages for server-side rendering and Blazor for client-side development. Blazor, introduced as part of ASP.NET Core, allows building interactive web UIs with C# and Razor syntax, leveraging WebAssembly for client-side execution. Explore further at the Blazor Official Documentation.
Key Differences
Aspect
|
Angular
|
Blazor
|
---|---|---|
Language
|
Typescript/JavaScript, compiled to JavaScript
|
C#, with Razor syntax, compiled to WebAssembly or server-side
|
Performance
|
Client-side, JavaScript execution, suitable for typical web apps, may lag in heavy computations
|
WebAssembly mode offers potential performance gains for intensive tasks, initial load may be slower due to WebAssembly
|
Development Experience
|
Steep learning curve, supported by Angular CLI, rich tooling for JavaScript developers
|
Familiar for .NET developers, integrates with Visual Studio, simpler for C# teams, but less mature ecosystem
|
Community and Ecosystem
|
Large, mature community, extensive third-party libraries, ideal for JavaScript-heavy projects
|
Growing .NET community, smaller than Angular’s, benefits from Microsoft’s support, fewer third-party options
|
Integration with Back-end
|
Integrates via RESTful APIs with ASP.NET Core, requires separate front-end/back-end management
|
Tight integration with ASP.NET Core, allows shared code, enhances full-stack .NET development
|
Language and Paradigm
Performance Considerations
Development Experience
Angular’s development experience is robust, with a steep learning curve due to its comprehensive features, supported by Angular CLI for project management, testing, and deployment. Blazor, integrated with .NET tools like Visual Studio, offers a familiar environment for .NET developers, simplifying development for teams already invested in the ecosystem. However, its ecosystem is less mature, potentially limiting third-party library options.
Community and Ecosystem
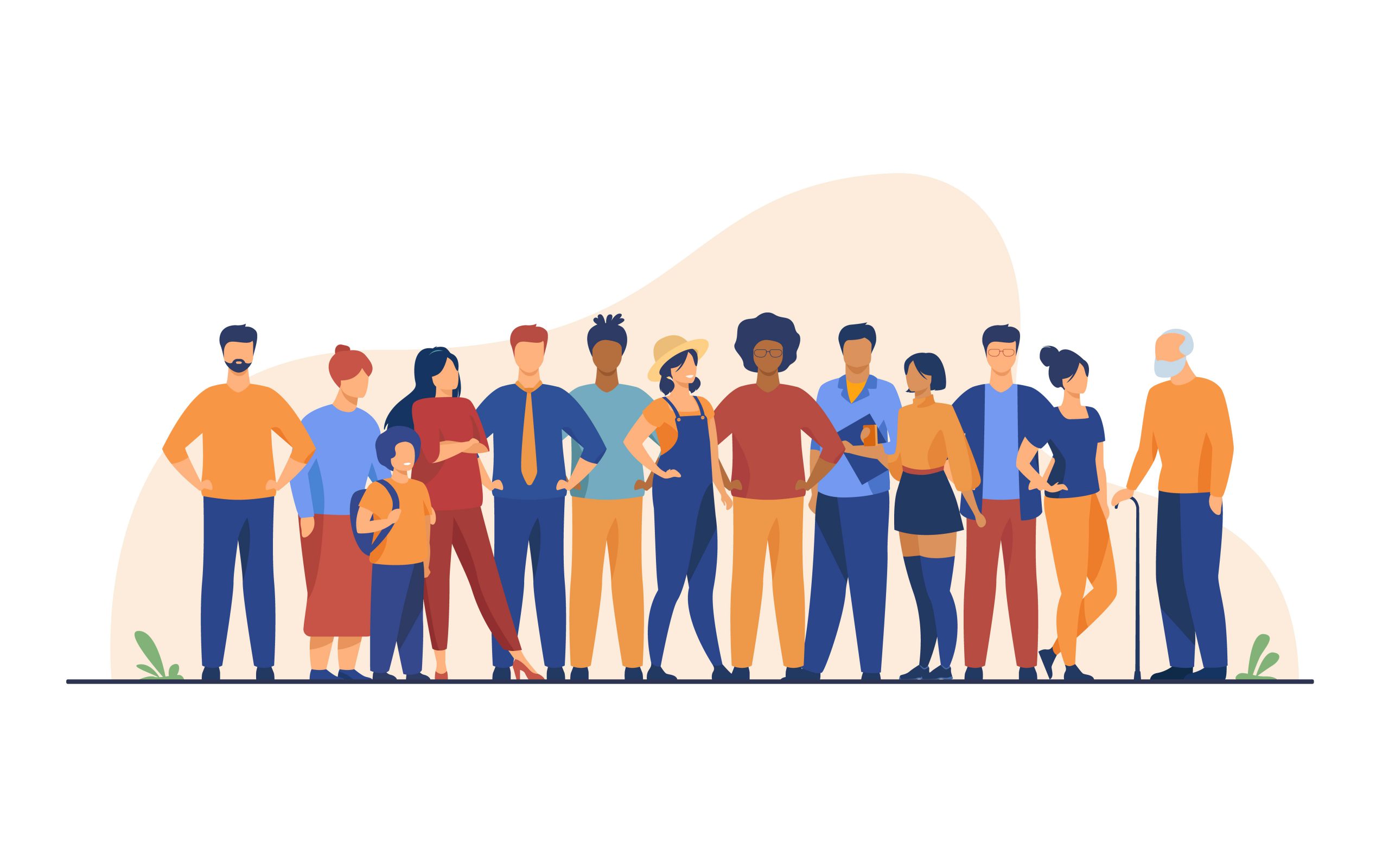
Integration with Back-end
Both frameworks integrate with ASP.NET Core back-end via APIs, but Blazor’s tight integration allows shared code and easier communication, enhancing full-stack development. Angular, requiring separate front-end and back-end management, may introduce complexity in data passing and model management, as noted in discussions on Stack Overflow (ASP.NET Core 2.0 Razor vs Angular/React/etc).
When to Choose Each
-
Choose Angular if:
-
Your team is comfortable with JavaScript/Typescript and prefers a mature, extensive ecosystem.
-
The project requires extensive use of JavaScript libraries or has complex front-end needs.
-
You need a large community for support and resources, ideal for large-scale, JavaScript-heavy projects.
-
-
Choose Blazor if:
-
Your team is .NET-focused, preferring C# for both front-end and back-end development.
-
You want a unified language and ecosystem, simplifying full-stack development.
-
Performance-critical applications benefit from WebAssembly, or you seek tighter integration with ASP.NET Core.
-
Examples: Implementing a Todo List Application
First, set up an Angular project using the Angular CLI:
ng new todo-app
// src/app/todo-list/todo-list.component.ts
import { Component } from '@angular/core';
import { Todo } from '../models/todo';
import { TodoService } from '../services/todo.service';
@Component({
selector: 'app-todo-list',
templateUrl: './todo-list.component.html',
styleUrls: ['./todo-list.component.css']
})
export class TodoListComponent {
todos: Todo[];
constructor(private todoService: TodoService) {
this.todos = this.todoService.getTodos();
}
}
Set up a Blazor project in ASP.NET Core:
dotnet new blazor -o todo-app
// todo-app/Pages/TodoList.razor
@page "/todo-list"
@using todo_app.Data
<h1>Todo List</h1>
<ul>
@foreach (var todo in todos)
{
<li>@todo.Name</li>
}
</ul>
@code {
private List<Todo> todos = new List<Todo>();
protected override void OnInitialized()
{
// Fetch todos from service or database
// For simplicity, hardcoding here
todos.Add(new Todo { Name = "Task 1" });
todos.Add(new Todo { Name = "Task 2" });
}
}
Conclusion
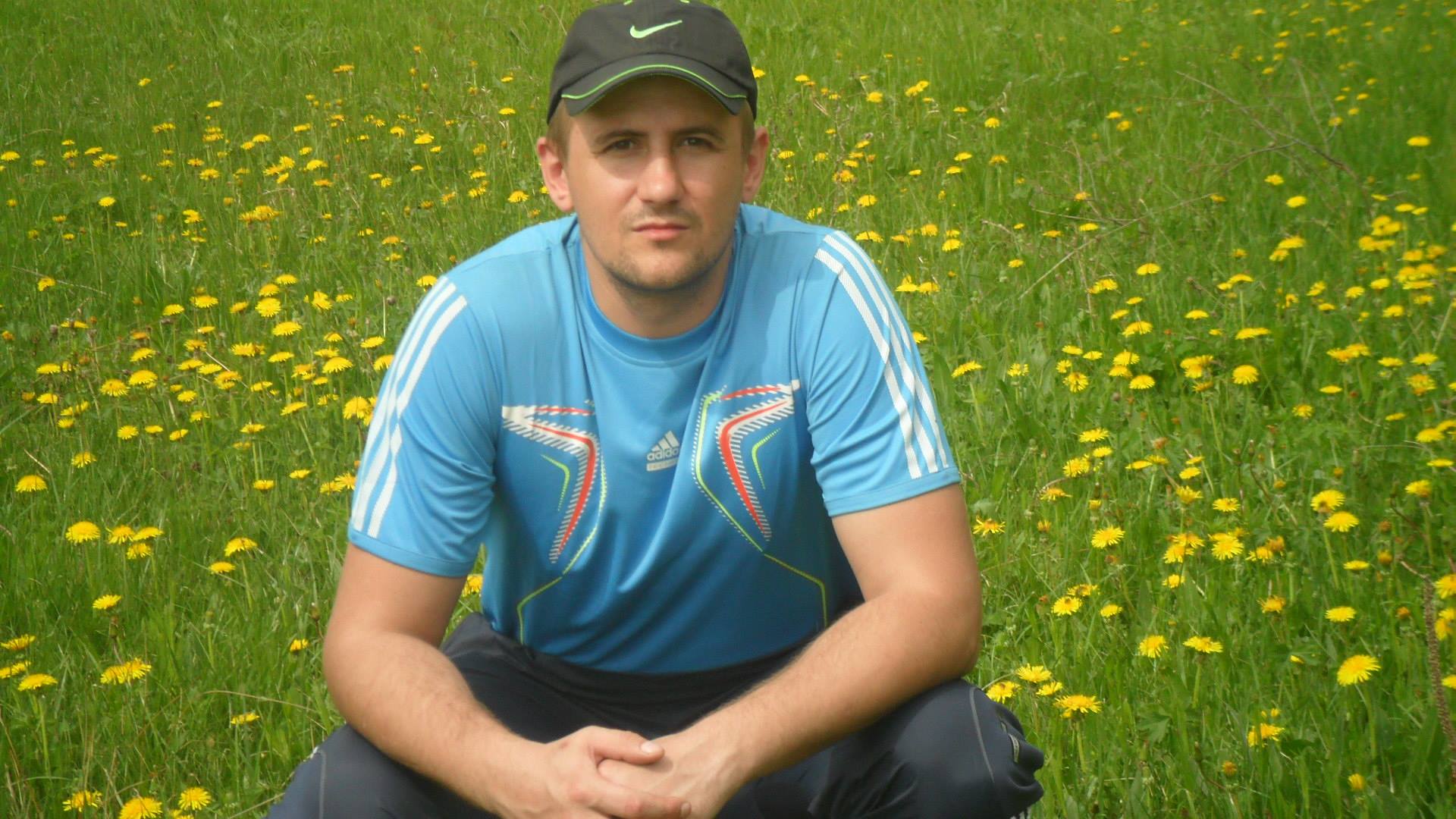
Yury Sobolev is Full Stack Software Developer by passion and profession working on Microsoft ASP.NET Core. Also he has hands-on experience on working with Angular, Backbone, React, ASP.NET Core Web API, Restful Web Services, WCF, SQL Server.