Runtime errors during production builds in Angular applications can be frustrating, especially when the development environment works seamlessly. These errors often stem from misconfigurations, code inconsistencies, or unoptimized settings specific to the production environment. This article will delve into common causes of runtime errors in production builds, practical solutions, and best practices to ensure smooth deployment.
Understanding Runtime Errors in Angular Production Builds
Runtime errors occur when code that runs perfectly in development encounters problems in production. Angular’s production build (ng build --prod
) optimizes and minifies code to improve performance, which may expose underlying issues. Common symptoms include broken functionality, missing assets, or crashes upon application load.
Common Causes of Runtime Errors in Production
- Missing Production-Specific Configuration
Development configurations often differ from production. If production-specific configurations are incomplete or incorrect, errors can occur. - Unoptimized Lazy Loading
Incorrect route configurations for lazy-loaded modules may cause navigation issues or module failures. - Improper Usage of Third-Party Libraries
Some libraries may behave differently in production due to code minification or tree-shaking. - Missing or Misconfigured Polyfills
Older browsers may fail to run modern JavaScript features if polyfills are not included. - Uncaught Errors During Optimization
Angular’s optimization process can expose coding issues like missing types, unused imports, or bad logic. - Environment Variable Mismanagement
Failing to properly configure environment-specific settings may cause mismatches between development and production. - Uncaught Errors in Templates
Errors in Angular templates, such as null references or bindings, might only surface in production.
Step-by-Step Guide to Fix Runtime Errors
Step 1: Verify Build Command
Always use the correct command for production builds:
ng build --prod
Step 2: Enable Debugging for Production Builds
To better understand runtime errors, include source maps in your production build:
ng build --prod --source-map
Step 3: Check Environment Files
Ensure your environment files (environment.ts
, environment.prod.ts
) are configured correctly. For instance, verify API endpoints or feature toggles:
export const environment = { production: true, apiEndpoint: 'https://api.example.com' };
Step 4: Optimize Lazy Loading
Incorrect lazy loading can lead to runtime errors. Verify module paths in AppRoutingModule
:
const routes: Routes = [ { path: 'feature', loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule) } ];
Step 5: Update Third-Party Libraries
Outdated libraries can cause compatibility issues. Update dependencies in package.json
:
npm outdated npm install <package-name>@latest
Step 6: Include Necessary Polyfills
Ensure the polyfills.ts
file includes compatibility for older browsers:
import 'zone.js/dist/zone'; // Required for Angular import 'core-js/features/array/includes'; // Example polyfill
Step 7: Handle Null References
Errors like Cannot read property of null
often arise in templates. Use safe navigation operators (?.
) to prevent such errors:
<p>{{ user?.name }}</p>
Step 8: Check for Global Variables
Avoid relying on global variables or browser-specific behaviors. Use Angular’s dependency injection system to manage shared resources.
Step 9: Run a Pre-Deployment Test
Run the production build locally to catch errors before deployment:
ng serve --prod
Ensure all templates, decorators, and Angular modules adhere to AOT requirements:
- Avoid dynamic templates.
- Declare all components and modules explicitly.
- Ensure all types are correctly defined.
Best Practices for Avoiding Runtime Errors
1. Use TypeScript Strict Mode
Enable strict mode in tsconfig.json
to catch potential errors during development:
"strict": true, "noImplicitAny": true
2. Conduct Code Audits
Regularly review your codebase for unused imports, undefined variables, or potential pitfalls.
3. Monitor Browser Compatibility
Use tools like Can I Use to ensure your application supports target browsers.
4. Implement Comprehensive Testing
Use unit tests and end-to-end tests to validate functionality. Tools like Jasmine and Protractor can help automate testing.
5. Leverage Linting Tools
Linting tools like ESLint can identify code issues early. Configure Angular’s linting rules in angular.json
.
6. Use CI/CD Pipelines
Integrate a CI/CD pipeline to automate builds, tests, and deployments. This reduces the chance of human error.
Examples of Fixes
Fixing a Null Reference Error
Before:
<p>{{ user.name }}</p>
After:
<p>{{ user?.name }}</p>
Fixing Lazy Loading Issue
Before:
const routes: Routes = [ { path: 'feature', loadChildren: './feature/feature.module#FeatureModule' } ];
After:
const routes: Routes = [ { path: 'feature', loadChildren: () => import('./feature/feature.module').then(m => m.FeatureModule) } ];
Conclusion
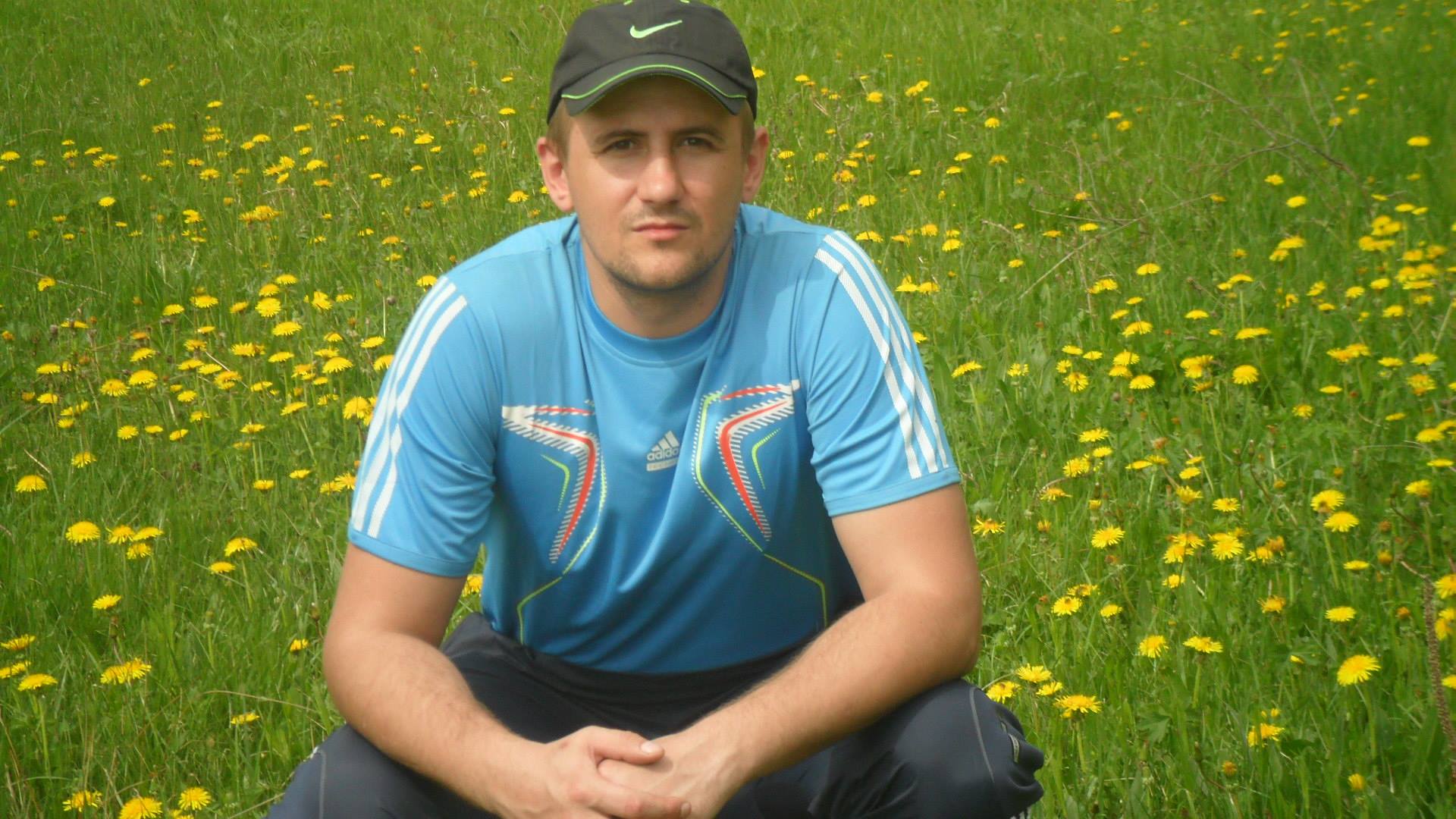
Yury Sobolev is Full Stack Software Developer by passion and profession working on Microsoft ASP.NET Core. Also he has hands-on experience on working with Angular, Backbone, React, ASP.NET Core Web API, Restful Web Services, WCF, SQL Server.